Android Bottom Navigation Bar : Selected Item Background Color
August 14, 2023
On this page we will learn to set selected item background color using XML as well as programatically.
1. Using <selector>
Find the selector XML to set selected item background color.res/navigation/navigation_bar_selector.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:drawable="@color/green" android:state_selected="true"/> <item android:drawable="@color/gray"/> </selector>
<item>
without state must be at last.
Now associate the above selector with
BottomNavigationView
using app:itemBackground
attribute.
res/layout/activity_main.xml
<com.google.android.material.bottomnavigation.BottomNavigationView --------- app:itemBackground="@navigation/navigation_bar_selector" />
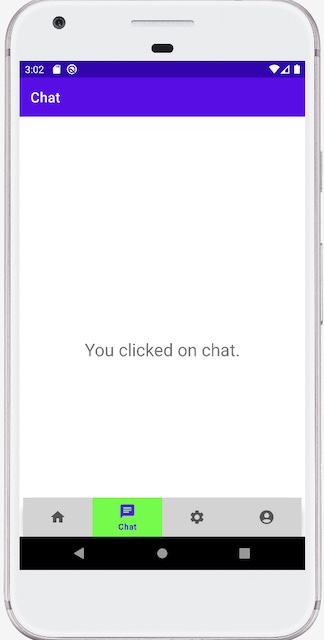
2. Using StateListDrawable
Find the code to set selected item background color programatically usingStateListDrawable
.
BottomNavigationView bottomNavView = findViewById(R.id.bottom_nav_view); StateListDrawable stDrawable = new StateListDrawable(); stDrawable.addState(new int[]{android.R.attr.state_selected}, new ColorDrawable(Color.GREEN)); stDrawable.addState(new int[]{}, new ColorDrawable(Color.GRAY)); bottomNavView.setItemBackground(stDrawable);
3. Using ColorStateList with ColorStateListDrawable
Find the code to set selected item background color programatically usingColorStateList
and ColorStateListDrawable
.
int[][] states = new int[][] { new int[] { android.R.attr.state_selected}, new int[] {} }; int[] colors = new int[] { getResources().getColor(R.color.green, getTheme()), getResources().getColor(R.color.gray, getTheme()) }; ColorStateList colorStateList = new ColorStateList(states, colors); ColorStateListDrawable drawable = new ColorStateListDrawable(colorStateList); bottomNavView.setItemBackground(drawable);
states
array is for first index value of colors
array. The element without state in states
array must be last element.
4. Using ColorStateList with MaterialShapeDrawable
Find the code to set selected item background color programatically usingColorStateList
with MaterialShapeDrawable
.
BottomNavigationView bottomNavView = findViewById(R.id.bottom_nav_view); MaterialShapeDrawable materialShapeDrawable = (MaterialShapeDrawable)bottomNavView.getBackground(); int[][] states = new int[][] { new int[] { android.R.attr.state_selected}, new int[] {} }; int[] colors = new int[] { Color.GREEN, Color.GRAY }; ColorStateList colorStateList = new ColorStateList(states, colors); materialShapeDrawable.setFillColor(colorStateList); bottomNavView.setItemBackground(materialShapeDrawable);
states
array must be last element.
Find the code to set the items' icons tint.
bottomNavView.setItemIconTintList(colorStateList);
MainActivity.java
package com.cp.app; import android.content.res.ColorStateList; import android.graphics.Color; import android.os.Bundle; import androidx.appcompat.app.AppCompatActivity; import androidx.navigation.NavController; import androidx.navigation.Navigation; import androidx.navigation.ui.AppBarConfiguration; import androidx.navigation.ui.NavigationUI; import com.cp.app.databinding.ActivityMainBinding; import com.google.android.material.bottomnavigation.BottomNavigationView; import com.google.android.material.shape.CornerFamily; import com.google.android.material.shape.MaterialShapeDrawable; import com.google.android.material.shape.ShapeAppearanceModel; public class MainActivity extends AppCompatActivity { private ActivityMainBinding binding; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); binding = ActivityMainBinding.inflate(getLayoutInflater()); setContentView(binding.getRoot()); // Creating ShapeAppearanceModel object BottomNavigationView bottomNavView = findViewById(R.id.bottom_nav_view); MaterialShapeDrawable materialShapeDrawable = (MaterialShapeDrawable)bottomNavView.getBackground(); //Setting rounded corner float cornerRadius = getResources().getFloat(R.dimen.corner_radius); ShapeAppearanceModel shapeAppearanceModel = materialShapeDrawable.getShapeAppearanceModel().toBuilder() .setAllCorners(CornerFamily.ROUNDED, cornerRadius).build(); materialShapeDrawable.setShapeAppearanceModel(shapeAppearanceModel); // Setting selected item background int[][] states = new int[][] { new int[] { android.R.attr.state_selected}, new int[] {} }; int[] colors = new int[] { Color.GREEN, Color.GRAY }; ColorStateList colorStateList = new ColorStateList(states, colors); materialShapeDrawable.setFillColor(colorStateList); bottomNavView.setItemBackground(materialShapeDrawable); // end AppBarConfiguration appBarConfiguration = new AppBarConfiguration.Builder( R.id.navigation_home, R.id.navigation_chat, R.id.navigation_settings, R.id.navigation_account) .build(); NavController navController = Navigation.findNavController(this, R.id.nav_host_fragment); NavigationUI.setupActionBarWithNavController(this, navController, appBarConfiguration); NavigationUI.setupWithNavController(binding.bottomNavView, navController); } }
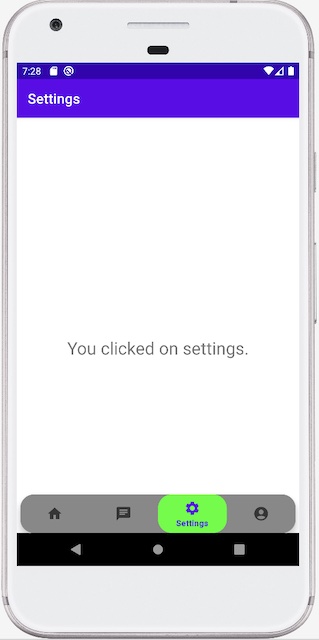