Angular Material Radio Button
September 10, 2018
This page will walk through Angular Material radio button example. To work with Angular Material radio button we need to import MatRadioModule
in application module. Angular provides MatRadioGroup
to group radio buttons. The selector of MatRadioGroup
is mat-radio-group
. Radio button is created using MatRadioButton
and its selector is mat-radio-button
. MatRadioButton
creates radio button enhanced with Material design styling and animations. A mat-radio-group
may contain one or more than one mat-radio-button
. The name provided to mat-radio-group
is used by all radio buttons inside that group. MatRadioChange
is emitted by change
event of MatRadioButton
and MatRadioGroup
. MatRadioChange
is used to fetch selected radio button.
On this page we will discuss properties of
MatRadioGroup
and MatRadioButton
and their uses, perform radio button validation and will create reactive and template-driven form using Angular Material radio buttons.
Contents
- 1. Technologies Used
- 2. Import MatRadioModule
- 3. Using MatRadioGroup
- 4. Using MatRadioButton
- 5. Using MatRadioChange
- 6. Validation
- 7. Create <mat-radio-button> dynamically using ngFor
- 8. Reactive Form Example using Radio Buttons
- 9. Template-Driven Form Example using Radio Buttons
- 10. Run Application
- 11. References
- 12. Download Source Code
1. Technologies Used
Find the technologies being used in our example.1. Angular 6.1.0
2. Angular CLI 6.1.3
3. Angular Material 6.4.7
4. TypeScript 2.7.2
5. Node.js 10.3.0
6. NPM 6.1.0
2. Import MatRadioModule
To work with Angular Material radio button we need to importMatRadioModule
in application module.
import { MatRadioModule } from '@angular/material/radio'; @NgModule({ imports: [ ------ MatRadioModule ], ------ }) export class AppModule { }
3. Using MatRadioGroup
MatRadioGroup
is a group of radio buttons. The selector of MatRadioGroup
is mat-radio-group
. Radio button is created using MatRadioButton
and its selector is mat-radio-button
. A mat-radio-group
may contain one or more than one mat-radio-button
. Find the sample example.
<mat-radio-group> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group>
MatRadioGroup
provides properties such as disabled, labelPosition, name, required, selected, value and change. We will discuss here some of them.
3.1. disabled
disabled
is used to make a mat-radio-group
disabled. It is declared in MatRadioGroup
as following.
@Input() disabled: Boolean
disabled
with mat-radio-group
in HTML template.
<mat-radio-group disabled> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group>
isDisabled= true;
<mat-radio-group [disabled]="isDisabled"> ------ </mat-radio-group>
3.2. labelPosition
labelPosition
decides if labels should appear before or after the radio buttons. It is declared in MatRadioGroup
as following.
@Input() labelPosition: 'before' | 'after'
labelPosition
with mat-radio-group
in HTML template.
<mat-radio-group labelPosition="before"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group>
labelPosition="before"
with mat-radio-group
will cause to appear label before radio button for all radio buttons inside mat-radio-group
.
3.3. name
name
is the name of radio button group. This name is used by all radio buttons inside this group. It is declared in MatRadioGroup
as following.
@Input() name: string
name
with mat-radio-group
in HTML template.
<mat-radio-group name="gender"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group>
3.4. required
required
is used with radio group for required validation. It is declared in MatRadioGroup
as following.
@Input() required: Boolean
required
with mat-radio-group
in HTML template.
<mat-radio-group name="gender" ngModel required #gender="ngModel"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group> <br/>{{gender.valid}} <br/>{{gender.value}}
3.5. change
change
event emits MatRadioChange
when a group value changes by user interaction. It is declared in MatRadioGroup
as following.
@Output() change: EventEmitter<MatRadioChange>
change
event.
