Angular Standalone – provideRouter()
January 30, 2024
On this page, we will learn to use provideRouter()
in our Angular standalone application. provideRouter()
sets provider for routing. In this method, we pass an array of routes and extra router features that need to be enabled for routing.
Now let us learn
provideRouter()
in detail.
1. provideRouter()
provideRouter()
is used to set providers for router functionality in Angular standalone application. Find its construct from Angular doc.
provideRouter(routes: Routes, ...features: RouterFeatures[]): EnvironmentProviders
features : Adds additional route behaviour. This is optional.
Find the sample code.
bootstrapApplication(AppComponent, { providers: [ provideRouter(ROUTES) ] });
Route
created for navigation.
export const ROUTES: Routes = [ ------ ];
provideRouter()
.
1. withComponentInputBinding() : Enables binding
Router
state information to @Input()
properties of component.
providers: [ provideRouter(ROUTES, withComponentInputBinding()) ]
providers: [ provideRouter(ROUTES, withDebugTracing()) ]
providers: [ provideRouter(ROUTES, withDisabledInitialNavigation()) ]
providers: [ provideRouter(ROUTES, withEnabledBlockingInitialNavigation()) ]
providers: [ provideRouter(ROUTES, withHashLocation()) ]
providers: [ provideRouter(ROUTES, withInMemoryScrolling()) ]
providers: [ provideRouter(ROUTES, withNavigationErrorHandler((e: NavigationError) => console.log(e)) ) ]
providers: [ provideRouter(ROUTES, withPreloading(PreloadAllModules)) ]
providers: [ provideRouter(ROUTES, withRouterConfig({ urlUpdateStrategy: 'eager', onSameUrlNavigation: 'reload' })) ]
We can add as many features as we want to
provideRouter()
.
providers: [ provideRouter(ROUTES, withComponentInputBinding(), withPreloading(PreloadAllModules), withDebugTracing() ) ]
2. Using withComponentInputBinding()
In standalone application, to fetch parameters from URL using@Input()
decorator, Angular provides withComponentInputBinding()
feature used with provideRouter()
.
Find the sample code.
app/app.routes.ts
import { Routes } from '@angular/router'; import { BookComponent } from './book.component'; import { WriterComponent } from './writer.component'; export const ROUTES: Routes = [ { path: 'bookdetail', component: BookComponent }, { path: 'writerdetail', component: WriterComponent }, ];
import { ApplicationConfig } from '@angular/core'; import { provideRouter, withComponentInputBinding } from '@angular/router'; import { ROUTES } from './app.routes'; export const APP_CONFIG: ApplicationConfig = { providers: [ provideRouter(ROUTES, withComponentInputBinding()), ] };
import { bootstrapApplication } from '@angular/platform-browser'; import { APP_CONFIG } from './app/app.config'; import { AppComponent } from './app/app.component'; bootstrapApplication(AppComponent, APP_CONFIG) .catch((err) => console.error(err));
import { Component } from '@angular/core'; import { RouterModule } from '@angular/router'; @Component({ selector: 'app-root', standalone: true, imports: [RouterModule], template: ` <nav> <ul> <li> <a routerLink="/bookdetail" [queryParams]="{id: 101, bname:'Ramayan'}">Book Details</a> </li> <li> <a routerLink="/writerdetail" [queryParams]="{wname:'Valmiki', city: 'Ayodhya'}">Writer Details</a> </li> </ul> </nav> <router-outlet></router-outlet> ` }) export class AppComponent { }
import { Component, Input, OnInit } from '@angular/core'; import { CommonModule } from '@angular/common'; @Component({ standalone: true, imports: [CommonModule], template: 'Book Component' }) export class BookComponent implements OnInit { @Input() id!: number; @Input() bname!: string; ngOnInit() { console.log(this.id); console.log(this.bname); } }
import { Component, Input, OnInit } from '@angular/core'; import { CommonModule } from '@angular/common'; @Component({ standalone: true, imports: [CommonModule], template: 'Writer Component' }) export class WriterComponent implements OnInit { @Input() wname!: string; @Input() city!: string; ngOnInit() { console.log(this.wname); console.log(this.city); } }
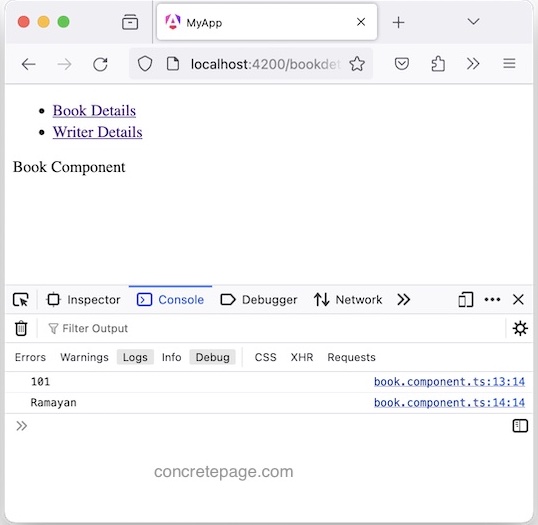