Angular Material Sidebar Menu with Submenu Example
November 20, 2022
On this page we will create Angular Material sidebar menu with submenu using Material sidenav, list and expansion panel.
1. Sidenav
The sidenav components are used to add side content to a full screen app. Sidenav are created using following components.<mat-sidenav-container> : Acts as a structural container for our content and sidenav.
<mat-sidenav> : Represents the sidenav.
<mat-sidenav-content> : Represents the main content.
<mat-sidenav-container> <mat-sidenav> ------ </mat-sidenav> <mat-sidenav-content> ------ </mat-sidenav-content> </mat-sidenav-container>
2. List
Angular Material providesmat-nav-list
for navigation list and list items are created using mat-list-item
.
<mat-nav-list> <a mat-list-item> </a> <a mat-list-item> </a> ------ </mat-nav-list>
3. Expansion Panel
The<mat-expansion-panel>
provides an expandable details-summary view. The <mat-expansion-panel-header>
shows a summary of the panel content. It contains title and description.
<mat-expansion-panel> <mat-expansion-panel-header> <mat-panel-title> Title </mat-panel-title> <mat-panel-description> Summary of the content </mat-panel-description> </mat-expansion-panel-header> Primary content of the panel </mat-expansion-panel>
<mat-sidenav-container> <mat-sidenav> <mat-nav-list> <mat-expansion-panel> <mat-expansion-panel-header> ------ </mat-expansion-panel-header> <mat-nav-list> ------ </mat-nav-list> </mat-expansion-panel> ------ </mat-nav-list> </mat-sidenav> <mat-sidenav-content> ------ </mat-sidenav-content> </mat-sidenav-container>
4. Complete Example
Find the technologies being used in our example.1. Angular 13.1.0
2. Angular Material 13.3.9
3. Node.js 12.20.0
4. NPM 8.2.0
Now find the complete code.
example-sidenav.component.html
<mat-toolbar color="primary"> <button mat-icon-button (click)="sidenav.toggle()" matTooltip="Menu"> <mat-icon>menu</mat-icon> </button> <span>ConcretePage.com</span> <span class="toolbar-item-spacer"></span> <button mat-icon-button matTooltip="Like"> <mat-icon>favorite</mat-icon> </button> <button mat-icon-button matTooltip="Share"> <mat-icon>share</mat-icon> </button> <button mat-icon-button matTooltip="Notifications"> <mat-icon>notifications</mat-icon> </button> <button mat-icon-button matTooltip="My Account" [matMenuTriggerFor]="userAccountMenu"> <mat-icon>account_circle</mat-icon> </button> <mat-menu #userAccountMenu [overlapTrigger]="false" yPosition="below"> <button mat-menu-item routerLink="#"> <mat-icon>person</mat-icon><span>My Account</span> </button> <button mat-menu-item routerLink="#"> <mat-icon>settings</mat-icon><span>Settings</span> </button> <button mat-menu-item routerLink="#"> <mat-icon>help</mat-icon><span>Help</span> </button> <mat-divider></mat-divider> <button mat-menu-item routerLink="#"> <mat-icon>exit_to_app</mat-icon>Logout </button> </mat-menu> </mat-toolbar> <mat-sidenav-container class="app-container"> <mat-sidenav #sidenav mode="side" [class.mat-elevation-z4]="true" class="app-sidenav"> <mat-nav-list> <mat-list-item