<mat-radio-group (change)="onChange($event)" name="gender"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group>
onChange(mrChange: MatRadioChange) { console.log(mrChange.value); let mrButton: MatRadioButton = mrChange.source; console.log(mrButton.name); console.log(mrButton.checked); console.log(mrButton.inputId); }
value
and source
are the properties of MatRadioChange
.
4. Using MatRadioButton
MatRadioButton
creates radio button enhanced with Material design styling and animations. The selector of MatRadioButton
is mat-radio-button
that works same as <input type="radio">
. All radio buttons with same name
creates a set and we can select only one of them. To make a group of radio buttons, all mat-radio-button
are placed inside mat-radio-group
. MatRadioButton
provides properties such as ariaDescribedby, ariaLabel, ariaLabelledby, checked, color, disableRipple, disabled, id, labelPosition, name, required, value, change, inputId, radioGroup. We will discuss here some of them.
4.1. checked
Usingchecked
with mat-radio-button
, we make a radio button checked by default. It is declared in MatRadioButton
as following.
@Input() checked: Boolean
checked
with mat-radio-button
in HTML template.
<mat-radio-group (change)="onSelectionChange()"> <mat-radio-button value="m" checked>Male</mat-radio-button> <mat-radio-button value="f" #female>Female</mat-radio-button> </mat-radio-group>
Male
radio button will be checked by default.
Using
checked
property in TS file, we can know if a radio button is in checked state or not.
@ViewChild('female') femaleRB: MatRadioButton; onSelectionChange() { console.log(this.femaleRB.checked); }
Female
radio button, we will get true
value.
4.2. color
color
assigns theme color palette for the component. It is declared in MatRadioButton
as following.
@Input() color: ThemePalette
color
with mat-radio-button
in HTML template.
<mat-radio-group> <mat-radio-button value="1" color="primary">Email</mat-radio-button> <mat-radio-button value="2" color="accent">Mobile</mat-radio-button> <mat-radio-button value="3" color="warn">Both</mat-radio-button> </mat-radio-group>
deeppurple-amber.css
pre-built theme in styles.css
file.
4.3. disableRipple
disableRipple
is used to disable ripples. We see ripples while clicking on radio buttons. It is declared in MatRadioButton
as following.
@Input() disableRipple: Boolean
disableRipple
with mat-radio-button
in HTML template.
<mat-radio-button value="f" disableRipple>Female</mat-radio-button>
disableRipple
to enable/disable ripples dynamically.
<mat-radio-button value="f" [disableRipple]="true">Female</mat-radio-button>
4.4. disabled
disabled
is used to disable the radio button. It is declared in MatRadioButton
as following.
@Input() disabled: Boolean
disabled
with mat-radio-button
in HTML template.
<mat-radio-button value="f" disabled>Female</mat-radio-button>
disabled
to enable/disable radio button dynamically.
<mat-radio-button value="f" [disabled]="true">Female</mat-radio-button>
4.5. id
id
is used to assign a unique id for radio button. It is declared in MatRadioButton
as following.
@Input() id: string
id
with mat-radio-button
in HTML template.
<mat-radio-button value="f" id="femGenId">Female</mat-radio-button>
4.6. labelPosition
labelPosition
defines whether label should appear before or after the radio button. Default value is after
. It is declared in MatRadioButton
as following.
@Input() labelPosition: 'before' | 'after'
labelPosition
with mat-radio-button
in HTML template.
<mat-radio-group> <mat-radio-button value="m" labelPosition="before">Male</mat-radio-button> <mat-radio-button value="f" labelPosition="before">Female</mat-radio-button> </mat-radio-group>
4.7. name
name
is analog to HTML name
attribute. It is used to group radio buttons for unique selection. It is declared in MatRadioButton
as following.
@Input() name: string
name
with mat-radio-button
in HTML template.
<mat-radio-group> <mat-radio-button name="gender" value="m" labelPosition="before">Male</mat-radio-button> <mat-radio-button name="gender" value="f" labelPosition="before">Female</mat-radio-button> </mat-radio-group>
4.8. value
value
defines the value of radio button. It is declared in MatRadioButton
as following.
