Spring Boot Interview Questions
November 23, 2023
1. What are the features of Spring Boot applications?
Ans: Spring Boot has following features.1. Performs lots of configurations automatically. Hence the development is easier and faster.
2. Includes support for embedded Tomcat, Jetty, and Undertow servers with default port 8080.
3. Auto configures the necessary infrastructure to send and receive messages using JMS.
4. Uses Commons Logging for all internal logging, but we can also implement our Logging. By default Logback is used.
5. Provides auto-configuration for Redis, MongoDB, Neo4j, Elasticsearch, Solr and Cassandra NoSQL technologies.
6. Externalize our configurations so that we will be able to work with the same application code in different environments. We can use .properties files, YAML files, environment variables and command-line arguments to externalize configuration.
7. Provides
@EnableAutoConfiguration
that allows Spring Boot to configure Spring application based on JAR dependencies that we have added.
8. Provides
@SpringBootApplication
annotation that is the combination of @Configuration
, @EnableAutoConfiguration
and @ComponentScan
annotations.
9. Uses
SpringApplication.run()
inside Java main method to bootstrap the application.
10. Provides a
@SpringBootTest
annotation to test application.
2. How to Create Spring Boot Project using Spring Initializer?
Ans: Go to the spring initializer URL https://start.spring.io and select following details.a. Select Maven project or Gradle project. For the example, I have selected maven project.
b. Select the language such as Java, Kotlin or Groovy. I have selected Java.
c. Now select the Spring Boot version. In our example, I have selected Spring Boot version as 2.0.4.
d. We need to specify artifact coordinates i.e. group and artifact name. In our example I have specified project metadata as following.
Group: com.concretepage
Artifact: spring-demo
e. Now select dependencies required by project. If we want to create web project then enter web keyword and we will get drop-down for web and then select it. This will provide all required JAR dependencies to develop web project.
f. Now click on Generate Project button. A project will get started to download.
Find the print screen.
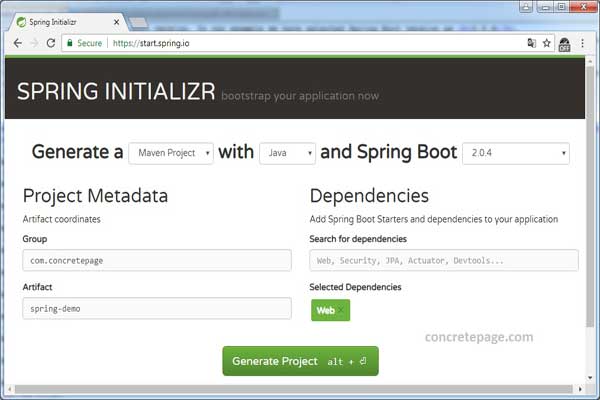
3. What are the Spring Boot Starters?
Ans: There are many Spring Boot starters for different purposes. Find some of them.spring-boot-starter-parent: It is a special starter that provides useful Maven defaults. It is used in parent section in the POM.
spring-boot-starter-web: Starter for building web and RESTful application with MVC.
spring-boot-starter-security: Starter for using Spring Security.
spring-boot-starter-web-services: Starter for using Spring web services.
spring-boot-starter-thymeleaf: Starter for Spring MVC using Thymeleaf views.
spring-boot-starter-freemarker: Starter for Spring MVC using FreeMarker views.
Find a sample
pom.xml
to start a Spring Boot web application.
pom.xml
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.concretepage</groupId> <artifactId>spring-boot-app</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>spring-boot-app</name> <description>Spring Boot Application</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.0.4.RELEASE</version> <relativePath/> </parent> <properties> <java.version>9</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
4. What is the role of @EnableAutoConfiguration annotation?
Ans:EnableAutoConfiguration
enables auto-configurations that we need in our applications. Auto-configurations are usually based on our classpath and beans we have defined. If tomcat-embedded.jar
is available in our classpath then in web application and embedded tomcat will be started on application startup. It is recommended that we should place @EnableAutoConfiguration
in a root package so that all sub-packages and classes can be searched. We create a Java main class annotated with EnableAutoConfiguration
as application starter.
@Configuration @EnableAutoConfiguration @ComponentScan public class ApplicationMain { public static void main(String[] args) { SpringApplication.run(ApplicationMain.class, args); } }
5. What is the role of @SpringBootApplication annotation?
Ans:@SpringBootApplication
annotation is the combination of @Configuration
, @EnableAutoConfiguration
and @ComponentScan
annotations. Our application starter main class is created by annotating @SpringBootApplication
.
