Angular FormGroup addControl() and removeControl()
March 27, 2020
This page will walk through Angular addControl
and removeControl
methods of FormGroup
example. The FormGroup
can add, update or remove a form control at runtime. The form control can be FormGroup
, FormControl
and FormArray
. Find the methods of FormGroup
used to add, update or remove controls.
addControl(): Adds a control and updates the value and validity of the control.
removeControl(): Removes a control and updates the value and validity of the control.
setControl(): Replace an existing control and updates the value and validity of the control.
contains(): Checks if enabled control exists for the given name.
registerControl(): Registers a control but does not update the value or validity of the control.
Here on this page we will provide complete example to add and remove
FormGroup
, FormControl
and FormArray
controls to an existing FormGroup
.
Contents
1. Technologies Used
Find the technologies being used in our example.1. Angular 9.0.2
2. Node.js 12.5.0
3. NPM 6.9.0
2. addControl()
TheaddControl
adds a control to the FormGroup
at runtime. Find the method declaration.
addControl(name: string, control: AbstractControl): void
FormGroup
.
control: This is the any implementation class of
AbstractControl
such as FormGroup
, FormControl
and FormArray
.
Find the sample code.
this.personForm.addControl('nationality', this.formBuilder.control('', [Validators.required]));
3. removeControl()
TheremoveControl
removes the control from the FormGroup
at runtime by given control name. Find the method declaration.
removeControl(name: string): void
FormGroup
. Find the code to use it.
this.personForm.removeControl('nationality');
4. setControl()
ThesetControl
replaces existing control. Find the method declaration.
setControl(name: string, control: AbstractControl): void
control: We can pass here instance of
FormGroup
, FormControl
and FormArray
.
Find the sample example.
this.personForm.setControl('nationality', this.formBuilder.control('', [Validators.required]));
5. contains()
Thecontains
checks if there is any enabled control for the specified control name in that FormGroup
. Find the method declaration.
contains(controlName: string): Boolean
FormGroup
, FormControl
and FormArray
.
The return value is Boolean.
Find the sample example.
const isAvailable = this.personForm.contains('nationality');
6. Add and Remove FormControl
Suppose we have aFormGroup
as following.
personForm: FormGroup = this.formBuilder.group({ });
FormControl
to existing FormGroup
at runtime.
this.isIndian.valueChanges.subscribe(res => { if (res === 'false') { this.personForm.addControl('nationality', this.formBuilder.control('', [Validators.required])); } else { this.personForm.removeControl('nationality'); } });
<ng-container *ngIf="isIndian.value === 'false'"> <input formControlName="nationality"> <label *ngIf="personForm.get('nationality').errors?.required" class="error"> Enter nationality. </label> </ng-container>
7. Add and Remove FormGroup
Suppose we have an existing instance ofFormGroup
as personForm. Now we will create one more FormGroup
as companyFG.
companyFG: FormGroup = this.formBuilder.group({ compName: ['', Validators.required], compLocation: ['', Validators.required] });
this.isEmp.valueChanges.subscribe(val => { if (val === 'true' && !this.personForm.contains('company')) { this.personForm.addControl('company', this.companyFG); } else { this.personForm.removeControl('company'); } });
<ng-container *ngIf="isEmp.value === 'true'"> <div formGroupName="company"> Company: <input formControlName="compName"> <label *ngIf="companyFG.get('compName').errors?.required" class="error"> Enter company name. </label> Location: <input formControlName="compLocation"> <label *ngIf="companyFG.get('compLocation').errors?.required" class="error"> Enter company location. </label> </div> </ng-container>
8. Add and Remove FormArray
Suppose we have an existing instance ofFormGroup
as personForm. We will add and remove FormArray
to the personForm conditionally at runtime.