@Input() value: any
value
with mat-radio-button
in HTML template.
<mat-radio-group name="gender" ngModel #gender="ngModel"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group> <br/>{{gender.value}}
Male
radio button, we will get value as m
and for Female
, value will be f
.
4.9. change
change
event emits MatRadioChange
when the checked state of the radio button changes by user interaction. It is declared in MatRadioButton
as following.
@Output() change: EventEmitter<MatRadioChange>
change
is used with radio button as following.
<mat-radio-button value="f" (change)="onChange($event)">Female</mat-radio-button>
onChange(mrChange: MatRadioChange) { console.log(mrChange.value); let mrButton: MatRadioButton = mrChange.source; console.log(mrButton.name); console.log(mrButton.checked); console.log(mrButton.inputId); console.log(mrButton.radioGroup.name); }
inputId
and radioGroup
are the properties of MatRadioButton
. inputId
gives the id of native input element inside <mat-radio-button>
and radioGroup
gives parent radio group if present.
4.10. focus()
focus()
method of MatRadioButton
focuses the radio button. Suppose we have following radio buttons.
<mat-radio-group> <mat-radio-button value="1" #email>Email</mat-radio-button> <mat-radio-button value="2">Mobile</mat-radio-button> </mat-radio-group> <br/> <button mat-raised-button (click)="focusOnEmailRB()">Focus on Email</button>
focus()
method as following.
@ViewChild('email') emailRB: MatRadioButton; focusOnEmailRB() { this.emailRB.focus(); }
5. Using MatRadioChange
MatRadioChange
is emitted by change
event of MatRadioButton
and MatRadioGroup
. MatRadioChange
has following properties.
source: This is the object of
MatRadioButton
emitted by change
event.
value: This is the value of
MatRadioButton
.
Here we will provide sample example of
MatRadioChange
with change
event of MatRadioGroup
. First find the HTML template using change
event.
<mat-radio-group (change)="onChange($event)" name="gender"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group>
MatRadioChange
.
onChange(mrChange: MatRadioChange) { console.log(mrChange.value); let mrButton: MatRadioButton = mrChange.source; console.log(mrButton.name); console.log(mrButton.checked); console.log(mrButton.inputId); }
6. Validation
We can validate radio button for required validation usingValidators.required
with FormControl
and using required
property of MatRadioGroup
. Find the sample code.
a. Using Validators.required with FormControl
Create a
FormControl
object in TS file.
notificationTypeFC= new FormControl('', Validators.required);
<mat-radio-group [formControl]="notificationTypeFC"> <mat-radio-button value="1">Email</mat-radio-button> <mat-radio-button value="2">Mobile</mat-radio-button> <mat-radio-button value="3">Both</mat-radio-button> </mat-radio-group> <div *ngIf="notificationTypeFC.hasError('required')" class="error"> Notification Type is required. </div>
<mat-radio-group name="gender" ngModel required #gender="ngModel"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group> <div *ngIf="gender.hasError('required')" class="error"> Gender selection is required. </div>
7. Create <mat-radio-button> dynamically using ngFor
To create radio buttons dynamically we can usengFor
. Suppose we have an array in TS file as following.
languages: Language[] = [ {id: 'H', name: 'Hindi'}, {id: 'E', name: 'English'} ];
ngFor
.
