Angular setErrors() Example
January 23, 2024
setErrors()
sets error on a form control manually. setErrors()
can be called by the instance of FormControl
, FormGroup
and FormArray
. Calling setErrors()
on a form control also updates the validation of parent form control.
Find the method signature of
setErrors()
from Angular doc.
setErrors(errors: ValidationErrors, opts: { emitEvent?: boolean; } = {}): void
ValidationErrors
type which is a map of error key and its value.
emitEvent : If true,
statusChanges
emits an event. When emitEvent
is not provided, the default value will be true.
1. Using setErrors()
setErrors()
is called on the instances of FormControl
, FormGroup
and FormArray
.
Suppose we have a
FormGroup
as below.
customerForm = this.formBuilder.group({ customerName: '', orderNum: '' });
this.customerName.setErrors({ 'invalidName': true }); this.orderNum.setErrors({ 'invalidOrder': true });
<div *ngIf="orderNum?.hasError('invalidOrder')" class="error"> Invalid order number. </div> <div *ngIf="customerName?.hasError('invalidName')" class="error"> Invalid name. </div>
2. Clearing Errors
Errors set bysetErrors()
will clear on call of updateValueAndValidity
.
this.customerName.updateValueAndValidity(); this.orderNum.updateValueAndValidity();
3. Complete Example
customer.component.tsimport { Component, OnInit } from '@angular/core'; import { FormBuilder, Validators, ReactiveFormsModule, FormControl } from '@angular/forms'; import { CustomerService } from './customer-service'; import { CommonModule } from '@angular/common'; import { CustomerValidators } from './customer-validators'; @Component({ selector: 'app-reactive', standalone: true, imports: [CommonModule, ReactiveFormsModule], templateUrl: './customer.component.html' }) export class CustomerComponent implements OnInit { orderNumPrefixVal = "CO-"; constructor(private formBuilder: FormBuilder, private customerService: CustomerService) { } ngOnInit() { this.customerName.statusChanges.subscribe(status => { console.log("customerName: " + status); }) this.orderNum.statusChanges.subscribe(status => { console.log("orderNum: " + status); }) } customerForm = this.formBuilder.group({ customerName: ['', [Validators.minLength(3)]], orderNum: ['', [Validators.required]] }); get customerName() { return this.customerForm.get('customerName') as FormControl; } get orderNum() { return this.customerForm.get('orderNum') as FormControl; } onFormSubmit() { if (this.customerForm.valid) { this.customerService.createOrder(this.customerForm.value); this.customerForm.reset(); } else { this.customerForm.setErrors({ "errorOccured": true }); } } setCustomerErrors() { this.customerName.setErrors({ 'invalidName': true }); this.orderNum.setErrors({ 'invalidOrder': true }); } clearCustomerErrors() { this.customerName.updateValueAndValidity(); this.orderNum.updateValueAndValidity(); } }
<h3>Customer Form</h3> <form [formGroup]="customerForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td>Customer Name: </td> <td> <input formControlName="customerName"> <div *ngIf="customerName?.hasError('minLength')" class="error"> Minimum length 3. </div> <div *ngIf="customerName?.hasError('invalidName')" class="error"> Invalid name. </div> {{customerName.errors | json}} </td> </tr> <tr> <td>Order Number: </td> <td> <input formControlName="orderNum"> <div *ngIf="orderNum?.hasError('required')" class="error"> Enter order number. </div> <div *ngIf="orderNum?.hasError('invalidOrder')" class="error"> Invalid order number. </div> {{orderNum.errors | json}} </td> </tr> <tr> <td colspan="2"> <div *ngIf="customerForm?.hasError('errorOccured')" class="error"> Error occured. </div> <button>Submit</button> <button type="button" (click)="setCustomerErrors()">Add Errors</button> <button type="button" (click)="clearCustomerErrors()">Clear Errors</button> </td> </tr> </table> </form>
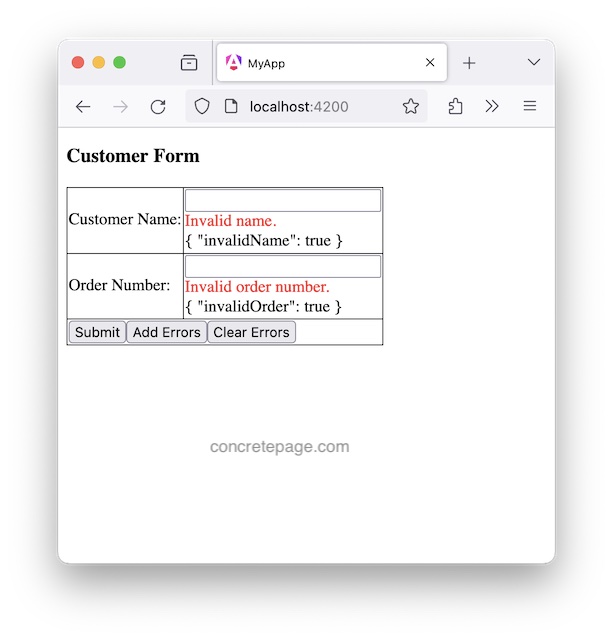