Angular Radio Button in Reactive Form
December 27, 2023
To create a radio button in reactive form, create a FormControl
in FormGroup
and bind it to radio button element using formControlName
directive.
TS code:
this.regForm = this.formBuilder.group({ payMethod: ['', Validators.required], ------ });
<div> <input type="radio" value="cash" formControlName="payMethod"> Cash <input type="radio" value="card" formControlName="payMethod"> Card <input type="radio" value="upi" formControlName="payMethod"> UPI </div>
On this page, I will create a reactive form for student registration that will contain radio button groups. In our demo application, I will get, set the value of radio button as well as submit the form to save data.
1. Create Component
In my demo application, I am creating student registration application that will have a form to register a student in the school.Our registration form will contain following fields.
regform.ts
export interface RegistrationForm { stdName: string; stdGender: string; infoSource: string; payMethod: string; }
school-registration.component.ts
import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, ReactiveFormsModule, Validators } from '@angular/forms'; import { RegistrationService } from './services/reg-service'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-reactive', standalone: true, imports: [ReactiveFormsModule, CommonModule], templateUrl: './school-registration.component.html', styleUrls: ['./style.css'] }) export class SchoolRegistrationComponent implements OnInit { isRegFormValid!: boolean; regForm = {} as FormGroup; constructor(private formBuilder: FormBuilder, private regService: RegistrationService) { } ngOnInit() { this.regForm = this.formBuilder.group({ stdName: ['', [Validators.required]], stdGender: ['', [Validators.required]], infoSource: ['', [Validators.required]], payMethod: ['', Validators.required] }); } onFormSubmit() { this.isRegFormValid = false; if (this.regForm.invalid) { return; } this.isRegFormValid = true; this.regService.saveRegForm(this.regForm.value); this.reset(); } reset() { this.regForm.reset({ stdGender: "", infoSource: "", payMethod: "" }); } get stdGender() { return this.regForm.get('stdGender'); } get infoSource() { return this.regForm.get('infoSource'); } get payMethod() { return this.regForm.get('payMethod'); } setDefaultValues() { this.regForm.patchValue({ stdGender: 'male', infoSource: 'website', payMethod: "upi" }); } onChangeStdGender() { console.log(this.stdGender); } onChangeInfoSource() { console.log(this.infoSource); } onChangePaymentMethod() { console.log(this.payMethod); } }
regForm
is a FormGroup
created by FormBuilder.group()
method.
2. All the fields of registration form are being
required
validated.
3. I have created
onChangeStdGender()
, onChangeInfoSource()
and onChangePaymentMethod()
methods to handle change
event of radio buttons used in our student registration form.
4. We can set default values to the
FormGroup
using its patchValue()
or setValue()
methods.
2. Create HTML Template
Find the HTML template used in our student registration application.school-registration.component.html
<h3>Student Registration Form</h3> <p *ngIf="isRegFormValid && regForm.pristine" ngClass="success"> Form submitted successfully. </p> <form [formGroup]="regForm" (ngSubmit)="onFormSubmit()"> <table> <tr> <td>Student Name:</td> <td> <input formControlName="stdName"> <div *ngIf="regForm.get('stdName')?.invalid && isRegFormValid != null && !isRegFormValid" ngClass="error"> Name required. </div> </td> </tr> <tr> <td>Student Gender:</td> <td> <input type="radio" value="male" formControlName="stdGender" (change)="onChangeStdGender()"> Male <input type="radio" value="female" formControlName="stdGender" (change)="onChangeStdGender()"> Female <div *ngIf="stdGender?.invalid && isRegFormValid != null && !isRegFormValid" ngClass="error"> Select gender. </div> </td> </tr> <tr> <td>How did you hear about school? </td> <td> <input type="radio" value="newspaper" formControlName="infoSource" (change)="onChangeInfoSource()"> Newspaper <input type="radio" value="tv" formControlName="infoSource" (change)="onChangeInfoSource()"> T.V. <input type="radio" value="website" formControlName="infoSource" (change)="onChangeInfoSource()"> Website <input type="radio" value="friend" formControlName="infoSource" (change)="onChangeInfoSource()"> Friend <div *ngIf="infoSource?.invalid && isRegFormValid != null && !isRegFormValid" ngClass="error"> Select information source. </div> </td> </tr> <tr> <td>Fee payment method: </td> <td> <input type="radio" value="cash" formControlName="payMethod" (change)="onChangePaymentMethod()"> Cash <input type="radio" value="card" formControlName="payMethod" (change)="onChangePaymentMethod()"> Card <input type="radio" value="upi" formControlName="payMethod" (change)="onChangePaymentMethod()"> UPI <div *ngIf="payMethod?.invalid && isRegFormValid != null && !isRegFormValid" ngClass="error"> Select payment method. </div> </td> </tr> <tr> <td colspan="2"> <button>Submit</button> <button type="button" (click)="setDefaultValues()">Set Default</button> <button type="button" (click)="reset()">Reset</button> </td> </tr> </table> </form>
formControlName
syncs a FormControl
to a form control element by name.
2. I have created three radio button groups, one to select gender, second to select information source and third to select payment method.
3. Use 'Set Default' button to make radio button selected with default values.
4. If we submit the form without selecting the radio buttons, validation error will be displayed.
Now find the service used in our student registration demo application.
reg-service.ts
import { Injectable } from '@angular/core'; import { RegistrationForm } from '../domain/regform'; @Injectable({ providedIn: 'root' }) export class RegistrationService { saveRegForm(regForm: RegistrationForm) { console.log(regForm); } }
Output
Find the print-screen of the output.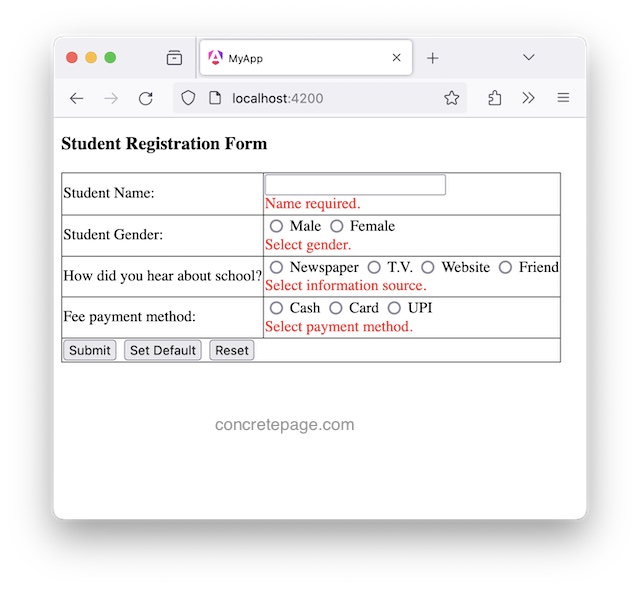