Angular Material Select : Getting and Setting value
October 03, 2019
Angular Material Select is created using <mat-select>
which is a form control for selecting a value from a set of options. To add elements to Select option, we need to use <mat-option>
element and to bind value with <mat-option>
, use value
property of it. To set and get a value for <mat-select>
, use value
, ngModel
, formControl
and formControlName
property. To work with Angular Material Select, we need to import MatSelectModule
in application module file. Angular Material Select can also be created using native <select>
element by adding matNativeControl
attribute with it. To create Angular Material select either by <mat-select>
or native <select>
, we need to keep them inside <mat-form-field>
element. For multiple selection, we need to use multiple
attribute with <mat-select>
and native <select>
. Now let us discuss to work with Angular Material Select in detail step-by-step.
Contents
- Technologies Used
- Import
MatSelectModule
- Create Select using
<mat-select>
- Create Select using native
<select>
withmatNativeControl
- Getting and setting with
value
- Getting and setting with
NgModel
- Populate Select Option Dynamically
- Angular Material Select and Multiple Select Example using Reactive Form
- Run Application
- Reference
- Download Source Code
Technologies Used
Find the technologies being used in our example.1. Angular 8.2.8
2. Angular Material 8.2.1
3. Node.js 12.5.0
4. NPM 6.9.0
Import MatSelectModule
To work with Angular Material Select, we need to import MatSelectModule
in application module file.
import { MatSelectModule } from '@angular/material'; @NgModule({ imports: [ ------ MatSelectModule ], ------ }) export class AppModule { }
MatSelectModule
is the API reference for Angular Material select.
Create Select using <mat-select>
Angular Material Select is created using <mat-select>
which is a form control for selecting a value from a set of options. To add elements to select option, we need to use <mat-option>
element. To bind value with <mat-option>
, we need to use value
property of it. The <mat-select>
has also value
property to bind selected value to keep it selected. We need to use <mat-select>
inside <mat-form-field>
element. Find the code snippet.
<mat-form-field> <mat-label>Select student</mat-label> <mat-select> <mat-option value="101">Krishna</mat-option> <mat-option value="102">Mahesh</mat-option> <mat-option value="103">Shiva</mat-option> </mat-select> </mat-form-field>
Create Select using native <select>
with matNativeControl
Angular Material Select can also be created using native <select>
element by adding matNativeControl
attribute with it. We will keep <select>
inside <mat-form-field>
in same way as we do in <mat-select>
element. Find the code snippet.
<mat-form-field> <mat-label>Select student</mat-label> <select matNativeControl> <option value="101">Krishna</option> <option value="102">Mahesh</option> <option value="103">Shiva</option> </select> </mat-form-field>
Getting and setting with value
The value
property of <mat-select>
element is used to bind selected value selected.
Suppose we have component property.
selectedGame = "Football";
selectedGame
with value
property of <mat-select>
and then value of selectedGame
will be shown selected in select option and will change on selection change.
<mat-form-field> <mat-label>Select game</mat-label> <mat-select [(value)]="selectedGame"> <mat-option value="Hockey">Hockey</mat-option> <mat-option value="Football">Football</mat-option> <mat-option value="Cricket">Cricket</mat-option> </mat-select> </mat-form-field> <div>{{selectedGame}}</div>
ngModel
.
Getting and setting with NgModel
We can use NgModel
to get and set values in Select option for Angular Material Select as well as native Select.
Suppose we have component property.
selectedGame = "Football";
ngModel
with <mat-select>
to set and get value as following.
<mat-form-field> <mat-label>Select game</mat-label> <mat-select [(ngModel)]="selectedGame"> <mat-option value="Hockey">Hockey</mat-option> <mat-option value="Football">Football</mat-option> <mat-option value="Cricket">Cricket</mat-option> </mat-select> </mat-form-field> <div>{{selectedGame}}</div>
ngModel
with native <select>
to set and get value as following.
