Angular Material Textarea Disabled
April 19, 2024
In Angular Material, textarea is created as <textarea matInput>
. We can disable textarea in following ways.
1. Using
disabled
attribute with <textarea>
element.
2. Pass
disabled:true
to FormControl
while creating its object.
3. Enable and disable textarea using
enable()
and disable()
methods of FormControl
.
1. Using 'disabled' Attribute
To disable textarea, usedisabled
attribute.
<textarea matInput disabled></textarea>
TS code:
isDisabled = true;
<textarea matInput [disabled]="isDisabled"></textarea>
2. Using enable() and disable() of FormControl
We can disable aFormControl
by passing disabled
value as true.
TS code:
msg = new FormControl({ value: "", disabled: true });
<textarea matInput [formControl]="msg"></textarea>
FormControl
using its enable()
and disable()
methods.
1. Enables the textarea to edit.
this.msg?.enable();
this.msg?.disable();
3. With FormGroup
To disable textarea in a form created withFormGroup
, write code as below.
TS code:
userForm = this.formBuilder.group({ desc: [{ value: "", disabled: true }] });
<textarea matInput formControlName="desc"></textarea>
enable()
and disable()
methods of FormControl
.
get desc() { return this.userForm.get('desc'); } descEnable() { this.desc?.enable(); } descDisable() { this.desc?.disable(); }
4. Complete Example
user.component.html<h3>1. With NgModel</h3> <mat-form-field> <mat-label>Comment: </mat-label> <textarea matInput [disabled]="isDisabled" [(ngModel)]="comment"></textarea> </mat-form-field> <button (click)="commentEnable()">Enable</button> <button (click)="commentDisable()">Disable</button> <h3>2. With FormControl</h3> <mat-form-field> <mat-label>Message: </mat-label> <textarea matInput [formControl]="msg"></textarea> </mat-form-field> <button (click)="msgEnable()">Enable</button> <button (click)="msgDisable()">Disable</button> <h3>3. With FormGroup</h3> <form [formGroup]="userForm" (ngSubmit)="onFormSubmit()"> <mat-form-field> <mat-label>Description: </mat-label> <textarea matInput formControlName="desc"></textarea> </mat-form-field> <button (click)="descEnable()">Enable</button> <button (click)="descDisable()">Disable</button> </form>
import { Component } from '@angular/core'; import { MatInputModule } from '@angular/material/input'; import { MatFormFieldModule } from '@angular/material/form-field'; import { FormBuilder, FormControl, FormsModule, ReactiveFormsModule, Validators } from '@angular/forms'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-user', standalone: true, imports: [MatFormFieldModule, MatInputModule, FormsModule, ReactiveFormsModule, CommonModule], templateUrl: './user.component.html' }) export class UserComponent { comment = ""; isDisabled = true; commentEnable() { this.isDisabled = false; } commentDisable() { this.isDisabled = true; } msg = new FormControl({ value: "", disabled: true }, [Validators.maxLength(100)]); msgEnable() { this.msg?.enable(); } msgDisable() { this.msg?.disable(); } constructor(private formBuilder: FormBuilder) { } userForm = this.formBuilder.group({ desc: [{ value: "", disabled: true }, [Validators.maxLength(100)]] }); get desc() { return this.userForm.get('desc'); } descEnable() { this.desc?.enable(); } descDisable() { this.desc?.disable(); } onFormSubmit() { } }
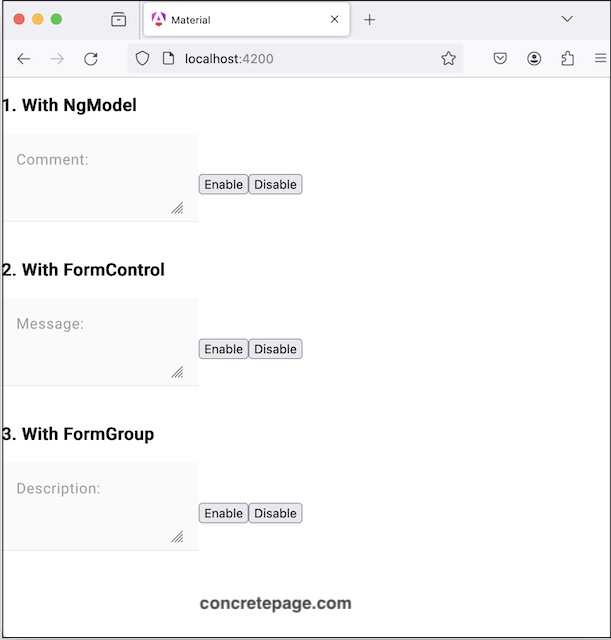