Custom Error Message in Spring Security
November 26, 2019
Spring Security facilitates custom error messages to handle failed authentication. In Spring Security XML configuration, using <http>
, we declare <form-login/>
to create custom login form. The <http>
tag has the attributes such as login-page
and default-target-url
. The login-page
attribute configures the login page URL and default-target-url
attribute configures page path for successful login. Find the below configuration for custom login page.
Custom Login Page in Spring Security
<http auto-config="true"> <intercept-url pattern="/login" access="ROLE_USER" /> <form-login login-page='/customLogin?login_error=1' default-target-url="/loginSuccess"/> </http>
<form name='form' action='j_spring_security_check' method='POST'> <table> <tr> <td>User Name:</td> <td><input type='text' name='j_username' value=''></td> </tr> <tr> <td>Password:</td> <td><input type='password' name='j_password'/></td> </tr> <tr> <td colspan='2'> <input name="submit" type="submit" value="Login"/></td> </tr> </table> </form>
j_spring_security_check
that sends the form to spring authentication. Username text name and password field will be j_username
and j_password
. In this way built-in spring authentication process will fetch the user input.
Custom Error Message in Spring Security
Now for the custom error message, we need to declare query string parameter aslogin_error=1
in <form-login/>
. It has been declared in our example as following.
<form-login login-page='/customLogin?login_error=1' default-target-url="/loginSuccess"/>
<c:if test="${not empty SPRING_SECURITY_LAST_EXCEPTION}"> <font color="red"> Your login attempt was not successful due to <br/><br/> <c:out value="${SPRING_SECURITY_LAST_EXCEPTION.message}"/>. </font> </c:if>
XML and Java files
security-config.xml<beans:beans xmlns="http://www.springframework.org/schema/security" xmlns:beans="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.1.xsd http://www.springframework.org/schema/security http://www.springframework.org/schema/security/spring-security-3.1.xsd"> <http auto-config="true"> <intercept-url pattern="/login" access="ROLE_USER" /> <form-login login-page='/customLogin?login_error=1' default-target-url="/loginSuccess"/> </http> <authentication-manager> <authentication-provider> <user-service> <user name="concretepage" password="con1234" authorities="ROLE_USER" /> </user-service> </authentication-provider> </authentication-manager> </beans:beans>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.1.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.1.xsd"> <context:component-scan base-package="com.concretepage.security.controller" /> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/pages/"/> <property name="suffix" value=".jsp"/> </bean> </beans>
<?xml version="1.0" encoding="ISO-8859-1" ?> <web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version="2.4"> <display-name>Spring Security Application</display-name> <servlet> <servlet-name>dispatcher</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>dispatcher</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> <context-param> <param-name>contextConfigLocation</param-name> <param-value> /WEB-INF/dispatcher-servlet.xml, /WEB-INF/security-config.xml </param-value> </context-param> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <!-- Spring Security Configuration --> <filter> <filter-name>springSecurityFilterChain</filter-name> <filter-class>org.springframework.web.filter.DelegatingFilterProxy</filter-class> </filter> <filter-mapping> <filter-name>springSecurityFilterChain</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
package com.concretepage.security.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.ModelMap; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; @Controller public class LoginController { @RequestMapping(value="/customLogin", method = RequestMethod.GET) public String customLogin(ModelMap map) { return "customLogin"; } @RequestMapping(value="/loginSuccess", method = RequestMethod.GET) public String success(ModelMap map) { map.addAttribute("msg", "Successfully logged in"); return "success"; } }
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <html> <head> <title>Spring Security Login page</title> </head> <body> <h2>Custom Login Form</h2> <c:if test="${not empty SPRING_SECURITY_LAST_EXCEPTION}"> <font color="red"> Your login attempt was not successful due to <br/><br/> <c:out value="${SPRING_SECURITY_LAST_EXCEPTION.message}"/>. </font> </c:if> <form name='form' action='j_spring_security_check' method='POST'> <table> <tr> <td>User Name:</td> <td><input type='text' name='j_username' value=''></td> </tr> <tr> <td>Password:</td> <td><input type='password' name='j_password'/></td> </tr> <tr> <td colspan='2'> <input name="submit" type="submit" value="Login"/></td> </tr> </table> </form> </body> </html>
<html> <body> ${msg} </body> </html>
<dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-core</artifactId> <version>3.1.4.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-web</artifactId> <version>3.1.4.RELEASE</version> </dependency> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-config</artifactId> <version>3.1.4.RELEASE</version> </dependency> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency>
Spring Security : eclipse configuration
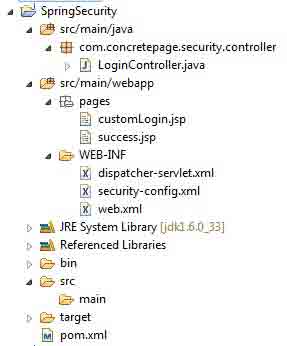
Output
Fetch our URL ashttp://localhost:8080/SpringSecurity/login
and we will get the below pages.
1. First Login Page
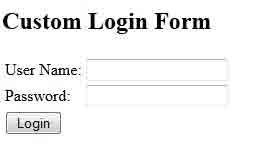
2. Login page after authentication failed.
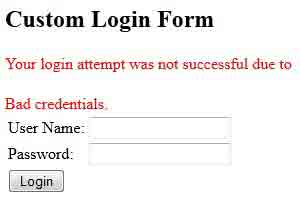