Create Dynamic Radio Button in Angular
December 28, 2023
On this page, I will create an Angular application that will generate radio buttons dynamically. We need to create an object that will contain radio button informations for its value and title. Then iterate this object in HTML template to create radio buttons.
TS code:
paymentMethodData = [ {value: "cash", title: "Cash"}, {value: "card", title: "Card"}, {value: "netbanking", title: "Net Banking"}, {value: "upi", title: "UPI"}, {value: "emi", title: "EMI"} ]; ngOnInit() { this.registrationForm = this.formBuilder.group({ payMethod: ['', Validators.required], ------ }); }
<div *ngFor="let paymentMethod of paymentMethodData"> <input type="radio" [value]="paymentMethod.value" formControlName="payMethod" (change)="onPaymentMethodChange()"> {{paymentMethod.title}} </div>
Complete Example
In my demo application, I have a student registration form that will contain three radio button groups i.e. select gender, select information source about school and select fee payment method. I will create these radio buttons dynamically using seed data. Im using reactive form to create our student registration form.Now find the code.
Find the file that will contain radio button information data.
regdata.ts
export const STD_GENDER = [ { value: "male", title: "Male" }, { value: "female", title: "Female" } ]; export const INFO_SOURCE_SCHOOL = [ { value: "newspaper", title: "Newspaper" }, { value: "tv", title: "T.V" }, { value: "website", title: "Website" }, { value: "socialmedia", title: "Social Media" }, { value: "friend", title: "Friend" }, { value: "other", title: "Other" } ]; export const PAYMENT_METHOD = [ { value: "cash", title: "Cash" }, { value: "card", title: "Card" }, { value: "netbanking", title: "Net Banking" }, { value: "upi", title: "UPI" }, { value: "emi", title: "EMI" } ];
school-registration.component.ts
import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, ReactiveFormsModule, Validators } from '@angular/forms'; import { StudentRegistrationService } from './services/reg-service'; import { CommonModule } from '@angular/common'; import { INFO_SOURCE_SCHOOL, PAYMENT_METHOD, STD_GENDER } from './constants/regdata'; @Component({ selector: 'app-reactive', standalone: true, imports: [ReactiveFormsModule, CommonModule], templateUrl: './school-registration.component.html', styleUrls: ['./style.css'] }) export class SchoolRegistrationComponent implements OnInit { isFormValid!: boolean; stdGenData = STD_GENDER; infoSourceData = INFO_SOURCE_SCHOOL; paymentMethodData = PAYMENT_METHOD; registrationForm = {} as FormGroup; constructor(private formBuilder: FormBuilder, private regService: StudentRegistrationService) { } ngOnInit() { this.registrationForm = this.formBuilder.group({ stdName: ['', [Validators.required]], stdGender: ['', [Validators.required]], infoSource: ['', [Validators.required]], payMethod: ['', Validators.required] }); } onFormSubmit() { this.isFormValid = false; if (this.registrationForm.invalid) { return; } this.isFormValid = true; this.regService.saveRegistrationForm(this.registrationForm.value); this.reset(); } reset() { this.registrationForm.reset({ stdGender: "", infoSource: "", payMethod: "" }); } get stdGender() { return this.registrationForm.get('stdGender'); } get infoSource() { return this.registrationForm.get('infoSource'); } get payMethod() { return this.registrationForm.get('payMethod'); } setDefaultValues() { this.registrationForm.patchValue({ stdGender: 'female', infoSource: 'friend', payMethod: "card" }); } onStdGenderChange() { console.log(this.stdGender?.value); } onInfoSourceChange() { console.log(this.infoSource?.value); } onPaymentMethodChange() { console.log(this.payMethod?.value); } }
school-registration.component.html
<h3>Registration Form</h3> <p *ngIf="isFormValid && registrationForm.pristine" ngClass="success"> Student registered successfully. </p> <form [formGroup]="registrationForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td>Student Name:</td> <td> <input formControlName="stdName"> <div *ngIf="registrationForm.get('stdName')?.invalid && isFormValid != null && !isFormValid" ngClass="error"> Name required. </div> </td> </tr> <tr> <td>Gender:</td> <td> <div *ngFor="let gen of stdGenData"> <input type="radio" [value]="gen.value" formControlName="stdGender" (change)="onStdGenderChange()"> {{gen.title}} </div> <div *ngIf="stdGender?.invalid && isFormValid != null && !isFormValid" ngClass="error"> Select gender. </div> </td> </tr> <tr> <td>Source of information about school: </td> <td> <div *ngFor="let source of infoSourceData"> <input type="radio" [value]="source.value" formControlName="infoSource" (change)="onInfoSourceChange()"> {{source.title}} </div> <div *ngIf="infoSource?.invalid && isFormValid != null && !isFormValid" ngClass="error"> Select source. </div> </td> </tr> <tr> <td>How will you pay fee? </td> <td> <div *ngFor="let paymentMethod of paymentMethodData"> <input type="radio" [value]="paymentMethod.value" formControlName="payMethod" (change)="onPaymentMethodChange()"> {{paymentMethod.title}} </div> <div *ngIf="payMethod?.invalid && isFormValid != null && !isFormValid" ngClass="error"> Select payment method. </div> </td> </tr> <tr> <td colspan="2"> <button>Submit</button> <button type="button" (click)="setDefaultValues()">Set Default</button> <button type="button" (click)="reset()">Reset</button> </td> </tr> </table> </form>
export interface StudentRegistrationForm { stdName: string; stdGender: string; infoSource: string; payMethod: string; }
import { Injectable } from '@angular/core'; import { StudentRegistrationForm } from '../domain/registrationform'; @Injectable({ providedIn: 'root' }) export class StudentRegistrationService { saveRegistrationForm(regForm: StudentRegistrationForm) { console.log(regForm); } }
Output
Find the print-screen of the output.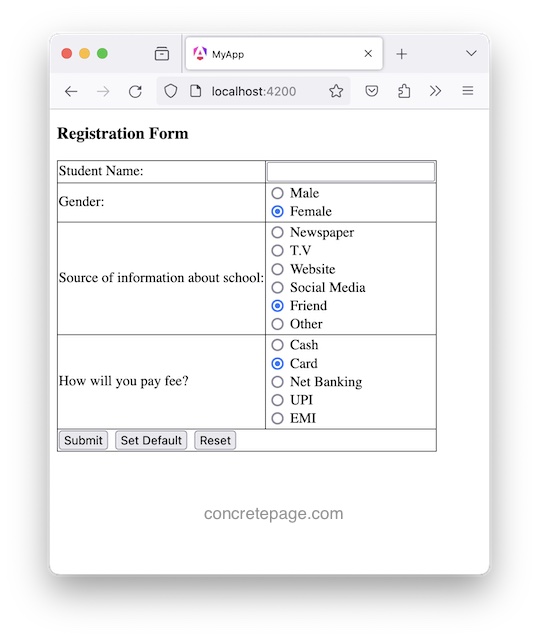