this.favBookEntry.valueChanges.subscribe(fbEntry => { if (fbEntry && !this.personForm.contains('favBooks')) { this.personForm.addControl('favBooks', this.formBuilder.array([new FormControl('', [Validators.required])])); } else { this.personForm.removeControl('favBooks'); } });
<ng-container *ngIf="favBookEntry.value === true"> <div formArrayName="favBooks"> <div *ngFor="let b of favBooks.controls; index as i"> <input [formControlName]="i"> <label *ngIf="favBooks.controls[i].errors?.required" class="error"> Enter the name. </label> <button type="button" (click)="deleteBook(i)">Delete</button> </div> </div> <button type="button" (click)="addMoreBooks()">Add More Books</button> </ng-container>
9. Complete Example
person.component.tsimport { Component, OnInit } from '@angular/core'; import { HttpResponse, HttpEventType } from '@angular/common/http'; import { FormControl, FormArray, FormBuilder, Validators, FormGroup } from '@angular/forms'; import { PersonService } from './person.service'; @Component({ selector: 'app-person', templateUrl: 'person.component.html' }) export class PersonComponent implements OnInit { constructor(private formBuilder: FormBuilder, private personService: PersonService) { } personForm: FormGroup = this.formBuilder.group({ pname: ['', Validators.required], isIndian: ['', Validators.required], isEmp: ['', Validators.required], favBookEntry: '' }); companyFG: FormGroup = this.formBuilder.group({ compName: ['', Validators.required], compLocation: ['', Validators.required], profile: ['', Validators.required] }); ngOnInit() { this.handleNationality(); this.handleEmploymentStatus(); this.handleFavoriteBooks(); } get pname() { return this.personForm.get('pname') as FormControl; } get isIndian() { return this.personForm.get('isIndian') as FormControl; } get isEmp() { return this.personForm.get('isEmp') as FormControl; } get favBookEntry() { return this.personForm.get('favBookEntry') as FormControl; } get favBooks() { return this.personForm.get('favBooks') as FormArray; } handleNationality() { this.isIndian.valueChanges.subscribe(res => { if (res === 'false') { this.personForm.addControl('nationality', this.formBuilder.control('', [Validators.required])); } else { this.personForm.removeControl('nationality'); } }); } handleEmploymentStatus() { this.isEmp.valueChanges.subscribe(val => { if (val === 'true' && !this.personForm.contains('company')) { this.personForm.addControl('company', this.companyFG); } else { this.personForm.removeControl('company'); } }); } handleFavoriteBooks() { this.favBookEntry.valueChanges.subscribe(fbEntry => { if (fbEntry && !this.personForm.contains('favBooks')) { this.personForm.addControl('favBooks', this.formBuilder.array([new FormControl('', [Validators.required])])); } else { this.personForm.removeControl('favBooks'); } }); } deleteBook(index: number) { this.favBooks.removeAt(index); } addMoreBooks() { this.favBooks.push(new FormControl('', [Validators.required])); } onFormSubmit() { if (this.personForm.valid) { this.personService.savePerson(this.personForm.value); } } }
