Hibernate 4 Annotation Example with Gradle
July 28, 2014
In this page we will learn Hibernate example using annotation. In hibernate creating SessionFactory is changed. StandardServiceRegistryBuilder is used to create SessionFactory. To resolve the JAR dependency , gardle has been used. I am providing complete example to run the demo. You can also find the download link at the bottom.
Software Used
In our Hibernate Example, we are using below software.1. JDK 7
2. Eclipse
3. Gradle 2.0
4. MySQL 5.5
Project Structure in Eclipse
Before start, check the class and cfg file location in eclipse.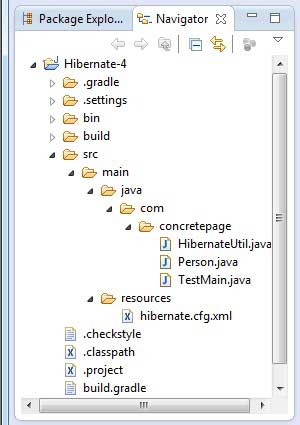
Create Database Table in MySQL
For the demo, I am using MySQL 5.5. I have created a database schema with the name "concretepage" and inside that schema I have created a table named as person.person
CREATE TABLE `person` ( `id` INT(10) NULL DEFAULT NULL, `name` VARCHAR(50) NULL DEFAULT NULL )
Hibernate JAR Dependency Using Gradle
To resolve JAR dependency of Hibernate 4, find the below Gradle script.build.gradle
apply plugin: 'java' apply plugin: 'eclipse' archivesBaseName = 'Concretepage' version = '1.0-SNAPSHOT' repositories { mavenCentral() } dependencies { compile 'org.hibernate:hibernate-core:4.3.6.Final' compile 'javax.servlet:javax.servlet-api:3.1.0' compile 'org.slf4j:slf4j-simple:1.7.7' compile 'org.javassist:javassist:3.15.0-GA' compile 'mysql:mysql-connector-java:5.1.31' }
Create Annotation based Entity for the Demo
In our example we are using a Person table and so find the person entity with annotation.Person.java
package com.concretepage; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name="person") public class Person { @Id @Column(name="id") private int id; @Column(name="name") private String name; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Create hibernate.cfg.xml
Now find the hibernate configuration file that will have database connection setting and entity registration.hibernate.cfg.xml
<!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url"> jdbc:mysql://localhost:3306/concretepage</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.connection.password"></property> <property name="hibernate.connection.pool_size">10</property> <property name="show_sql">true</property> <property name="dialect">org.hibernate.dialect.MySQLDialect</property> <property name="hibernate.hbm2ddl.auto">update</property> <mapping class="com.concretepage.Person"/> </session-factory> </hibernate-configuration>
Create SessionFactory using StandardServiceRegistryBuilder in Hibernate 4
This part is important regarding hibernate 4 feature. Now create SessionFactory using StandardServiceRegistryBuilder.HibernateUtil.java
package com.concretepage; import org.hibernate.SessionFactory; import org.hibernate.boot.registry.StandardServiceRegistryBuilder; import org.hibernate.cfg.Configuration; public class HibernateUtil { private static SessionFactory sessionFactory ; static { Configuration configuration = new Configuration().configure(); StandardServiceRegistryBuilder builder = new StandardServiceRegistryBuilder().applySettings(configuration.getProperties()); sessionFactory = configuration.buildSessionFactory(builder.build()); } public static SessionFactory getSessionFactory() { return sessionFactory; } }
Create Main Class to Test
Finally we are in the position to test our hibernate 4 example. In main method we are just saving a person entity in database.TestMain.java
package com.concretepage; import org.hibernate.Session; public class TestMain { public static void main(String[] args) { Person person = new Person(); person.setId(1); person.setName("Concretepage"); Session session = HibernateUtil.getSessionFactory().openSession(); session.beginTransaction(); session.save(person); session.getTransaction().commit(); session.close(); System.out.println("Done"); } }
Hibernate: insert into person (name, id) values (?, ?) Done