Angular Material Button Toggle Default Selected
November 02, 2022
On this page we will learn to set Material button toggle selected as default in our Angular Material application.
1. Material button toggle is created using
<mat-button-toggle>
component. The <mat-button-toggle>
creates on/off toggles with the appearance of a button.
2. The
<mat-button-toggle>
are used within <mat-button-toggle-group>
component.
3. Button toggles are configured to behave as either radio-buttons or checkboxes.
4. To use Material button toggle, we need to import following module in our application module.
import { MatButtonToggleModule } from '@angular/material/button-toggle';
ngModel
, FormControl
and checked
property. The checked
is the boolean property of <mat-button-toggle>
component.
Contents
Technologies Used
Find the technologies being used in our example.1. Angular 13.1.0
2. Angular Material 13.3.9
3. Node.js 12.20.0
4. NPM 8.2.0
Using ngModel
The default selection of button toggle can be achieved usingngModel
binding.
TypeScript code:
travelBy = "car";
<mat-button-toggle-group [(ngModel)]="travelBy"> <mat-button-toggle value="bus">Bus</mat-button-toggle> <mat-button-toggle value="car">Car</mat-button-toggle> <mat-button-toggle value="train">Train</mat-button-toggle> </mat-button-toggle-group> <p><br/>Chosen value is <b>{{travelBy}}</b></p>
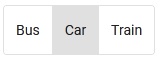
Using FormControl
Here we will create button toggle with default selected usingFormControl
.
Type Script code:
season = new FormControl('mansoon');
<mat-button-toggle-group [formControl]="season"> <mat-button-toggle value="summer">Summer</mat-button-toggle> <mat-button-toggle value="winter">Winter</mat-button-toggle> <mat-button-toggle value="mansoon">Mansoon</mat-button-toggle> </mat-button-toggle-group> <p><br/>Chosen value is <b>{{season.value}}</b></p>
Using checked
The <mat-button-toggle>
has checked
property that accepts boolean value to decide whether the button is checked.
<mat-button-toggle-group name="favoriteColor" #colorGroup="matButtonToggleGroup"> <mat-button-toggle value="red">Red</mat-button-toggle> <mat-button-toggle value="green" checked>Green</mat-button-toggle> <mat-button-toggle value="blue">Blue</mat-button-toggle> </mat-button-toggle-group> <p><br/>Chosen value is <b>{{colorGroup.value}}</b></p>
Multiple Default Selection
The multiple selection in button toggle is enabled usingmultiple
attribute. To set more than one button selected as default, we can use checked
property of <mat-button-toggle>
component.
<mat-button-toggle-group multiple #cityGroup="matButtonToggleGroup"> <mat-button-toggle value="vns">Varanasi</mat-button-toggle> <mat-button-toggle value="ayo" checked>Ayodhya</mat-button-toggle> <mat-button-toggle value="del">Delhi</mat-button-toggle> <mat-button-toggle value="pra" checked>Prayagraj</mat-button-toggle> </mat-button-toggle-group> <p><br/>Chosen value is <b>{{cityGroup.value}}</b></p>
Complete Example with Reactive Form
Here we are creating reactive form with button toggles that are selected as default.app.component.html
<h2>Reactive Form</h2> <form [formGroup]="personForm" (ngSubmit)="onFormSubmit()"> <div> <mat-form-field> <input matInput formControlName="name" placeholder="Name"> </mat-form-field> </div> <div> <br/>Travel by: <mat-button-toggle-group formControlName="travelBy"> <mat-button-toggle value="bus">Bus</mat-button-toggle> <mat-button-toggle value="car">Car</mat-button-toggle> <mat-button-toggle value="train">Train</mat-button-toggle> </mat-button-toggle-group> </div> <div> <br/>Season: <mat-button-toggle-group formControlName="season"> <mat-button-toggle value="summer">Summer</mat-button-toggle> <mat-button-toggle value="winter">Winter</mat-button-toggle> <mat-button-toggle value="mansoon">Mansoon</mat-button-toggle> </mat-button-toggle-group> </div> <div> <br/>Company: <mat-button-toggle-group formControlName="company"> <mat-button-toggle value="reliance">Reliance</mat-button-toggle> <mat-button-toggle value="tata">Tata</mat-button-toggle> <mat-button-toggle value="infosys">Infosys</mat-button-toggle> <mat-button-toggle value="hcl">HCL</mat-button-toggle> </mat-button-toggle-group> </div> <div> <br/> <button mat-raised-button>Submit</button> </div> </form>
import { Component } from '@angular/core'; import { FormControl, FormBuilder } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html' }) export class AppComponent { constructor(private formBuilder: FormBuilder) { } personForm = this.formBuilder.group({ name: '', travelBy: 'car', season: 'mansoon', company: 'tata' }); onFormSubmit() { console.log(this.personForm.value); } }
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { FormsModule } from '@angular/forms'; import { ReactiveFormsModule } from '@angular/forms'; import { MatFormFieldModule } from '@angular/material/form-field'; import { MatButtonModule } from '@angular/material/button'; import { MatButtonToggleModule } from '@angular/material/button-toggle'; import { MatInputModule } from '@angular/material/input'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, BrowserAnimationsModule, FormsModule, ReactiveFormsModule, MatFormFieldModule, MatButtonModule, MatButtonToggleModule, MatInputModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Run Application
To run the application, find the steps.1. Install Angular CLI using link.
2. Install Angular Material using link.
3. Download source code using download link given below on this page.
4. Use downloaded src in your Angular CLI application.
5. Run ng serve using command prompt.
6. Access the URL http://localhost:4200
Find the print screen of the output.
