Angular markAllAsTouched()
September 05, 2023
On this page, we will learn to use markAllAsTouched()
method in our Angular application.
1. The
markAllAsTouched()
is the method of Angular AbstractControl
class. The markAllAsTouched()
method marks the control and all its descendant controls as touched.
2. We call a control as touched when focus and blur events occur that do not change the value. We can programmatically mark a control touched using
markAsTouched()
and markAllAsTouched()
methods of AbstractControl
.
a. markAsTouched() : Marks a control as touched.
b. markAllAsTouched() : Marks the control and all its descendant controls as touched.
3. The touched is a boolean property of
AbstractControl
that has true value if the control is touched by focus and blur events.
On this page we will create a form validation application using
markAllAsTouched()
method.
Using markAllAsTouched()
ThemarkAllAsTouched()
is the method of AbstractControl
class that marks the control and all its descendant controls as touched.
markAllAsTouched(): void
compForm: FormGroup = this.formBuilder.group({ ------ });
markAllAsTouched()
on the FormGroup
and it will mark all controls of the form as touched.
this.compForm.markAllAsTouched();
Complete Example
Find our example that has a form with some controls. The controls are required validated. When the user submits the form without entering data, we callmarkAllAsTouched()
method on the FormGroup
to make the form controls as touched so that error messages are displayed.
company.component.ts
import { Component } from '@angular/core'; import { FormGroup, FormArray, Validators, FormBuilder, ReactiveFormsModule, FormControl } from '@angular/forms'; import { CompanyService } from './company.service'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-comp', standalone: true, imports: [ CommonModule, ReactiveFormsModule ], templateUrl: './company.component.html' }) export class CompanyComponent { formSubmitted = false; constructor( private formBuilder: FormBuilder, private compService: CompanyService) { } compForm: FormGroup = this.formBuilder.group({ compName: ['', Validators.required], ceo: ['', Validators.required], locations: this.formBuilder.array([ new FormControl('', [Validators.required]), new FormControl('', [Validators.required]), ]) }); get locations(): FormArray { return this.compForm.get('locations') as FormArray; } onFormSubmit() { if (this.compForm.valid) { this.compService.saveComp(this.compForm.value); this.formSubmitted = true; this.compForm.reset(); } else { this.compForm.markAllAsTouched(); this.formSubmitted = false; } } }
<h3>markAllAsTouched() Example</h3> <div *ngIf="formSubmitted && compForm.pristine" class="submitted"> Form submitted successfully. </div> <div class="team"> <form [formGroup]="compForm" (ngSubmit)="onFormSubmit()"> <p>Company Name* : <br/><input formControlName="compName"> <br /><label *ngIf="compForm.get('compName')?.touched && compForm.get('compName')?.hasError('required')" class="error"> Company name required. </label> </p> <p>CEO* : <br/><input formControlName="ceo"> <br /><label *ngIf="compForm.get('ceo')?.touched && compForm.get('ceo')?.hasError('required')" class="error"> CEO required. </label> </p> Locations* : <br/> <div formArrayName="locations"> <div *ngFor="let emp of locations.controls; let idx = index"> <input [formControlName]="idx"> <br/><br/> </div> <label *ngIf="compForm.get('locations')?.touched && compForm.get('locations')?.invalid" class="error"> Locations required. </label> </div> <button>SUBMIT</button> </form> </div>
import { Injectable } from '@angular/core'; @Injectable() export class CompanyService { saveComp(data: any) { const comp = JSON.stringify(data); console.log(comp); } }
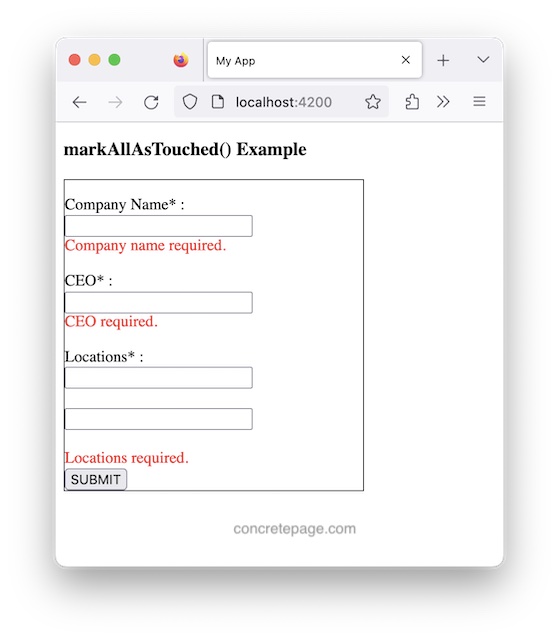