Angular Json Pipe Example
February 12, 2024
On this page we will provide Angular Json pipe example. Angular JsonPipe converts data into JSON string. It uses json
keyword with pipe operator to convert any expression result into JSON string. In our example we will create object and array of objects and then we will display them into JSON string using JsonPipe
. It is used as follows.
<pre> {{person | json}} </pre>
Where
person
is an object. JsonPipe
is working as a function that is taking arguments of given expression and json
keyword and returning JSON string. <pre> tag helps to get JSON string as pretty print. Now find the JsonPipe
example step by step using typescript.
Contents
Create Component and Classes used in Example
In our example we are using following classes whose object will be converted into JSON string.name.ts
export class Name { constructor(public fname:string, public lname:string) { } }
export class Address { constructor(public vill:string, public district:string, public country:string) { } }
import {Name} from './name'; import {Address} from './address'; export class Person { constructor(public id:number, public pname:Name, public dob:Date, public address:Address) { } }
import {Component} from '@angular/core'; import {Person} from './person'; import {Name} from './name'; import {Address} from './address'; @Component({ selector: 'app-root', template: ` <b>JSON for Address Class</b> <pre> {{address1 | json}} </pre> <b>JSON for Array of Address</b> <pre> <div [innerHTML]="addressArray | json"></div> </pre> <b>JSON for Person Name Property</b> <pre> {{person.pname | json}} </pre> <b>JSON for Person class</b> <pre>{{person | json}}</pre> ` }) export class JsonPipeComponent { address1 = new Address('Dhananjaypur', 'Varanasi', 'India'); address2 = new Address('Moonsi', 'Bhadohi', 'India'); addressArray = [this.address1, this.address2]; nameObj = new Name('Narendra', 'Modi'); dob = new Date(1950, 9, 17); person = new Person(100, this.nameObj, this.dob, this.address1); }
JsonPipe
JsonPipe
is an angular PIPE
. JsonPipe
converts an expression value into JSON string. JsonPipe
uses JSON.stringify
to convert into JSON string. In JSON.stringify
, JSON
is a subset of JavaScript. JsonPipe
uses json
keyword to convert value into JSON string using pipe operator as follows.
expression | json
For the example find the object of
Address
class in our component.
address1 = new Address('Dhananjaypur', 'Varanasi', 'India');
json
keyword with pipe operator (|) to convert the given object into JSON string.
<pre> {{address1 | json}} </pre>
{ "vill": "Dhananjaypur", "district": "Varanasi", "country": "India" }
JsonPipe with Array
Here we will create an array of objects ofAddress
class and then we will display it into JSON format using JsonPipe
. Find the array created in our component.
address1 = new Address('Dhananjaypur', 'Varanasi', 'India'); address2 = new Address('Moonsi', 'Bhadohi', 'India'); addressArray = [this.address1, this.address2];
json
keyword with pipe operator (|).
<pre> {{addressArray | json}} </pre>
JsonPipe
as given below.
<pre> <div [innerHTML]="addressArray | json"></div> >/pre>
[ { "vill": "Dhananjaypur", "district": "Varanasi", "country": "India" }, { "vill": "Moonsi", "district": "Bhadohi", "country": "India" } ]
JsonPipe with Object of Objects
In our example we have aPerson
class. This class will be initialized by passing objects of Name
and Address
class in our component.
address1 = new Address('Dhananjaypur', 'Varanasi', 'India'); nameObj = new Name('Narendra', 'Modi'); dob = new Date(1950, 9, 17); person = new Person(100, this.nameObj, this.dob, this.address1);
person
object's any property into JSON string using JsonPipe
, we can do as follows.
<pre> {{person.pname | json}} </pre>
{ "fname": "Narendra", "lname": "Modi" }
JsonPipe
with person
object.
<pre> {{person | json}} </pre>
{ "id": 100, "pname": { "fname": "Narendra", "lname": "Modi" }, "dob": "1950-10-16T18:30:00.000Z", "address": { "vill": "Dhananjaypur", "district": "Varanasi", "country": "India" } }
Create Module
app.module.tsimport {NgModule} from '@angular/core'; import {BrowserModule} from '@angular/platform-browser'; import {JsonPipeComponent} from './jsonpipe.component'; @NgModule({ imports: [BrowserModule], declarations: [JsonPipeComponent], bootstrap: [JsonPipeComponent] }) export class AppModule { }
Output
Find the print-screen of the output.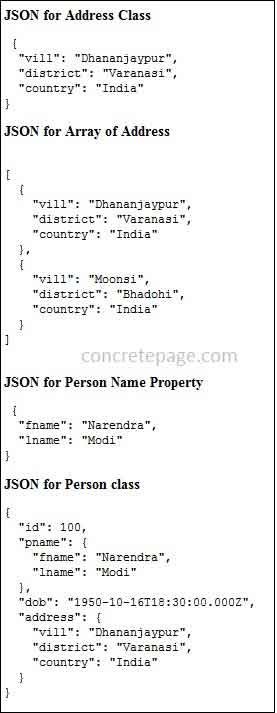