Angular Radio Button and Checkbox Example
March 24, 2020
This page will walk through Angular radio button and checkbox example. We will provide demo using template-driven form and reactive form. Using both type of form we will see how to create radio button and checkbox, fetch values and set them checked and validate them. Validation of radio button means at least one option must be checked and validation of checkbox means checkbox must be checked. We will create two separate components, one for template-driven form and another for reactive form in our application. Template-driven approach uses NgForm
and NgModel
directive. Reactive form uses FormGroup
and FormControl
classes to handle form elements. Now let us discuss complete example step by step.
Contents
- Technologies Used
- Project Structure
- Using Template-Driven Form
- 1. Creating Radio Button and Checkbox
- 2. Fetch Value on Form Submit
- 3. Set Radio Button and Checkbox Checked
- 4. Validation
- Using Reactive Form
- 1. Creating Radio Button and Checkbox
- 2. Fetch Value on Form Submit
- 3. Set Radio Button and Checkbox Checked
- 4. Validation
- Complete Example
- Run Application
- References
- Download Source Code
Technologies Used
Find the technologies being used in our example.1. Angular 9.0.2
2. Node.js 12.5.0
3. NPM 6.9.0
Project Structure
Find the project structure of our example.angular-demo | |--src | | | |--app | | | | | |--domain | | | | | | | |--user.ts | | | | | |--services | | | | | | | |--user-service.ts | | | | | |--template-driven-form.component.ts | | |--reactive-form.component.ts | | |--template-driven-form.component.html | | |--reactive-form.component.html | | |--style.css | | | | | |--app.component.ts | | |--app.module.ts | | | |--main.ts | |--index.html | |--styles.css | |--node_modules |--package.json
Using Template-Driven Form
We will use template-driven from to create radio button and checkbox here. To work with template-driven from we need to useNgForm
and NgModel
directive. To enable it in our application we need to configure FormsModule
in our application module.
Now we will create radio button and checkbox using template-driven from. We will see how to fetch values and validate them. Suppose we have a class whose fields will be populated by the form elements.
export class User { userName: string; gender: string; isMarried: boolean = false; isTCAccepted: boolean; constructor() { } }
1. Creating Radio Button and Checkbox
We need to usengModel
as an attribute in radio button and checkbox so that they can be registered with NgForm
directive.
Create button
<input type="radio" value="male" name="gender" ngModel> Male <input type="radio" value="female" name="gender" ngModel> Female
<input type="checkbox" name="tc" ngModel>
2. Fetch Value on Form Submit
When we submit the form and suppose the form value is stored in the instance ofNgForm
as form
then we can fetch radio button and checkbox value using form.controls[...]
as given below.
this.user.gender = form.controls['gender'].value; this.user.isTCAccepted = form.controls['tc'].value;
gender
is the radio button name and tc
is the checkbox name.
3. Set Radio Button and Checkbox Checked
To set radio button and checkbox checked we need to use property binding. Find the code.<p> <input type="radio" value="male" name="gender" [ngModel]="user.gender" > Male <input type="radio" value="female" name="gender" [ngModel]="user.gender" > Female </p> <p> <input type="checkbox" name="tc" [ngModel]="user.isTCAccepted" > </p>
user.gender
has value as male
then that radio button will be checked. For the checkbox if user.isTCAccepted
has value as true
then checkbox will be checked.
4. Validation
Suppose we wantrequired
validation with radio button.
<p> <input type="radio" value="male" name="gender" required #gender="ngModel"> Male <input type="radio" value="female" name="gender"required #gender="ngModel"> Female </p>
<div *ngIf="gender.invalid"> Gender required. </div>
required
validation with checkbox.
<p> <input type="checkbox" name="tc" required #tc="ngModel"> </p>
<div *ngIf="tc.invalid> Accept T & C. </div>
Using Reactive Form
We will use reactive form to create radio button and checkbox here. We will provide how to use them, set default value and validate them. In reactive form we need to useFormGroup
and FormControl
from @angular/forms
library. To enable reactive form in our application, we need to configure ReactiveFormsModule
in our application module.
1. Creating Radio Button and Checkbox
To create radio button and checkbox we will create an instance ofFormGroup
that will have properties of FormControl
.
userForm = new FormGroup({ gender: new FormControl(), tc: new FormControl() });
formControlName
to map form elements.