@SpringBootApplication public class ApplicationMain { public static void main(String[] args) { SpringApplication.run(ApplicationMain.class, args); } }
6. What are frequently used commands to create and run Spring Boot Applications?
Ans: Here we are consolidating some commands used to create and run spring boot applications.1. With Maven
mvn dependency:tree: Prints tree of JAR dependencies.
mvn clean eclipse:eclipse: Creates
.classpath
mvn clean package: Creates JAR/WAR for the application.
mvn spring-boot:run: Starts application in exploded form.
2. With Gradle
gradle dependencies: Prints list of direct and transitive dependencies.
gradle clean eclipse: Creates
.classpath
gradle clean build: Creates JAR/WAR for the application.
gradle bootRun: Starts application in exploded form.
3. Using Java Command
a. Run Executable JAR using Java command.
java -jar <JAR-NAME>
java -Xdebug -Xrunjdwp:server=y,transport=dt_socket,address=8000,suspend=n \ -jar <JAR-NAME>
7. How to create executable JAR using Maven?
Ans: Find the steps to create executable JAR using Maven.Step 1: Make sure your
pom.xml
should contain.
a.
<packaging>jar</packaging>
<plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin>
Step 3: For the configuration
<artifactId>spring-boot-demo</artifactId> <version>0.0.1-SNAPSHOT</version>
spring-boot-demo-0.0.1-SNAPSHOT.jar : This is executable JAR
spring-boot-demo-0.0.1-SNAPSHOT.jar.original : This is original JAR and not executable.
Step 4: Run executable JAR as following.
java -jar target/spring-boot-demo-0.0.1-SNAPSHOT.jar
8. How to create executable JAR using Gradle?
Ans: spring-boot-gradle-plugin is available in Gradle. We need not to configure it separately. Like Maven, we can create executable JAR using Gradle. spring-boot-gradle-plugin provides the following command functionality.1. gradle clean build : Create executable and original JAR.
2. gradle bootRun : Starts the application in exploded form.
9. How to use Spring Boot Developer Tools?
Ans: Spring providesspring-boot-devtools
for developer tools. This is helpful in application development mode. One of the features of developer tool is automatic restart of the server for any code change. To configure developer tools using Maven, we need to add spring-boot-devtools
dependency in pom.xml
.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> <optional>true</optional> </dependency>
1. When we create a JAR or WAR as fully packaged application and run it then developer tools are automatically disabled.
2. When we run the application using java -jar or special classloader, then it is considered a "production application" and developer tools will be automatically disabled.
3. It is best practice to set <optional>true</optional> that will avoid developer tools to apply transitively on other module.
4. When developer tools has been configured, the project in exploded form are started using restart classloader and fully packaged application are started using base classloader by Spring Boot.
10. How to use LiveReload in Browser with Spring Boot Dev Tools?
Ans: In web project development mode, whenever we change any file, generally we need to restart the server and refresh the browser to get updated data. Spring Boot developer tools have automatized these two tasks. To refresh browser automatically we need to install LiveReload in our browser. Follow the below steps to get ready with LiveReload.1. Go to the LiveReload extension link and install it.
2. Make sure that
spring-boot-devtools
is there in pom.xml
.
2. Spring Boot developer tool will start a LiveReload server.
3. LiveReload can be enabled and disabled.
11. How to use CommandLineRunner?
Ans: To useCommandLineRunner
, we create a class and implement it and override its run()
method and annotate this class with Spring stereotype such as @Component
. When Spring Boot application starts, just before finishing startup, CommandLineRunner
is executed. We can pass command line arguments to CommandLineRunner
. It is used to start any scheduler or log any message before application starts. Find the sample code to implement CommandLineRunner
.
@Component public class MyAppCommandLineRunner implements CommandLineRunner { private static final Logger logger = LoggerFactory.getLogger(MyAppCommandLineRunner.class); public void run(String... args) { String data = Arrays.stream(args).collect(Collectors.joining("|")); logger.info("Arguments:" + data); } }
12. How to use custom banner in Spring Boot application?
Ans: We use custom banner as following.Text Banner: For text banner, just create a file named as
banner.txt
with desired text and keep it at the location src\main\resources
.
Image Banner: For image banner, just create a file named as
banner.gif
and keep it at the location src\main\resources
. Other file extensions such as jpg, png can also be used. Console should support to display image.
In the
application.properties
we can configure following banner properties.
banner.charset: Configures banner encoding. Default is UTF-8
banner.location: Banner file location. Default is classpath:banner.txt.
banner.image.location: Configures banner image file location. Default is classpath:banner.gif. File can also be jpg, png.
banner.image.width: Configures width of the banner image in
char
. Default is 76.
banner.image.height: Configures height of the banner image in
char
. Default is based on image height.
banner.image.margin: Left hand image margin in
char
. Default is 2.
banner.image.invert: Configures if images should be inverted for dark terminal themes. Default is false.
