Android UserDictionary Content Provider Insert/Update/Delete/Fetch Example
July 29, 2015
On this page we will provide Android UserDictionary Content Provider with insert, update, delete and fetch operation example using ContentValues, ContentResolver and Uri. Content provider manages central repository for content. Here we will use UserDictionary content provider in our example. ContentValues holds the data to insert or update as key-value pair. While inserting content, android creates an immutable Uri using UserDictionary.Words.CONTENT_URI that can be used to update and delete inserted content later. We have to provide read permission to read/fetch the content and write permission for insert, update and delete operation in AndroidManifest.xml.
<uses-permission android:name="android.permission.READ_USER_DICTIONARY"/> <uses-permission android:name="android.permission.WRITE_USER_DICTIONARY"/>
Content Provider : UserDictionary
Content provider manages data with an id _ID which is assigned automatically to the content. Content provider facilitates easy insert, update, delete and fetch operation. Data can be used by external applications. Data is kept in table. Here we will discuss a content providerUserDictionary
. We can add words in dictionary. UserDictionary
class has an inner class UserDictionary.Words
which has constants as _ID, APP_ID, CONTENT_ITEM_TYPE, CONTENT_TYPE, DEFAULT_SORT_ORDER, FREQUENCY, LOCALE, SHORTCUT, WORD that are used as key to insert words.
ContentValues
android.content.ContentValues contains the values as key/value pair like HashMap. The methods ofContentValues
are put(String key, String value), getAsString(String key), containsKey(String key) etc. Find the code snippet.
ContentValues conValues = new ContentValues(); conValues.put(UserDictionary.Words.LOCALE, "hi-IN"); conValues.put(UserDictionary.Words.WORD, "Mahesh");
ContentResolver
android.content.ContentResolver helps applications to access contents. It is initialized by callingContentResolver resolver = getContentResolver();
Activity
class. ContentResolver
has different methods to manage content. Some methods are
insert(Uri url, ContentValues values) update(Uri uri, ContentValues values, String where, String[] selectionArgs) delete(Uri url, String where, String[] selectionArgs)
Uri
android.net.Uri is an immutable reference for the content row. While inserting content,Uri
is created and returned. It has different methods. Some of them are as follows.
getQueryParameter(String key) : Returns query parameter as String searching in query string for the first value of key.
normalizeScheme() : Returns equivalent Uri with lowercase scheme component.
fromFile(File file) : Returns Uri creating from File.
getPath() : Returns decoded path as string.
Complete Example
Find the complete example for insert, update, delete and fetch which are self-explanatory.ContentHelper.java
package com.concretepage; import android.content.ContentResolver; import android.content.ContentValues; import android.database.Cursor; import android.net.Uri; import android.provider.UserDictionary; public class ContentHelper { /** INSERT */ public static Uri insertContent(ContentResolver resolver) { ContentValues conValues = new ContentValues(); conValues.put(UserDictionary.Words.APP_ID, "com.concretepage"); conValues.put(UserDictionary.Words.LOCALE, "hi-IN"); conValues.put(UserDictionary.Words.WORD, "Mahesh"); conValues.put(UserDictionary.Words.FREQUENCY, "10"); Uri uri = resolver.insert(UserDictionary.Words.CONTENT_URI, conValues); return uri; } /** FETCH */ public static String fetchContent(ContentResolver resolver, Uri uri) { StringBuffer data = new StringBuffer(); String[] projection = { UserDictionary.Words._ID, UserDictionary.Words.LOCALE, UserDictionary.Words.WORD, UserDictionary.Words.FREQUENCY }; String sortOrder = UserDictionary.Words.LOCALE; Cursor cursor = resolver.query(uri, projection, null, null, sortOrder); while (cursor.moveToNext()) { data.append(cursor.getString(1) + "," + cursor.getString(2) + ", " + cursor.getString(3)); data.append("\n"); } return data.toString(); } /** UPDATE */ public static int updateContent(ContentResolver resolver, Uri uri) { ContentValues conValues = new ContentValues(); String selectionClause = UserDictionary.Words.WORD + " LIKE ?"; String[] selectionArgs = {"M%"}; conValues.put(UserDictionary.Words.WORD, "Krishna"); int rowsUpdated = resolver.update(uri, conValues, selectionClause, selectionArgs); return rowsUpdated; } /** DELETE */ public static int deleteContent(ContentResolver resolver) { String selectionClause = UserDictionary.Words.LOCALE + " LIKE ?"; String[] selectionArgs = {"hi-IN"}; int rowsDeleted = resolver.delete(UserDictionary.Words.CONTENT_URI, selectionClause, selectionArgs); return rowsDeleted; } }
MainActivity.java
package com.concretepage; import android.app.Activity; import android.content.ContentResolver; import android.net.Uri; import android.os.Bundle; import android.util.Log; import android.widget.TextView; public class MainActivity extends Activity { private static final String TAG = "MainActivity"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); } @Override protected void onStart() { super.onStart(); ContentResolver resolver = getContentResolver(); Uri uri = ContentHelper.insertContent(resolver); Log.d(TAG, "Uri:"+uri.getPath()); String data = ContentHelper.fetchContent(resolver, uri); TextView view = (TextView)findViewById(R.id.data); view.setText(data); int rows = ContentHelper.updateContent(resolver, uri); Log.d(TAG, "Rows Updated:"+ rows); data = ContentHelper.fetchContent(resolver, uri); TextView updateView = (TextView)findViewById(R.id.updatedata); updateView.setText(data); rows = ContentHelper.deleteContent(resolver); Log.d(TAG, "Rows Deleted:"+ rows); } }
main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#C98C00" tools:context=".MainActivity"> <TextView android:id="@+id/data" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="30sp"/> <TextView android:id="@+id/updatedata" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="30sp"/> </LinearLayout>
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.concretepage" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="11"/> <uses-permission android:name="android.permission.READ_USER_DICTIONARY"/> <uses-permission android:name="android.permission.WRITE_USER_DICTIONARY"/> <application android:allowBackup ="false" android:icon="@drawable/ic_launcher" android:label="@string/app_name" > <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Output
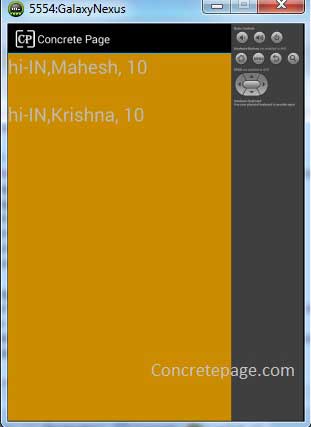
07-29 06:13:29.440: D/MainActivity(762): Uri:/words/25 07-29 06:13:29.530: D/MainActivity(762): Rows Updated:1 07-29 06:13:29.590: D/MainActivity(762): Rows Deleted:1