<form [formGroup]="userForm" (ngSubmit)="onFormSubmit()"> <p> <input type="radio" value="male" formControlName="gender"> Male <input type="radio" value="female" formControlName="gender"> Female </p> <p> <input type="checkbox" formControlName="tc"> </p> </form>
2. Fetch Value on Form Submit
When form is submitted, we can fetch radio button and checkbox values usingFormGroup
instance, for example userForm
. To fetch values we need to call get()
method of FormGroup
and pass the name of form control to this method as follows.
this.user.gender = this.userForm.get('gender').value; this.user.isTCAccepted = this.userForm.get('tc').value;
3. Set Radio Button and Checkbox Checked
To set a value in radio button and checkbox, we can use two methods ofFormGroup
.
setValue()
: It sets values to all form controls.
patchValue()
: It sets values to selected form controls.
For the example we are using
patchValue()
here.
setDefaultValues() { this.userForm.patchValue({gender:'male', tc:true}); }
male
and checkbox will also be checked.
4. Validation
To validate radio button and checkbox, we need to useValidators
from @angular/forms
library. It is used while instantiating FormGroup
.
userForm = new FormGroup({ gender: new FormControl('', Validators.required), tc: new FormControl('', Validators.requiredTrue) });
Validators.required
for radio button and Validators.requiredTrue
for checkbox. It means if we do not select any radio button or checkbox is not checked then form will not be valid. In reactive form Validators.requiredTrue
validates a control for true
value and we commonly use it for required checkboxes. To display validation message in HTML template, we can write code as follows.
<div *ngIf="userForm.get('gender').invalid"> Gender required. </div> <div *ngIf="userForm.get('tc').invalid"> Accept T & C. </div>
Complete Example
user.tsexport class User { userName: string; gender: string; isMarried: boolean = false; isTCAccepted: boolean; constructor() { } }
import { Injectable } from '@angular/core'; import { User } from '../domain/user'; @Injectable() export class UserService { createUser(user: User) { //Log user data in console console.log("User Name: " + user.userName); console.log("Gender: " + user.gender); console.log("Married?: " + user.isMarried); console.log("T & C accepted?: " + user.isTCAccepted); } }
import { Component, OnInit } from '@angular/core'; import { NgForm } from '@angular/forms'; import { UserService } from './services/user-service'; import { User } from './domain/user'; @Component({ selector: 'app-template', templateUrl: './template-driven-form.component.html', styleUrls: ['./style.css'] }) export class TemplateDrivenFormComponent { isValidFormSubmitted = false; user = new User(); constructor(private userService: UserService) { } onFormSubmit(form: NgForm) { this.isValidFormSubmitted = false; if(form.invalid){ return; } this.isValidFormSubmitted = true; this.user.userName = form.controls['uname'].value; this.user.gender = form.controls['gender'].value; this.user.isMarried = form.controls['married'].value; this.user.isTCAccepted = form.controls['tc'].value; this.userService.createUser(this.user); this.resetForm(form); } resetForm(form: NgForm) { this.user = new User(); form.resetForm({ married: false }); } setDefaultValues() { this.user.userName = 'Krishna'; this.user.gender = 'male'; this.user.isMarried = true; this.user.isTCAccepted = false; } }
<h3>Template Driven Form</h3> <p *ngIf="isValidFormSubmitted" [ngClass] = "'success'"> Form submitted successfully. </p> <form #userForm="ngForm" (ngSubmit)="onFormSubmit(userForm)"> <table> <tr> <td>Name:</td> <td> <input name="uname" [ngModel]="user.userName" required #uname="ngModel"> <div *ngIf="uname.invalid && userForm.submitted && !isValidFormSubmitted" [ngClass] = "'error'"> Name required. </div> </td> </tr> <tr> <td>Gender:</td> <td> <input type="radio" value="male" name="gender" [ngModel]="user.gender" required #gender="ngModel"> Male <input type="radio" value="female" name="gender" [ngModel]="user.gender" required #gender="ngModel"> Female <div *ngIf="gender.invalid && userForm.submitted && !isValidFormSubmitted" [ngClass] = "'error'"> Gender required. </div> </td> </tr> <tr> <td>Are you married? </td> <td> <input type="checkbox" name="married" [ngModel]="user.isMarried"> </td> </tr> <tr> <td>Accept T & C </td> <td> <input type="checkbox" name="tc" [ngModel]="user.isTCAccepted" required #tc="ngModel"> <div *ngIf="tc.invalid && userForm.submitted && !isValidFormSubmitted" [ngClass] = "'error'"> Accept T & C. </div> </td> </tr> <tr> <td colspan="2"> <button>Submit</button> <button type="button" (click)="setDefaultValues()">Set Default</button> <button type="button" (click)="resetForm(userForm)">Reset</button> </td> </tr> </table> </form>