<mat-radio-group formControlName="language" class="person-radio-group"> <mat-radio-button class="person-radio-button" *ngFor="let lang of languages" [value]="lang.id"> {{lang.name}} </mat-radio-button> </mat-radio-group>
8. Reactive Form Example using Radio Buttons
Find the complete code to create radio buttons using reactive form.reactive-form.component.html
<mat-radio-group (change)="onChange($event)" name="gender"> <mat-radio-button value="m">Male</mat-radio-button> <mat-radio-button value="f">Female</mat-radio-button> </mat-radio-group> <br/><br/> <mat-radio-group> <mat-radio-button value="1" #email>Email</mat-radio-button> <mat-radio-button value="2">Mobile</mat-radio-button> </mat-radio-group> <br/> <button mat-raised-button (click)="focusOnEmailRB()">Focus on Email</button> <h3>Person Reactive Form</h3> <form [formGroup]="personForm" (ngSubmit)="onFormSubmit()" #psForm="ngForm"> <div> <mat-form-field> <input matInput placeholder="Name" formControlName="name"> <mat-error *ngIf="name.hasError('required')"> Name is required. </mat-error> </mat-form-field> </div> <div> Notification Type *: <mat-radio-group formControlName="notificationType"> <mat-radio-button value="1" color="primary" class="person-radio-button">Email</mat-radio-button> <mat-radio-button value="2" color="accent" class="person-radio-button">Mobile</mat-radio-button> <mat-radio-button value="3" color="warn" class="person-radio-button">Both</mat-radio-button> </mat-radio-group> <div *ngIf="notificationType.hasError('required') && psForm.submitted" class="error"> Notification Type is required. </div> </div> <div> Language: <mat-radio-group formControlName="language" class="person-radio-group"> <mat-radio-button class="person-radio-button" *ngFor="let lang of languages" [value]="lang.id"> {{lang.name}} </mat-radio-button> </mat-radio-group> </div> <div> <button mat-raised-button>Submit</button> </div> </form>
import { Component, OnInit, ViewChild } from '@angular/core'; import { Validators, FormBuilder } from '@angular/forms'; import { MatRadioButton, MatRadioChange } from '@angular/material/radio'; import { PersonService } from './person.service'; import { Language } from './person'; @Component({ selector: 'app-reactive', templateUrl: './reactive-form.component.html' }) export class ReactiveFormComponent implements OnInit { constructor(private formBuilder: FormBuilder, private personService: PersonService) {} onChange(mrChange: MatRadioChange) { console.log(mrChange.value); let mrButton: MatRadioButton = mrChange.source; console.log(mrButton.name); console.log(mrButton.checked); console.log(mrButton.inputId); } @ViewChild('email') emailRB: MatRadioButton; focusOnEmailRB() { this.emailRB.focus(); } languages: Language[]; ngOnInit() { this.languages = this.personService.getLanguages(); } //Create a form personForm = this.formBuilder.group({ name: ['', Validators.required], notificationType: ['', Validators.required], language: '' }); onFormSubmit() { this.personService.savePerson(this.personForm.value); } get name() { return this.personForm.get('name'); } get notificationType() { return this.personForm.get('notificationType'); } }
export interface Person { name: string; notificationType: string; language: Language; } export interface Language { id: string; name: string; }
import { Injectable } from '@angular/core'; import { Person, Language } from './person'; const LANGUAGES: Language[] = [ {id: 'H', name: 'Hindi'}, {id: 'E', name: 'English'}, {id: 'M', name: 'Marathi'}, {id: 'T', name: 'Tamil'} ]; @Injectable({ providedIn: 'root' }) export class PersonService { getLanguages() { return LANGUAGES; } savePerson(person: Person) { console.log(person); } }
