SpringBootServletInitializer Example
December 03, 2021
On this page we will learn using SpringBootServletInitializer
class.
1. The
SpringBootServletInitializer
class is the implementation of WebApplicationInitializer
interface.
2. The
SpringBootServletInitializer
is used to run SpringApplication
from a traditional WAR deployment.
3. The
SpringBootServletInitializer
binds Servlet
, Filter
and ServletContextInitializer
beans from the application context to the server.
4. By default we run Spring Boot application by creating an executable JAR with embedded server. To run WAR file of Spring Boot application from a standalone web server, we need to use
SpringBootServletInitializer
.
5. To use it, the
main
class annotated with @SpringBootApplication
extends SpringBootServletInitializer
.
@SpringBootApplication public class SpringBootDemo extends SpringBootServletInitializer { public static void main(String[] args) { SpringApplication.run(SpringBootDemo.class, args); } }
6. The class extending
SpringBootServletInitializer
should either override its configure
method or make the initializer class itself a @Configuration
class.
Find the
configure
method from Spring doc.
SpringApplicationBuilder configure(SpringApplicationBuilder builder)
SpringBootServletInitializer
in combination with other WebApplicationInitializers
, we might need to add an @Ordered
annotation to configure a specific startup order.
Using SpringBootServletInitializer
pom.xml<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.concretepage</groupId> <artifactId>soap-ws</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>war</packaging> <name>spring-demo</name> <description>Spring Demo Application</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.6.1</version> <relativePath /> </parent> <properties> <java.version>16</java.version> <context.path>spring-app</context.path> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <version>3.2.0</version> <configuration> <warName>${context.path}</warName> </configuration> </plugin> </plugins> </build> </project>
package com.concretepage; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class MyController { @GetMapping(value="hello") public String hello() { return "Hello World!"; } }
package com.concretepage; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; import org.springframework.boot.web.servlet.support.SpringBootServletInitializer; @SpringBootApplication public class SpringBootDemo extends SpringBootServletInitializer { public static void main(String[] args) { SpringApplication.run(SpringBootDemo.class, args); } }
@SpringBootApplication
annotation is the combination of @Configuration
, @EnableAutoConfiguration
and @ComponentScan
annotations.
Now run application from a traditional WAR deployment.
1. Create
WAR
using below command.
mvn clean package
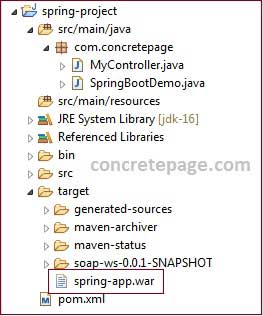
3. Access the URL.
http://localhost:8080/spring-app/hello