Hide and Show Password with Eye-Icon in Angular Material
April 23, 2024
On this page, I will create password input element to hide and show password with eye-icon in Angular Material application.
1. Eye-Icon
To create eye-icon, use keyword visibility and visibility_off.When password is visible, use visibility icon.
<mat-icon>visibility</mat-icon>
<mat-icon>visibility_off</mat-icon>
TS code:
pwdHide = true;
<mat-icon>{{pwdHide ? 'visibility_off' : 'visibility'}}</mat-icon>
2. Input Text
For password, input type is password and text has no visibility. To show password, we need to change input type as text. We need to change input type dynamically from password to text and vice-versa.TS code:
pwdHide = true;
<input matInput [type]="pwdHide ? 'password' : 'text'">
3. Using matPrefix and matSuffix
Within<mat-form-field>
, we can include icon button before or after input tag with the help of matPrefix
and matSuffix
.
a. Using matPrefix :
Adding
matPrefix
directive to an element, will make that element as prefix in that <mat-form-field>
.
Find the code to use
matPrefix
with mat-icon-button
.
<mat-form-field> <button mat-icon-button matPrefix (click)="pwdHide = !pwdHide"> <mat-icon >{{pwdHide ? 'visibility_off' : 'visibility'}}</mat-icon> </button> ------ </mat-form-field>
<mat-form-field>
.
b. Using matSuffix :
Adding
matSuffix
directive to an element, will make that element as suffix in that <mat-form-field>
.
Find the code snippet.
<mat-form-field> <button mat-icon-button matSuffix (click)="pwdHide = !pwdHide"> ------ </button> ------ </mat-form-field>
4. Example
Find the complete example to hide and show password with eye-icon in our Angular Material application.user.component.html
<h3>Eye-icon as Suffix</h3> <mat-form-field> <mat-label>Enter password</mat-label> <button mat-icon-button matSuffix (click)="visibilityToggle1()"> <mat-icon>{{getVisibility1()}}</mat-icon> </button> <input matInput [type]="getIputType1()"> </mat-form-field> <h3>Eye-icon as Prefix</h3> <mat-form-field> <mat-label>Enter password</mat-label> <button mat-icon-button matPrefix (click)="visibilityToggle2()"> <mat-icon>{{getVisibility2()}}</mat-icon> </button> <input matInput [type]="getIputType2()"> </mat-form-field>
import { Component } from '@angular/core'; import { MatInputModule } from '@angular/material/input'; import { MatFormFieldModule } from '@angular/material/form-field'; import { MatIconModule } from '@angular/material/icon'; import { MatButtonModule } from '@angular/material/button'; @Component({ selector: 'app-user', standalone: true, imports: [MatFormFieldModule, MatInputModule, MatButtonModule, MatIconModule], templateUrl: './user.component.html' }) export class UserComponent { pwdHide1 = true; pwdHide2 = true; visibilityToggle1() { this.pwdHide1 = !this.pwdHide1; } getIputType1() { return this.pwdHide1 ? 'password' : 'text'; } getVisibility1() { return this.pwdHide1 ? 'visibility_off' : 'visibility'; } visibilityToggle2() { this.pwdHide2 = !this.pwdHide2; } getIputType2() { return this.pwdHide2 ? 'password' : 'text'; } getVisibility2() { return this.pwdHide2 ? 'visibility_off' : 'visibility'; } }
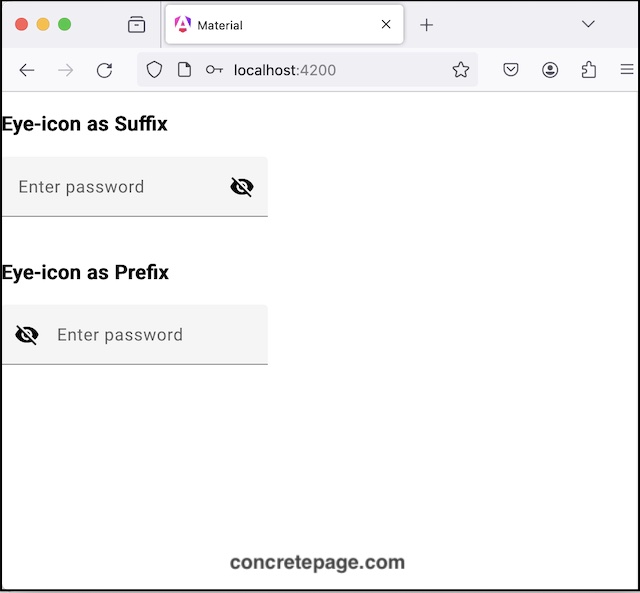