9. Template-Driven Form Example using Radio Buttons
Find the complete code to create radio buttons using template-driven form.template-driven-form.component.html
<h3>Person Template-Driven Form</h3> <form #personForm="ngForm" (ngSubmit)="onFormSubmit(personForm)"> <div> <mat-form-field> <input matInput placeholder="Name" name="name" required ngModel #name="ngModel"> <mat-error *ngIf="name.hasError('required')"> Name is required. </mat-error> </mat-form-field> </div> <div> Notification Type *: <mat-radio-group name="notificationType" ngModel required #notificationType="ngModel"> <mat-radio-button value="1" color="primary" class="person-radio-button">Email</mat-radio-button> <mat-radio-button value="2" color="accent" class="person-radio-button">Mobile</mat-radio-button> <mat-radio-button value="3" color="warn" class="person-radio-button">Both</mat-radio-button> </mat-radio-group> <div *ngIf="notificationType.hasError('required') && personForm.submitted" class="error"> Notification Type is required. </div> </div> <div> Language: <mat-radio-group name="language" ngModel class="person-radio-group"> <mat-radio-button class="person-radio-button" *ngFor="let lang of languages" [value]="lang.id"> {{lang.name}} </mat-radio-button> </mat-radio-group> </div> <div> <button mat-raised-button>Submit</button> </div> </form>
import { Component, OnInit } from '@angular/core'; import { PersonService } from './person.service'; import { Language } from './person'; @Component({ selector: 'app-template-driven', templateUrl: './template-driven-form.component.html' }) export class TemplateDrivenFormComponent implements OnInit { languages: Language[]; constructor(private personService: PersonService) {} ngOnInit() { this.languages = this.personService.getLanguages(); } onFormSubmit(form) { this.personService.savePerson(form.value); } }
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <app-reactive></app-reactive> <app-template-driven></app-template-driven> ` }) export class AppComponent { }
import { BrowserModule } from '@angular/platform-browser'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { NgModule } from '@angular/core'; import { FormsModule, ReactiveFormsModule } from '@angular/forms'; import { MatFormFieldModule } from '@angular/material/form-field'; import { MatInputModule } from '@angular/material/input'; import { MatButtonModule } from '@angular/material/button'; import { MatRadioModule } from '@angular/material/radio'; import { AppComponent } from './app.component'; import { ReactiveFormComponent } from './reactive-form.component'; import { TemplateDrivenFormComponent } from './template-driven-form.component'; @NgModule({ declarations: [ AppComponent, ReactiveFormComponent, TemplateDrivenFormComponent ], imports: [ BrowserModule, BrowserAnimationsModule, FormsModule, ReactiveFormsModule, MatFormFieldModule, MatInputModule, MatButtonModule, MatRadioModule ], providers: [ ], bootstrap: [AppComponent] }) export class AppModule { }
@import '~@angular/material/prebuilt-themes/deeppurple-amber.css'; .person-radio-group { display: inline-flex; flex-direction: column; } .person-radio-button { margin: 6px; } .error { color: red; }
10. Run Application
To run the application, find the steps.1. Install Angular CLI using link.
2. Install Angular Material using link.
3. Download source code using download link given below on this page.
4. Use downloaded src in your Angular CLI application.
5. Run ng serve using command prompt.
6. Access the URL http://localhost:4200
Find the print screen of the output.
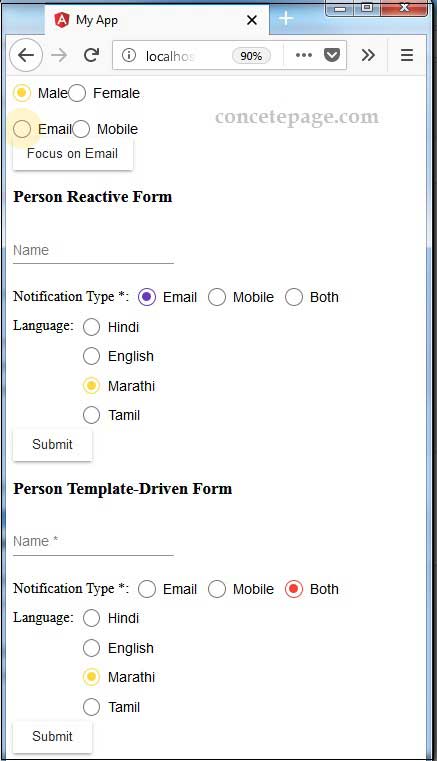
11. References
API reference for Angular Material radioAngular Tutorials