13. How to load XML configuration in Spring Boot?
Ans: To load XML configuration, we use@ImportResource
with @SpringBootApplication
annotation.
@SpringBootApplication @ImportResource("classpath:app-conf.xml") public class ApplicationMain { public static void main(String[] args) { SpringApplication.run(ApplicationMain.class, args); } }
14. How to change default server port?
Ans: Default server port in Spring Boot application can be changed as following.1. With
application.properties
:
server.port = 8585
application.yml
:
server: port: 8585
--server.port
java -jar my-app.jar --server.port=8585
SERVER.PORT
key.
@SpringBootApplication public class ApplicationMain { public static void main(String[] args) { SpringApplication application = new SpringApplication(ApplicationMain.class); Map<String, Object> map = new HashMap<>(); map.put("SERVER.PORT", "8585"); application.setDefaultProperties(map); application.run(args); } }
15. How to change context path in Spring Boot application?
Ans: We can change context path by configuring propertyserver.servlet.context-path
in property file and using command line.
Using property file.
server.servlet.context-path = /spring-boot-app server.port = 8585
java -jar my-app.jar --server.servlet.context-path=/spring-boot-app --server.port=8585
@SpringBootApplication public class ApplicationMain { public static void main(String[] args) { SpringApplication application = new SpringApplication(ApplicationMain.class); Map<String, Object> map = new HashMap<>(); map.put("server.servlet.context-path", "/spring-app"); map.put("server.port", "8585"); application.setDefaultProperties(map); application.run(args); } }
16. How to configure Spring Boot properties?
Ans: Spring Boot loadsapplication.properties
and application.yml
from classpath by default. If both files are present in the classpath then both are loaded and merged into environment. Using these files, we configure our properties.
a. Using application.properties
server.servlet.context-path = /spring-boot-app server.port = 8585
server: servlet: context-path: /spring-boot-app port: 8585
17. How to configure HikariCP in Spring Boot Application?
Ans: When usingspring-boot-starter-jdbc
or spring-boot-starter-data-jpa
, HikariCP are resolved by default.
To configure Hikari specific connection pool settings, Spring Boot provides spring.datasource.hikari.*
prefix. Find the sample configurations.
application.properties
spring.datasource.hikari.connection-timeout=10000 spring.datasource.hikari.minimum-idle=5 spring.datasource.hikari.maximum-pool-size=15 spring.datasource.hikari.idle-timeout=400000 spring.datasource.hikari.max-lifetime=1500000 spring.datasource.hikari.auto-commit=true
18. How to configure Tomcat Connection Pool?
Ans: To use tomcat connection Pool, we need to resolvetomcat-jdbc
dependency.
<dependency> <groupId>org.apache.tomcat</groupId> <artifactId>tomcat-jdbc</artifactId> </dependency>
spring.datasource.tomcat.*
prefix. Find the sample configurations.
spring.datasource.type = org.apache.tomcat.jdbc.pool.DataSource spring.datasource.tomcat.initial-size=10 spring.datasource.tomcat.max-wait=30000 spring.datasource.tomcat.max-active=100 spring.datasource.tomcat.max-idle=20 spring.datasource.tomcat.min-idle=10 spring.datasource.tomcat.default-auto-commit=true spring.datasource.tomcat.test-on-borrow=false
19. How to configure logging in Spring Boot application?
Ans: Spring Boot uses Logback, Log4J2 and java util logging. By default Spring Boot uses Logback for its logging. By default log is logged in console. Starter for Logback is spring-boot-starter-logging. When we use any starter then spring-boot-starter-logging is resolved by default. We need not to include it separately. If Logback JAR is available in classpath then Spring Boot will always choose Logback for logging. To use other logging such as Log4J2, we need to exclude Logback JAR and add Log4J2 JAR in classpath. By defaultERROR
, WARN
and INFO
log level messages are logged in console. To change log level, use logging.level property in property file.
To get logs in file, we can configure logging.file or logging.path in property file. Log files will rotate when they reach 10 MB. Default logging configurations can be changed using following properties.
logging.level.* : Used as prefix with package name to set log level.
logging.file: Configures a log file name to log message in file.
logging.path: Only configures path for log file. Spring Boot creates a log file with name
spring.log
.
logging.pattern.console: Defines logging pattern in console.
logging.pattern.file: Defines logging pattern in file.
logging.pattern.level: Defines the format to render log level. Default is
%5p
.
logging.exception-conversion-word: Defines conversion word when logging exceptions.
