Angular markAsDirty()
September 06, 2023
On this page we will learn to use markAsDirty()
method in our Angular application.
1. The
markAsDirty()
is the method of Angular AbstractControl
class that marks a control as dirty.
2. A control is considered as dirty when we change value through UI. Suppose we enter a text in text box, then this control status is dirty. Now if we delete that content from text box, still the status of this control is dirty. A control is considered to be touched when focus and blur events occur that do not change the value.
3. We can programmatically mark a control dirty using
markAsDirty()
method of AbstractControl
. When we call markAsDirty()
on a control, the value of dirty becomes true.
On this page I will create a form validation application and use
markAsDirty()
method to mark controls dirty programatically.
Using markAsDirty()
ThemarkAsDirty()
is the method of AbstractControl
class that marks the control as dirty.
markAsDirty(opts: { onlySelf?: boolean; } = {}): void
Suppose we have created a form as following.
compForm: FormGroup = this.formBuilder.group({ compName: ['', Validators.minLength(3)], empNo: ['', Validators.minLength(2)], locations: this.formBuilder.array([ new FormControl('', [Validators.minLength(3)]), new FormControl('', [Validators.minLength(3)]) ]) });
markAsDirty()
on the form controls.
1. For
FormControl
:
this.compForm.get('compName')?.markAsDirty(); this.compForm.get('empNo')?.markAsDirty();
FormArray
:
this.compForm.get('locations')?.markAsDirty();
FormGroup
:
this.compForm.markAsDirty();
Find the code to use onlySelf with
markAsDirty()
method.
this.compForm.get('compName')?.markAsDirty({onlySelf: true});
true : marks dirty only self.
false : marks dirty all direct ancestors. This is default value.
Complete Example
In this example, I will create a reactive form with some controls with minLength validator. To show the usability ofmarkAsDirty()
, I am creating a scenario that once the form is dirty, user has to save the form before going to next page. Find the code.
company.component.ts
import { Component } from '@angular/core'; import { FormGroup, FormArray, Validators, FormBuilder, ReactiveFormsModule, FormControl } from '@angular/forms'; import { CompanyService } from './company.service'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-comp', standalone: true, imports: [ CommonModule, ReactiveFormsModule ], templateUrl: './company.component.html' }) export class CompanyComponent { formSubmitted = false; constructor( private formBuilder: FormBuilder, private compService: CompanyService) { } compForm: FormGroup = this.formBuilder.group({ compName: ['', Validators.minLength(3)], empNo: ['', Validators.minLength(2)], locations: this.formBuilder.array([ new FormControl('', [Validators.minLength(3)]), new FormControl('', [Validators.minLength(3)]) ]) }); get locations(): FormArray { return this.compForm.get('locations') as FormArray; } onFormSubmit() { this.compService.saveComp(this.compForm.value); this.formSubmitted = true; this.compForm.reset(); } markDirty() { this.compForm.get('compName')?.markAsDirty(); this.compForm.get('empNo')?.markAsDirty(); this.compForm.get('locations')?.markAsDirty(); //this.compForm.markAsDirty(); } }
<h3>markAsDirty() Example</h3> <div *ngIf="formSubmitted && compForm.pristine" class="submitted"> Form submitted successfully. </div> <div class="team"> <form [formGroup]="compForm" (ngSubmit)="onFormSubmit()"> <p>Company Name* : <br/><input formControlName="compName"> <br /><label *ngIf="compForm.get('compName')?.hasError('minlength')" class="error"> Minimum length 3. </label> </p> <p>No. Of Employee* : <br/><input formControlName="empNo"> <br /><label *ngIf="compForm.get('empNo')?.hasError('minlength')" class="error"> Minimum length 2. </label> </p> Locations* : <br/> <div formArrayName="locations"> <div *ngFor="let emp of locations.controls; let idx = index"> <input [formControlName]="idx"> <br/><br/> </div> <label *ngIf="compForm.get('locations')?.invalid" class="error"> Minimum length 3. </label> </div> <button [disabled]="!compForm.dirty">SAVE</button> <button type="button" (click)="compForm.reset()">NEXT</button> <button type="button" (click)="markDirty()">MARK AS DIRTY</button> </form> </div> <br/><b>Form Touched</b> : {{compForm.touched}} <br/><b>Form Dirty</b> : {{compForm.dirty}} <br/><br/><b>Dirty controls: </b> <br/><b>compName</b> : {{compForm.get('compName')?.dirty}} <br/><b>empNo</b> : {{compForm.get('empNo')?.dirty}} <br/><b>locations</b> : {{compForm.get('locations')?.dirty}}
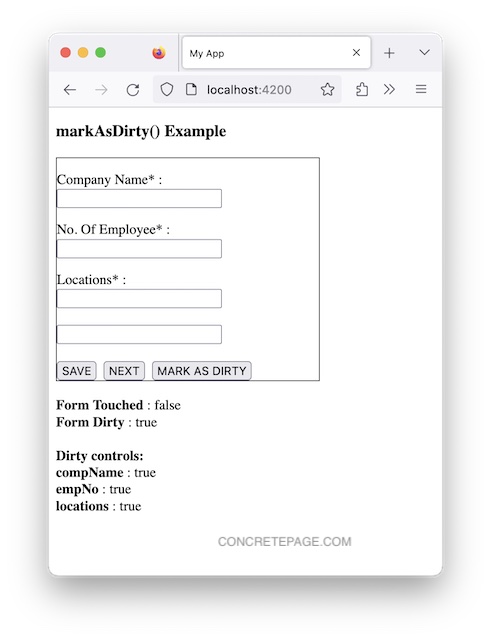