Angular Radio Button Selected By Default
December 26, 2023
In front-end application, the data display page or edit page needs to display radio button as selected with its existing values. In Angular application, the default value of radio button can be set easily. We can set default value using NgModel
, FormControl
and FormGroup
. Let us learn to use them one-by-one.
1. Using NgModel
We can set default value to a radio button group usingngModel
property binding. In HTML template, create a radio button group and use [ngModel]
property binding with a default value. Using property binding, we can also change selected radio button dynamically.
HTML code:
<input type="radio" value="service" name="compType" [ngModel]="comp.compType"> Service <input type="radio" value="product" name="compType" [ngModel]="comp.compType"> Product
comp = {} as Company; ngOnInit() { this.comp.compType = "service"; }
2. Using FormControl
To make radio button selected by defualt, we can useFormControl
. Instantiate FormControl
with a default value then bind it with radio button element.
HTML code:
<input type="radio" value="developer" [formControl]="myProfile"> Developer <input type="radio" value="manager" [formControl]="myProfile"> Manager
1. Assign default value using
FormControl
constructor.
myProfile= new FormControl("manager");
FormControl.setValue()
for default value.
myProfile= new FormControl(); ngOnInit() { this.myProfile.setValue("manager"); }
setValue
, we can change selected radio button dynamically.
3. Using FormGroup.patchValue()
FormGroup.patchValue()
patches the value of the FormGroup
. We can set default value on form loading as well as can set value dynamically for the selected field of the form.
TS code:
compForm = new FormGroup({ compSize: new FormControl('', Validators.required), ------ }); ngOnInit() { this.compForm.patchValue({compSize:"small"}); }
<form [formGroup]="compForm" (ngSubmit)="onFormSubmit()"> <div> <input type="radio" value="small" formControlName="compSize"> Small <input type="radio" value="big" formControlName="compSize"> Big </div> ------ </form>
4. Complete Example
Here I will create a demo Angular application that will contain form for a company detail. Radio buttons of the form will be selected by defualt for a specified value. In my application, I am using template-driven form as well as reactive form to create our company form.company.ts
export interface Company { compName: string; compType: string; compSize: string; }
<h3>Company Template-Driven Form</h3> <p *ngIf="isCompDataValid" ngClass="success"> Form submitted successfully. </p> <form #compForm="ngForm" (ngSubmit)="onFormSubmit(compForm)"> <table> <tr> <td>Name:</td> <td> <input name="compName" [ngModel]="comp.compName" required #cname="ngModel"> <div *ngIf="cname.invalid && compForm.submitted && !isCompDataValid" ngClass="error"> Name required. </div> </td> </tr> <tr> <td>Company Type:</td> <td> <input type="radio" value="service" name="compType" [ngModel]="comp.compType" required #ctype="ngModel"> Service <input type="radio" value="product" name="compType" [ngModel]="comp.compType" required #ctype="ngModel"> Product <div *ngIf="ctype.invalid && compForm.submitted && !isCompDataValid" ngClass="error"> Select type. </div> </td> </tr> <tr> <td>Company Size:</td> <td> <input type="radio" value="small" name="compSize" [ngModel]="comp.compSize" required #csize="ngModel"> Small <input type="radio" value="big" name="compSize" [ngModel]="comp.compSize" required #csize="ngModel"> Big <div *ngIf="csize.invalid && compForm.submitted && !isCompDataValid" ngClass="error"> Select size. </div> </td> </tr> <tr> <td colspan="2"> <button>Submit</button> <button type="button" (click)="setDefaultValues()">Set Default</button> <button type="button" (click)="resetForm(compForm)">Reset</button> </td> </tr> </table> </form>
