JavaScript Array entries() Method
November 03, 2019
JavaScript Array entries()
method returns a new Array iterator object that contains the key/value pairs for each index in the array. Find the syntax.
array.entries()
entries()
method is supported by Chrome, Edge, Firefox, Opera, Safari etc. Internet Explorer does not support JavaScript Array entries()
method.
Using Array entries() Method
In our example, we have an array and on this array we are callingentries()
method that returns a new Array iterator object. By calling iterator next()
method, we get the key/value pairs for indexes. In key/value pair, the index is key and value is array element.
var array = ['Mahesh', 'Krishna', 'Brahma']; var iterator = array.entries(); var el1 = iterator.next(); console.log(el1.value); var el2 = iterator.next(); console.log(el2.value); var el3 = iterator.next(); console.log(el3.value);
[ 0, "Mahesh" ] [ 1, "Krishna" ] [ 2, "Brahma" ]
Iterating with for...of loop
The iterator returned byentries()
method can be iterated using JavaScript for...of loop.
var array = ['Mangalyaan', 'Chandrayaan', 'Gaganyaan', 'Aditya']; console.log('--- Fetching key/value pairs ---'); for (const el of array.entries()) { console.log(el); } console.log('--- Fetching index and element ---'); for (const [index, element] of array.entries()) { console.log('Index: ' + index + ', Element: ' + element); }
--- Fetching key/value pairs --- [ 0, "Mangalyaan" ] [ 1, "Chandrayaan" ] [ 2, "Gaganyaan" ] [ 3, "Aditya" ] --- Fetching index and element --- Index: 0, Element: Mangalyaan Index: 1, Element: Chandrayaan Index: 2, Element: Gaganyaan Index: 3, Element: Aditya
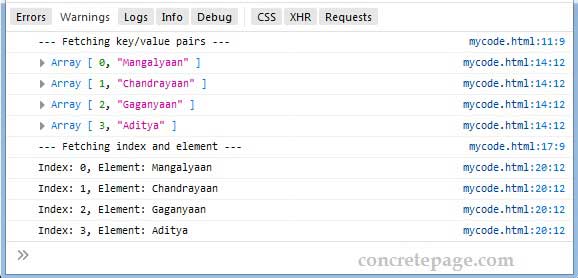
Two-Dimensional Array
Find the example to useentries()
method with two-dimensional array.
var array = [ ['a1', 'b1', 'c1'], ['a2', 'b2', 'c2'], ['a3', 'b3', 'c3'] ]; for (const [index, element] of array.entries()) { for (const el of element.entries()) { console.log(el); } }
[ 0, "a1" ] [ 1, "b1" ] [ 2, "c1" ] [ 0, "a2" ] [ 1, "b2" ] [ 2, "c2" ] [ 0, "a3" ] [ 1, "b3" ] [ 2, "c3" ]