routerLink="#"> <mat-icon>dashboard</mat-icon><span>Dashboard</span> </mat-list-item> <mat-expansion-panel [class.mat-elevation-z0]="true"> <mat-expansion-panel-header> Mobile Phone </mat-expansion-panel-header> <mat-nav-list> <a mat-list-item routerLink="#"> <mat-icon>apple</mat-icon><span>iOS</span> </a> <a mat-list-item routerLink="#"> <mat-icon>android</mat-icon><span>Android</span> </a> </mat-nav-list> </mat-expansion-panel> <mat-expansion-panel [class.mat-elevation-z0]="true"> <mat-expansion-panel-header> Frameworks </mat-expansion-panel-header> <mat-nav-list> <a mat-list-item routerLink="#">Spring</a> <a mat-list-item routerLink="#">Hibernate</a> <a mat-list-item routerLink="#">Struts</a> </mat-nav-list> </mat-expansion-panel> </mat-nav-list> </mat-sidenav> <mat-sidenav-content> <router-outlet> <div class="app-sidenav-content"> Components open here </div> </router-outlet> </mat-sidenav-content> </mat-sidenav-container>
import { Component } from '@angular/core'; @Component({ selector: 'example-sidenav', templateUrl: 'example-sidenav.component.html', styleUrls: ['example-sidenav.component.css'] }) export class ExampleSidenavComponent { }
.toolbar-item-spacer { flex: 1 1 auto; } .app-container { height: 90%; margin: 0; } .app-sidenav { width: 230px; } .app-sidenav-content { display: flex; height: 100%; align-items: center; justify-content: center; }
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: `<example-sidenav></example-sidenav>` }) export class AppComponent { }
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { FormsModule } from '@angular/forms'; import { MatButtonModule } from '@angular/material/button'; import { MatSidenavModule } from '@angular/material/sidenav'; import { MatMenuModule } from '@angular/material/menu'; import { MatToolbarModule } from '@angular/material/toolbar'; import { MatIconModule } from '@angular/material/icon'; import { MatListModule } from '@angular/material/list'; import { RouterModule, Routes } from '@angular/router'; import { MatExpansionModule } from '@angular/material/expansion'; import { MatTooltipModule } from '@angular/material/tooltip'; import { AppComponent } from './app.component'; import { ExampleSidenavComponent } from './example-sidenav.component'; @NgModule({ declarations: [ AppComponent, ExampleSidenavComponent, ], imports: [ BrowserModule, BrowserAnimationsModule, FormsModule, MatButtonModule, MatSidenavModule, MatMenuModule, MatToolbarModule, MatIconModule, MatListModule, RouterModule, MatExpansionModule, MatTooltipModule, RouterModule.forRoot([]) ], providers: [ ], bootstrap: [AppComponent] }) export class AppModule { }
5. Run Application
To run the application, find the steps.1. Install Angular CLI using link.
2. Install Angular Material using link.
3. Download source code using download link given below on this page.
4. Use downloaded src in your Angular CLI application.
5. Run ng serve using command prompt.
6. Access the URL http://localhost:4200
Find the print screen of the output.
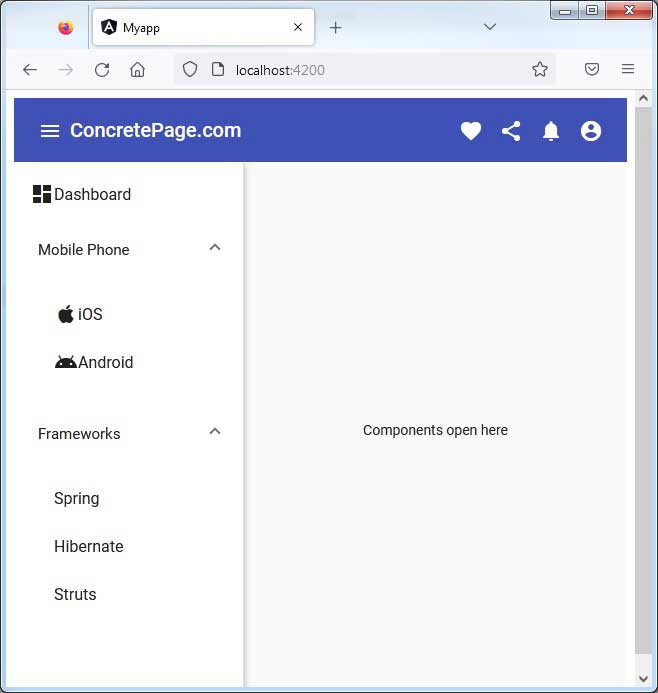
6. References
SidenavList
Expansion Panel