<mat-form-field> <mat-label>Select game</mat-label> <select matNativeControl [(ngModel)]="selectedGame"> <option value="Hockey">Hockey</option> <option value="Football">Football</option> <option value="Cricket">Cricket</option> </select> </mat-form-field> <div>{{selectedGame}}</div>
Populate Select Option Dynamically
We can populate Select option usingngFor
from an Array. Suppose we have following data in our component.
profiles = [ {id: 'dev', name: 'Developer'}, {id: 'man', name: 'Manager'}, {id: 'dir', name: 'Director'} ]; selectedProfile = this.profiles[1];
<mat-form-field> <mat-label>Select profile</mat-label> <mat-select [(value)]="selectedProfile"> <mat-option *ngFor="let p of profiles" [value]="p"> {{ p.name }} </mat-option> </mat-select> </mat-form-field> <div *ngIf="selectedProfile">{{selectedProfile.id}} - {{selectedProfile.name}}</div>
Angular Material Select and Multiple Select Example using Reactive Form
Here we will create Angular Material Select example using reactive form. We will create a form with input text, select option and multiple select option. We will populate values in select option dynamically and will fetch them on form submission. We will validate values before form submission. Now find the complete example for both Angular Material Select and native Select.select-option.component.html
<h3>Angular Material Select Demo</h3> <form [formGroup]="empForm" (ngSubmit)="onFormSubmit()"> <mat-form-field> <input matInput placeholder="Enter name" formControlName="name"> <mat-error *ngIf="name.hasError('required')"> Username is required. </mat-error> </mat-form-field> <br/> <br/> <mat-form-field> <mat-label>Select a profile</mat-label> <mat-select formControlName="profile"> <mat-option>None</mat-option> <mat-option *ngFor="let prf of allProfiles" [value]="prf"> {{ prf.name }} </mat-option> </mat-select> <mat-error *ngIf="profile.hasError('required')"> Profile is required. </mat-error> </mat-form-field> <br/> <br/> <mat-form-field> <mat-label>Select skills</mat-label> <mat-select multiple formControlName="skills"> <mat-option *ngFor="let skill of allSkills" [value]="skill"> {{skill.name}} </mat-option> </mat-select> <mat-error *ngIf="skills.hasError('required')"> Skill is required. </mat-error> </mat-form-field> <br/> <br/> <button mat-raised-button>Submit</button> <button mat-raised-button type="button" (click)="setDefault()">Default Value</button> <button mat-raised-button type="button" (click)="resetForm()">Reset</button> </form>
import { Component, OnInit } from '@angular/core'; import { FormControl, FormBuilder, Validators } from '@angular/forms'; import { Profile } from './domain/profile'; import { Skill } from './domain/skill'; import { EmployeeService } from './employee.service'; @Component({ selector: 'app-select', templateUrl: './select-option.component.html' }) export class SelectOptionComponent implements OnInit { allProfiles: Array<Profile>; allSkills: Array<Skill>; constructor(private formBuilder: FormBuilder, private empService: EmployeeService) { } ngOnInit() { this.allProfiles = this.empService.getProfiles(); this.allSkills = this.empService.getSkills(); } empForm = this.formBuilder.group({ name: ['', Validators.required], profile: ['', Validators.required], skills: [null, Validators.required] }); get name() { return this.empForm.get('name'); } get profile() { return this.empForm.get('profile'); } get skills() { return this.empForm.get('skills'); } onFormSubmit() { this.empService.saveEmployee(this.empForm.value); this.resetForm(); } setDefault() { let employee = { name: 'Amit Shah', profile: this.allProfiles[2], skills: [this.allSkills[1], this.allSkills[2]] }; this.empForm.setValue(employee); } resetForm() { this.empForm.reset(); } }