table { border-collapse: collapse; } table, th, td { border: 1px solid black; } .error { color: red; } .success { color: green; }
import { Component, OnInit } from '@angular/core'; import { FormControl, FormGroup, Validators } from '@angular/forms'; import { UserService } from './services/user-service'; import { User } from './domain/user'; @Component({ selector: 'app-reactive', templateUrl: './reactive-form.component.html', styleUrls: ['./style.css'] }) export class ReactiveFormComponent { isValidFormSubmitted: boolean = null; userForm = new FormGroup({ uname: new FormControl('', Validators.required), gender: new FormControl('', Validators.required), married: new FormControl(false), tc: new FormControl('', Validators.requiredTrue) }); user = new User(); constructor(private userService: UserService) { } onFormSubmit() { this.isValidFormSubmitted = false; if(this.userForm.invalid){ return; } this.isValidFormSubmitted = true; console.log(this.userForm.valid); this.user.userName = this.userForm.get('uname').value; this.user.gender = this.userForm.get('gender').value; this.user.isMarried = this.userForm.get('married').value; this.user.isTCAccepted = this.userForm.get('tc').value; this.userService.createUser(this.user); this.reset(); } reset() { this.userForm.reset({ married: false }); } setDefaultValues() { this.userForm.patchValue({uname: 'Krishna', gender:'male', married:true}); } }
<h3>Reactive Form</h3> <p *ngIf="isValidFormSubmitted && userForm.pristine" [ngClass] = "'success'"> Form submitted successfully. </p> <form [formGroup]="userForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td>Name:</td> <td> <input formControlName="uname"> <div *ngIf="userForm.get('uname').invalid && isValidFormSubmitted != null && !isValidFormSubmitted" [ngClass] = "'error'"> Name required. </div> </td> </tr> <tr> <td>Gender:</td> <td> <input type="radio" value="male" formControlName="gender"> Male <input type="radio" value="female" formControlName="gender"> Female <div *ngIf="userForm.get('gender').invalid && isValidFormSubmitted != null && !isValidFormSubmitted" [ngClass] = "'error'"> Gender required. </div> </td> </tr> <tr> <td>Are you married? </td> <td> <input type="checkbox" formControlName="married"> </td> </tr> <tr> <td>Accept T & C </td> <td> <input type="checkbox" formControlName="tc"> <div *ngIf="userForm.get('tc').invalid && isValidFormSubmitted != null && !isValidFormSubmitted" [ngClass] = "'error'"> Accept T & C. </div> </td> </tr> <tr> <td colspan="2"> <button>Submit</button> <button type="button" (click)="setDefaultValues()">Set Default</button> <button type="button" (click)="reset()">Reset</button> </td> </tr> </table> </form>
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <app-template></app-template> <app-reactive></app-reactive> ` }) export class AppComponent { }
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule, ReactiveFormsModule } from '@angular/forms'; import { AppComponent } from './app.component'; import { TemplateDrivenFormComponent } from './template-driven-form.component'; import { ReactiveFormComponent } from './reactive-form.component'; import { UserService } from './services/user-service'; @NgModule({ imports: [ BrowserModule, FormsModule, ReactiveFormsModule ], declarations: [ AppComponent, TemplateDrivenFormComponent, ReactiveFormComponent ], providers: [ UserService ], bootstrap: [ AppComponent ] }) export class AppModule { }
Run Application
To run the application, find the steps.1. Download source code using download link given below on this page.
2. Use downloaded src in your Angular CLI application. To install Angular CLI, find the link.
3. Run ng serve using command prompt.
4. Access the URL http://localhost:4200
Find the print screen.
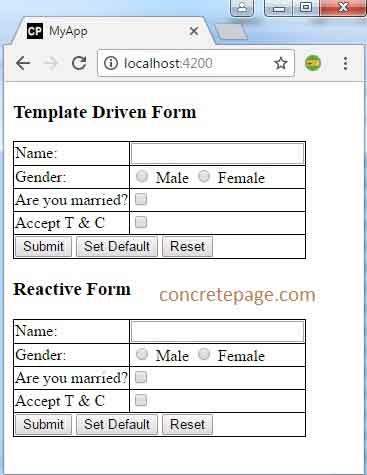
References
RadioControlValueAccessorCheckboxControlValueAccessor
Validators