<h3>Person Form</h3> <form [formGroup]="personForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td> Name: <input formControlName="pname"> <label *ngIf="pname.errors?.required" class="error"> Name required. </label> </td> </tr> <tr> <td> <input type="radio" value="true" formControlName="isIndian"> Indian <input type="radio" value="false" formControlName="isIndian"> Others <br/> <label *ngIf="isIndian.errors?.required" class="error"> Select nationality. </label> </td> </tr> <ng-container *ngIf="isIndian.value === 'false'"> <tr> <td> Nationality: <input formControlName="nationality"> <label *ngIf="personForm.get('nationality').errors?.required" class="error"> Enter nationality. </label> </td> </tr> </ng-container> <tr> <td> <input type="radio" value="true" formControlName="isEmp"> Employee <input type="radio" value="false" formControlName="isEmp"> Student <br/> <label *ngIf="isEmp.errors?.required" class="error"> Select any. </label> </td> </tr> <ng-container *ngIf="isEmp.value === 'true'"> <tr> <td> <div formGroupName="company"> <table> <tr> <td>Company:</td> <td> <input formControlName="compName"> <label *ngIf="companyFG.get('compName').errors?.required" class="error"> Enter company name. </label> </td> </tr> <tr> <td>Location:</td> <td> <input formControlName="compLocation"> <label *ngIf="companyFG.get('compLocation').errors?.required" class="error"> Enter company location. </label> </td> </tr> <tr> <td>Profile:</td> <td> <input formControlName="profile"> <label *ngIf="companyFG.get('profile').errors?.required" class="error"> Enter profile. </label> </td> </tr> </table> </div> </td> </tr> </ng-container> <tr> <td> <input type="checkbox" formControlName="favBookEntry"> Enter favorite books? </td> </tr> <ng-container *ngIf="favBookEntry.value === true"> <tr> <td> <div formArrayName="favBooks"> <div *ngFor="let b of favBooks.controls; index as i"> <input [formControlName]="i"> <label *ngIf="favBooks.controls[i].errors?.required" class="error"> Enter the name. </label> <button type="button" (click)="deleteBook(i)">Delete</button> </div> </div> <button type="button" (click)="addMoreBooks()">Add More Books</button> </td> </tr> </ng-container> </table> <button>Submit</button> </form>
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class PersonService { savePerson(personData) { console.log(personData); } }
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <app-person></app-person> ` }) export class AppComponent { }
table { border-collapse: collapse; } table, th, td { border: 1px solid black; padding: 10px; } .error { color: red; } .success { color: green; }
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { ReactiveFormsModule } from '@angular/forms'; import { AppComponent } from './app.component'; import { PersonComponent } from './person.component'; @NgModule({ imports: [ BrowserModule, ReactiveFormsModule ], declarations: [ AppComponent, PersonComponent ], providers: [ ], bootstrap: [ AppComponent ] }) export class AppModule { }
10. Run Application
To run the application, find the steps.1. Download source code using download link given below on this page.
2. Use downloaded src in your Angular CLI application. To install Angular CLI, find the link.
3. Run ng serve using command prompt.
4. Access the URL http://localhost:4200
Find the print screen of the output.
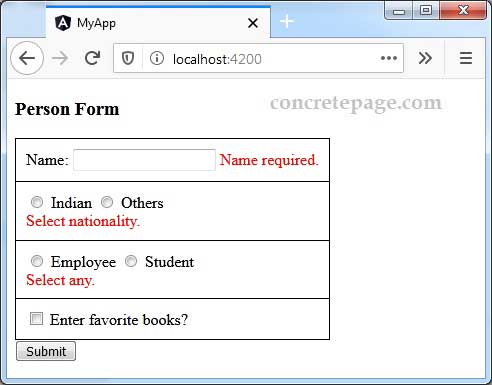
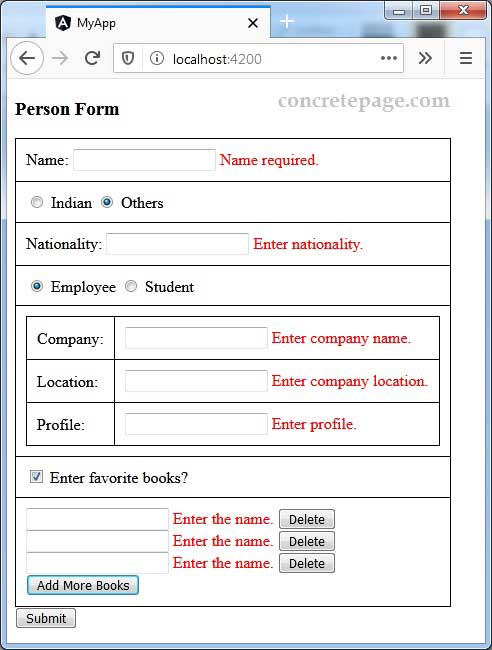
11. References
Angular FormGroupAngular valueChanges and statusChanges