Angular Router Events
March 05, 2024
On this page we will learn about router events and use them in our Angular router application.
1. For every navigation,
Router
emits navigation events that we can fetch by subscribing Router.events
property.
2. The event
NavigationStart
occurs when navigation starts and the event NavigationEnd
occurs when navigation ends successfully.
3. The event
GuardsCheckStart
occurs when route guard check starts and the event GuardsCheckEnd
occurs when route guard check ends successfully.
4. The event
ChildActivationStart
occurs when route children begin to activate and the event ChildActivationEnd
occurs when route children activation ends.
5. The event
ActivationStart
occurs when a route is activated and the event ActivationEnd
occurs when route activation ends.
6. Angular
Event
is a type-alias for all router events that allow us to track the lifecycle of the router.
7. All the router events are imported from
@angular/router
.
Contents
1. Router Events Order
Router events are fired in following order.1. NavigationStart : When navigation starts.
2. RouteConfigLoadStart : Before the router lazy loads a route configuration.
3. RouteConfigLoadEnd : After the router has lazy loaded a route.
4. RoutesRecognized : When the URL is parsed and the routes are recognized.
5. GuardsCheckStart : When the route guards check starts.
6. ChildActivationStart : When the route children begins to activate.
7. ActivationStart : When a route is activated.
8. GuardsCheckEnd : When the route guards phase finishes successfully.
9. ResolveStart : When the Resolve phase of routing starts.
10. ResolveEnd : When the Resolve phase of routing ends successfully.
11. ChildActivationEnd : When the route children activation ends.
12. ActivationEnd : When a route activation ends.
13. NavigationEnd : When navigation ends successfully.
14. NavigationCancel : When navigation is canceled.
15. NavigationError : When navigation fails due to an unexpected error.
16. Scroll : Represents a scrolling event.
2. Subscribe to Events
To subscribe router events, we need to useRouter.events
property.
class Router { events: Observable<Event> ------ }
events
is a read-only property that consist event stream for routing events.
We can subscribe
Router.events
as below.
this.router.events.subscribe(event => { console.log(event); });
constructor(private router: Router) { } ngOnInit() { this.router.events.subscribe(event => { if (event instanceof NavigationStart) { console.log("--- NavigationStart ---"); } else if (event instanceof RouteConfigLoadStart) { console.log("--- RouteConfigLoadStart ---"); } else if (event instanceof RouteConfigLoadEnd) { console.log("--- RouteConfigLoadEnd ---"); } else if (event instanceof RoutesRecognized) { console.log("--- RoutesRecognized ---"); } else if (event instanceof GuardsCheckStart) { console.log("--- GuardsCheckStart ---"); } else if (event instanceof ChildActivationStart) { console.log("--- ChildActivationStart ---"); } else if (event instanceof ActivationStart) { console.log("--- ActivationStart ---"); } else if (event instanceof GuardsCheckEnd) { console.log("--- GuardsCheckEnd ---"); } else if (event instanceof ResolveStart) { console.log("--- ResolveStart ---"); } else if (event instanceof ResolveEnd) { console.log("--- ResolveEnd ---"); } else if (event instanceof ChildActivationEnd) { console.log("--- ChildActivationEnd ---"); } else if (event instanceof ActivationEnd) { console.log("--- ActivationEnd ---"); } else if (event instanceof NavigationEnd) { console.log("--- NavigationEnd ---"); } else if (event instanceof NavigationCancel) { console.log("--- NavigationCancel ---"); } else if (event instanceof NavigationError) { console.log("--- NavigationError ---"); } else if (event instanceof Scroll) { console.log("--- Scroll ---"); } }); }
3. Filter Events
We can filter router events using RxJSfilter
operator.
constructor(private router: Router) { } ngOnInit() { this.router.events.pipe( filter(event => event instanceof NavigationEnd) ).subscribe((e) => { console.log("--- NavigationEnd ---"); console.log(this.router.url); }); }
NavigationEnd
event.
RouterEvent Class
RouterEvent
is the base class for GuardsCheckEnd, GuardsCheckStart, NavigationCancel, NavigationEnd, NavigationError, NavigationSkipped, NavigationStart, ResolveEnd, ResolveStart, RoutesRecognized. We can filter RouterEvent
as following.
ngOnInit() { this.router.events.pipe( filter(event => event instanceof RouterEvent) ).subscribe((e) => { // Perform any task }); }
4. Using NavigationStart and NavigationEnd
Here I will useNavigationStart
and NavigationEnd
events to display loading message for a navigation. On navigation start, loading message will start displaying. Once navigation ends successfully or navigation is canceled or there is navigation error, in all these cases loading message stops.
import { Component, OnInit } from '@angular/core'; import { NavigationCancel, NavigationEnd, NavigationError, NavigationStart, Router } from '@angular/router'; @Component({ template: ` <div *ngIf="isLoading"> Loading ... </div> ------ ` }) export class PersonComponent implements OnInit { isLoading = false; constructor(private router: Router) { } ngOnInit() { this.router.events.subscribe((event) => { if (event instanceof NavigationStart) { this.isLoading = true; } else if (event instanceof NavigationEnd) { this.isLoading = false; } else if (event instanceof NavigationCancel) { this.isLoading = false; } else if (event instanceof NavigationError) { this.isLoading = false; } console.log(this.isLoading); }); } }
5. Tracing Router Events
We can trace router events for debugging purpose in our application. We need to enable tracing in our application. Once tracing is enabled, for every navigation, all events will be logged to console.1. Using enableTracing in Router Module : Use
enableTracing
of ExtraOptions
to enable tracing. ExtraOptions
is a set of configuration options for a router module. It is passed to RouterModule.forRoot()
method. enableTracing
is a boolean property and when its value is true, all internal navigation events are logged to the console.
Find the code snippet.
const routes: Routes = [ ------ ]; const extraOptions: ExtraOptions = { "enableTracing": true }; @NgModule({ imports: [ RouterModule.forRoot(routes, extraOptions) ], ------ }) export class AppRoutingModule { }
withDebugTracing()
to provideRouter()
function. provideRouter()
configures providers required for routing. withDebugTracing()
function enables logging of all internal navigation events to the console.
Find the code snippet.
const routes: Routes = []; bootstrapApplication(AppComponent, { providers: [ provideRouter(routes, withDebugTracing()) ] } );
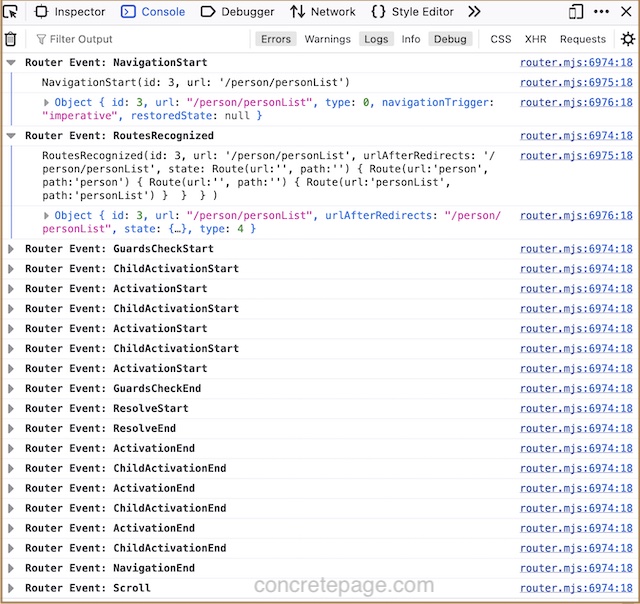