import { Component, OnInit } from '@angular/core'; import { FormsModule, NgForm } from '@angular/forms'; import { CompanyService } from './services/comp-service'; import { Company } from './domain/company'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-template', standalone: true, imports: [FormsModule, CommonModule], templateUrl: './comp-template-driven.component.html', styleUrls: ['./style.css'] }) export class CompTemplateDrivenComponent implements OnInit { isCompDataValid = false; comp = {} as Company; constructor(private compService: CompanyService) { } ngOnInit() { this.setDefaultValues(); } onFormSubmit(form: NgForm) { this.isCompDataValid = false; if (form.invalid) { return; } this.isCompDataValid = true; this.comp.compName = form.controls['compName'].value; this.comp.compType = form.controls['compType'].value; this.comp.compSize = form.controls['compSize'].value; this.compService.createCompany(this.comp); this.resetForm(form); } resetForm(form: NgForm) { this.comp = {} as Company; form.resetForm({ compType: "", compSize: "" }); } setDefaultValues() { this.comp= {compName: 'PQR Ltd', compType:'product', compSize:"small"}; } }
<h3>Company Reactive Form</h3> <p *ngIf="isCompDataValid && compForm.pristine" ngClass="success"> Form submitted successfully. </p> <form [formGroup]="compForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td>Name:</td> <td> <input formControlName="compName"> <div *ngIf="compForm.get('compName')?.invalid && isCompDataValid != null && !isCompDataValid" ngClass="error"> Name required. </div> </td> </tr> <tr> <td>Company Type:</td> <td> <input type="radio" value="service" formControlName="compType"> Service <input type="radio" value="product" formControlName="compType"> Product <div *ngIf="compType?.invalid && isCompDataValid != null && !isCompDataValid" ngClass="error"> Select type. </div> </td> </tr> <tr> <td>Company Size: </td> <td> <input type="radio" value="small" formControlName="compSize"> Small <input type="radio" value="big" formControlName="compSize"> Big <div *ngIf="compSize?.invalid && isCompDataValid != null && !isCompDataValid" ngClass="error"> Select size. </div> </td> </tr> <tr> <td colspan="2"> <button>Submit</button> <button type="button" (click)="setDefaultValues()">Set Default</button> <button type="button" (click)="reset()">Reset</button> </td> </tr> </table> </form>
import { Component, OnInit } from '@angular/core'; import { FormControl, FormGroup, ReactiveFormsModule, Validators } from '@angular/forms'; import { CompanyService } from './services/comp-service'; import { Company } from './domain/company'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-reactive', standalone: true, imports: [ReactiveFormsModule, CommonModule], templateUrl: './company-reactive.component.html', styleUrls: ['./style.css'] }) export class EmpReactiveComponent implements OnInit { isCompDataValid!: boolean; compForm = new FormGroup({ compName: new FormControl('', Validators.required), compType: new FormControl('', Validators.required), compSize: new FormControl('', Validators.required) }); company!: Company; constructor(private compService: CompanyService) { } ngOnInit() { this.setDefaultValues(); } onFormSubmit() { this.isCompDataValid = false; if (this.compForm.invalid) { return; } this.isCompDataValid = true; this.company = { compName: this.compForm.get('compName')?.value ?? "", compType: this.compForm.get('compType')?.value ?? "", compSize: this.compForm.get('compSize')?.value ?? "" } this.compService.createCompany(this.company); this.reset(); } reset() { this.compForm.reset({ compType: "", compSize: "" }); } get compType() { return this.compForm.get('compType'); } get compSize() { return this.compForm.get('compSize'); } setDefaultValues() { this.compForm.setValue({ compName: 'PQR Ltd', compType: 'product', compSize: "small" }); } }
import { Injectable } from '@angular/core'; import { Company } from '../domain/company'; @Injectable({ providedIn: 'root' }) export class CompanyService { createCompany(comp: Company) { console.log("Name: " + comp.compName); console.log("Type: " + comp.compType); console.log("Size: " + comp.compSize); } }
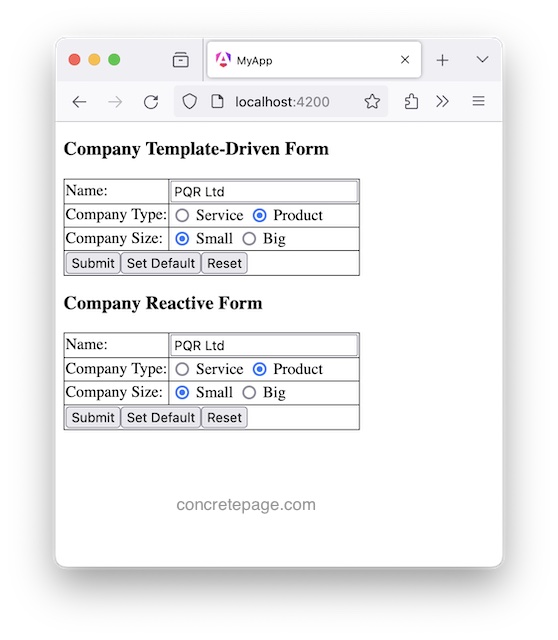