Angular Material Input Disabled
April 21, 2024
Input text in Angular Material is created as <input matInput>
. We can disable input text in following ways.
1. Use
disabled
attribute with <input> element.
2. Pass
disabled:true
to FormControl
constructor.
3. Use
enable()
and disable()
methods of FormControl
.
disabled
Attribute
To disable an input text, use disabled
attribute. To dynamically enable and disable input text, create a component property with default true/false value. Use property binding to bind component property with disabled
attribute.
TS code:
nameDisabled = true;
<input matInput [disabled]="nameDisabled" [(ngModel)]="name">
With FormControl
FormControl
accepts FormControlState
object. It has value and disabled property. Use it as below.
TS code:
city = new FormControl({ value: "", disabled: true });
<input matInput [formControl]="city">
Using enable()
and disable()
of FormControl
To enable and disable input text, we can use enable()
and disable()
methods of FormControl
.
TS code:
userForm = this.formBuilder.group({ age: [{ value: "", disabled: true }] }); get age() { return this.userForm.get('age'); } ageEnable() { this.age?.enable(); } ageDisable() { this.age?.disable(); }
<input matInput formControlName="age"> <button (click)="ageEnable()">Enable</button> <button (click)="ageDisable()">Disable</button>
Complete Example to Disable Input Text
user.component.tsimport { Component } from '@angular/core'; import { MatInputModule } from '@angular/material/input'; import { MatFormFieldModule } from '@angular/material/form-field'; import { FormBuilder, FormControl, FormsModule, ReactiveFormsModule, Validators } from '@angular/forms'; import { CommonModule } from '@angular/common'; @Component({ selector: 'app-user', standalone: true, imports: [MatFormFieldModule, MatInputModule, FormsModule, ReactiveFormsModule, CommonModule], templateUrl: './user.component.html' }) export class UserComponent { name = ""; nameDisabled = true; nameEnable() { this.nameDisabled = false; } nameDisable() { this.nameDisabled = true; } city = new FormControl({ value: "", disabled: true }, [Validators.maxLength(20)]); cityEnable() { this.city?.enable(); } cityDisable() { this.city?.disable(); } constructor(private formBuilder: FormBuilder) { } userForm = this.formBuilder.group({ age: [{ value: "", disabled: true }, [Validators.max(60)]] }); get age() { return this.userForm.get('age'); } ageEnable() { this.age?.enable(); } ageDisable() { this.age?.disable(); } onFormSubmit() { } }
<h3>1. With 'disabled' Attribute</h3> <mat-form-field> <mat-label>Name: </mat-label> <input matInput [disabled]="nameDisabled" [(ngModel)]="name"> </mat-form-field> <button (click)="nameEnable()">Enable</button> <button (click)="nameDisable()">Disable</button> <h3>2. With FormControl</h3> <mat-form-field> <mat-label>City: </mat-label> <input matInput [formControl]="city"> </mat-form-field> <button (click)="cityEnable()">Enable</button> <button (click)="cityDisable()">Disable</button> <h3>3. With FormGroup</h3> <form [formGroup]="userForm" (ngSubmit)="onFormSubmit()"> <mat-form-field> <mat-label>Age: </mat-label> <input matInput formControlName="age"> </mat-form-field> <button (click)="ageEnable()">Enable</button> <button (click)="ageDisable()">Disable</button> </form>
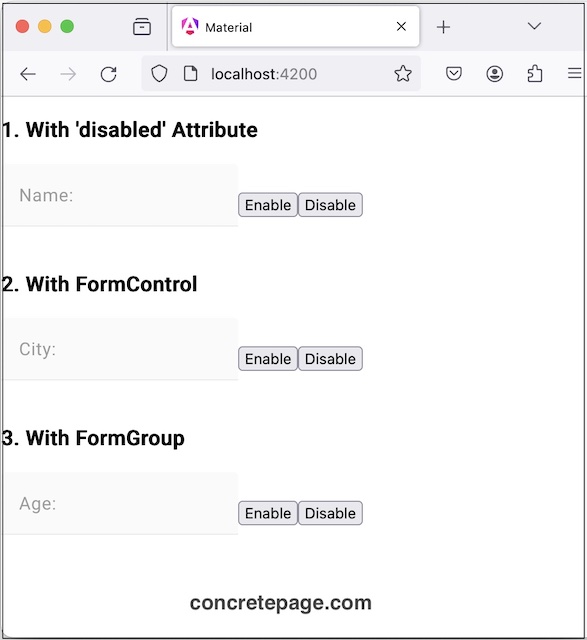