select-option-native.component.ts
<h3>Angular Material Select Demo with Native Select</h3> <form [formGroup]="empForm" (ngSubmit)="onFormSubmit()"> <mat-form-field> <input matInput placeholder="Enter name" formControlName="name"> <mat-error *ngIf="name.hasError('required')"> Username is required. </mat-error> </mat-form-field> <br/> <br/> <mat-form-field> <mat-label>Select a profile</mat-label> <select matNativeControl formControlName="profile"> <option>None</option> <option *ngFor="let prf of allProfiles" [value]="prf"> {{ prf.name }} </option> </select> <mat-error *ngIf="profile.hasError('required')"> Profile is required. </mat-error> </mat-form-field> <br/> <br/> <mat-form-field> <mat-label>Select skills</mat-label> <select matNativeControl multiple formControlName="skills"> <option *ngFor="let skill of allSkills" [value]="skill"> {{skill.name}} </option> </select> <mat-error *ngIf="skills.hasError('required')"> Skill is required. </mat-error> </mat-form-field> <br/> <br/> <button mat-raised-button>Submit</button> <button mat-raised-button type="button" (click)="setDefault()">Default Value</button> <button mat-raised-button type="button" (click)="resetForm()">Reset</button> </form>
export interface Skill { id: string; name: string; }
export interface Profile { id: string; name: string; }
import { Profile } from './profile'; import { Skill } from './skill'; export interface Employee { name: string; profile: Profile; skills: Array<Skill>; }
import { Injectable } from '@angular/core'; import { Employee } from './domain/employee'; const PROFILES = [ {id: 'dev', name: 'Developer'}, {id: 'man', name: 'Manager'}, {id: 'dir', name: 'Director'}, {id: 'tst', name: 'Tester'} ]; const SKILLS = [ {id: 'java', name: 'Java'}, {id: 'angular', name: 'Angular'}, {id: 'spring', name: 'Spring'}, {id: 'hibernate', name: 'Hibernate'}, {id: 'oracle', name: 'Oracle'} ]; @Injectable({ providedIn: 'root' }) export class EmployeeService { getProfiles() { return PROFILES; } getSkills() { return SKILLS; } saveEmployee(emp: Employee) { console.log(emp); } }
import { Component } from '@angular/core'; import { FormControl } from '@angular/forms'; @Component({ selector: 'app-root', template: ` <app-select></app-select> <app-select-native></app-select-native> ` }) export class AppComponent { }
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { FormsModule, ReactiveFormsModule } from '@angular/forms'; import { MatSelectModule, MatInputModule } from '@angular/material'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { AppComponent } from './app.component'; import { SelectOptionComponent } from './select-option.component'; import { SelectOptionNativeComponent } from './select-option-native.component'; @NgModule({ declarations: [ AppComponent, SelectOptionComponent, SelectOptionNativeComponent ], imports: [ BrowserModule, FormsModule, ReactiveFormsModule, BrowserAnimationsModule, MatSelectModule, MatInputModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Run Application
To run the application, find the steps.1. Install Angular CLI using link.
2. Install Angular Material using link.
3. Download source code using download link given below on this page.
4. Use downloaded src in your Angular CLI application.
5. Run ng serve using command prompt.
6. Access the URL http://localhost:4200
Find the print screen of the output.
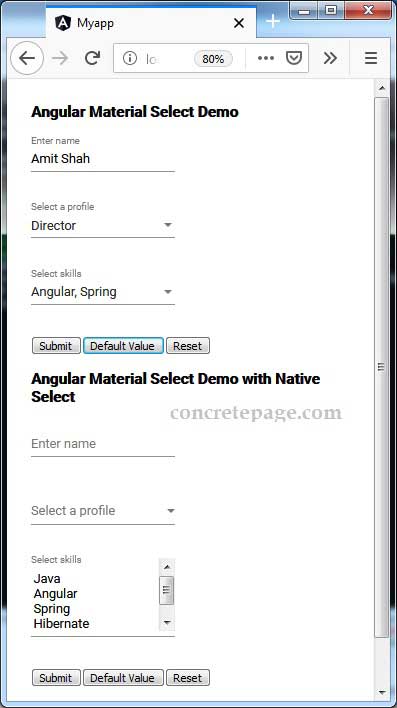