Angular @HostBinding with Examples
April 03, 2024
On this page, we will learn @HostBinding
decorator and provide examples to use it in our Angular application.
1. @HostBinding
@HostBinding
decorator binds DOM property or CSS class, style, attributes to a host element. Using change detection, Angular can automatically check host binding and then host element is updated for any binding changes.
@HostBinding
decorator accepts host property name as
Prefix class. for classes,
Prefix style. for styles and
Prefix attr. for attributes.
Lest us understand more about host binding.
1. Style binding : In style binding, style properties are bound to an element element as below.
<html_element [style.style_property] = "style_value">
@HostBinding('style.style_property') styleProperty = "style_value";
2. Class binding : In class binding, CSS classes are bound to an element as below.
<html_element [class.class_name] = "class_value">
@HostBinding('class.class_name') className = "class_value";
3. Attribute binding : In attribute binding, attributes are bound to an element as below.
<html_element [attr.attribute_name] = "attribute_value">
@HostBinding('attr.attribute_name') attributeName = "attribute_value";
@HostBinding
decorator can be used in a Directive. The element using that directive will show the effects of host binding. The values of @HostBinding
properties can be changed dynamically using @HostListener
decorator.
2. @HostBinding with Style
To use@HostBinding
with style, assign host property name with style. prefix. To use unit, we can suffix the unit name with host property name or can be used with value. Find the directive with some styles.
@Directive({ selector: '[myTheme]', standalone: true }) export class StyleBindingDirective { @HostBinding('style.color') color = "red"; @HostBinding('style.font-size.px') fontSize = 20; @HostBinding('style.width') width = "200px"; }
<div myTheme> Hello World! </div>
@HostBinding
will take effect.
In case HTML element is also using inline style, then inline style will overwrite the styles configured by
@HostBinding
.
<div style="color:green;" myTheme> Hello World! </div>
3. @HostBinding with Class
@HostBinding
uses class. prefix for class binding in host property name.
When the values of properties decorated with @HostBinding
are true, that class is applied to host element and if false, that class is removed.
Suppose I have class names as myClass1 and myClass2.
.myClass1 { color: blue; font-size: 10px; } .myClass2 { color: aqua; font-size: 20px; }
@HostBinding
as below.
@Directive({ selector: '[myTheme]', standalone: true }) export class ClassBindingDirective { @HostBinding('class.myClass1') myClass1: boolean = true; @HostBinding('class.myClass2') myClass2: boolean = false; }
<div myTheme> Hello World! </div>
If we add class inline as well as using
@HostBinding
, then inline class will overwrite the class properties configured by @HostBinding
.
<div class="myClass3" myTheme> Hello World! </div>
@HostBinding
decorator.
4. @HostBinding with Attribute
To bind attribute using@HostBinding
, use attr. prefix in host property name.
@Directive({ selector: '[myAttBind]', standalone: true }) export class AttBindingDirective { @HostBinding('attr.aria-required') @Input() required: boolean = false; @HostBinding('attr.aria-readonly') @Input() readonly: boolean = false; }
<input type="text" myAttBind/>
<input type="text" myattbind="" aria-required="false" aria-readonly="false">
@Input()
, we can also pass them values as below.
<input type="text" [required]="true" myAttBind/>
<input type="text" myattbind="" ng-reflect-required="true" aria-required="true" aria-readonly="false">
5. @HostBinding with Properties
To bind HTML element properties such as value, id, name, we need to pass property name to@HostBinding
decorator.
@Directive({ selector: '[myPropBind]', standalone: true }) export class PropBindingDirective { @HostBinding('value') elVal = "Hello World!"; @HostBinding('id') elId = "id1"; @HostBinding('name') elName = "name1"; }
<input type="text" myPropBind/>
<input type="text" myattbind="" id="id1" name="name1">
6. @HostBinding with @HostListener
The values of properties decorated with@HostBinding
can be updated dynamically using @HostListener
decorator. @HostListener
listens DOM event and is decorated on a handler method. When the host element emits that event then that handler method is executed.
Find the complete example.
1. Directive for style binding.
style-binding.directive.ts
import { Directive, HostBinding, HostListener } from '@angular/core'; @Directive({ selector: '[styleBindDemo]', standalone: true }) export class StyleBindingDirective { @HostBinding('style.background-color') bgColor = "white"; @HostBinding('style.font-size.px') fontSize = 15; @HostBinding('style.width') width = "150px"; @HostListener("focusin") handleKeyDown() { this.bgColor = "cyan"; this.fontSize = 25; this.width = "300px"; } @HostListener("focusout") handleKeyUp() { this.bgColor = "white"; this.fontSize = 15; this.width = "150px"; } }
2. CSS classes and Directive for class binding.
styles.css
.darkTheme { background-color: black; color: white; } .lightTheme { background-color: white; color: black; }
import { Directive, HostBinding, HostListener } from '@angular/core'; @Directive({ selector: '[classBindDemo]', standalone: true }) export class ClassBindingDirective { @HostBinding('class.darkTheme') darkTheme: boolean = true; @HostBinding('class.lightTheme') lightTheme: boolean = false; @HostListener('mouseover') handleMouseOver() { this.darkTheme = false; this.lightTheme = true; } @HostListener('mouseleave') handleMouseLeave() { this.darkTheme = true; this.lightTheme = false; } }
For 'mouseleave' event, the class '.darkTheme' will be added and '.lightTheme' will be removed.
3. Find the component that uses above directives.
app.component.ts
import { Component } from '@angular/core'; import { StyleBindingDirective } from './style-binding.directive'; import { ClassBindingDirective } from './class-binding.directive'; @Component({ selector: 'app-root', standalone: true, imports: [StyleBindingDirective, ClassBindingDirective], template: ` <input type="text" styleBindDemo /> <p classBindDemo> Welcome to you! </p> ` }) export class AppComponent { }
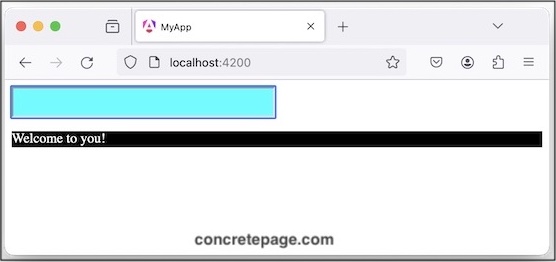