20. How to configure logging using application.properties?
Ans: Find the sample logging configuration usingapplication.properties
.
logging.level.org.springframework.security= DEBUG logging.level.org.hibernate= DEBUG logging.path = concretepage/logs logging.pattern.file= %d{yyyy-MMM-dd HH:mm:ss.SSS} %-5level [%thread] %logger{15} - %msg%n logging.pattern.console= %d{yyyy-MMM-dd HH:mm:ss.SSS} %-5level [%thread] %logger{15} - %msg%n
21. How to configure logging using application.yml?
Ans: Find the sample logging configuration usingapplication.yml
.
logging: level: org: springframework: security: DEBUG hibernate: DEBUG path: concretepage/logs pattern: file: '%d{yyyy-MMM-dd HH:mm:ss.SSS} %-5level [%thread] %logger{15} - %msg%n' console: '%d{yyyy-MMM-dd HH:mm:ss.SSS} %-5level [%thread] %logger{15} - %msg%n'
22. How to use profiles in Spring Boot application?
Ans: Spring provides@Profile
annotation that is used with @Configuration
, @Component
, @Service
etc. Different profile is created for different environment such production, development and testing environments.
In development environment we can enable development profile and in production environment we can enable production profile and so on. A profile is activated using .properties/.yml, command line and programmatically. Suppose we have a development profile as
dev
then we will create property file as application-dev.properties
and it can be activated in following ways.
1. Using
application.properties
file.
spring.profiles.active=dev
java -jar -Dspring.profiles.active=dev springapp.jar
@SpringBootApplication public class ApplicationMain { public static void main(String[] args) { SpringApplication application = new SpringApplication(ApplicationMain.class); application.setAdditionalProfiles("dev"); application.run(args); } }
23. How to use Thymeleaf in Spring Boot application?
Ans: To use Thymeleaf with Spring Boot application, we needspring-boot-starter-thymeleaf
starter in Maven or Gradle file. If Spring Boot scans Thymeleaf library in classpath, it will automatically configures Thymeleaf.
We can change the default Thymeleaf configurations in
application.properties
. Find some of them.
spring.thymeleaf.mode: Template mode that will be applied on templates. Default is
HTML 5
.
spring.thymeleaf.prefix: Value that will prepend with view name to build the URL. Default value is
classpath:/templates/
.
spring.thymeleaf.suffix: Value that will append with view name to build the URL. Default value is
.html
.
24. How to configure datasource using application.properties?
Ans: To connect with a database in our Spring Boot application, we need to includespring-boot-starter-jdbc
or spring-boot-starter-data-jpa
in our Maven or Gradle file. To configure datasource properties use prefix spring.datasource.*
in application.properties
.
application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/cp spring.datasource.username=root spring.datasource.password=cp
25. How to configure JPA properties using application.properties?
Ans: We configure JPA properties using prefixspring.jpa.properties.*
in application.properties
file. Find the sample JPA configurations with Hibernate.
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQLDialect spring.jpa.properties.hibernate.id.new_generator_mappings=true spring.jpa.properties.hibernate.format_sql=true
26. What is the Spring Boot starter to work with Database?
Ans: Usespring-boot-starter-data-jpa
to work with database.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency>
spring-boot-starter-jdbc
starter.
27. How to print datasource used by our Spring Boot application?
Ans: UseCommandLineRunner
as below.
@SpringBootApplication public class ApplicationMain implements CommandLineRunner { @Autowired DataSource dataSource; public static void main(String[] args) throws Exception { SpringApplication.run(ApplicationMain.class, args); } @Override public void run(String... args) throws Exception { System.out.println("DataSource = " + dataSource); } }
28. How to configure Spring Security in our Spring Boot web application?
Ans: To work with Spring Security we need to includespring-boot-starter-security
in our Maven or Gradle file. If Spring security is in the classpath then our web applications are automatically secured by default using basic authentication. A default username as 'user' and random password, that will be displayed in console when server starts, can be used for login authentication. The password is printed in console as follows.
Using default security password: 7e9850aa-d985-471a-bae1-25d741d4da23
1. To enable Spring Security in Spring Boot application just use the following Spring Boot starter.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-security</artifactId> </dependency>
security.user.password
property that needs to be configured in application.properties
as given below.
security.user.password=mypwd
security.*
prefix in application.properties
as given below.
security.basic.enabled: Enables basic authentication. Default value is true.
security.basic.path: Configures paths to apply security. We need to provide comma separated paths.
security.enable-csrf: Enables CSRF. Default value is false.
security.require-ssl: Enables and disables SSL. Default value is false.
security.sessions: Default value is stateless. Values can be always, never, if_required, stateless.
security.user.name: Configures user name. Default user is user.
security.user.password: Configures password.
security.user.role: Configures role. Default role is USER.
3. To fine-tuned logging configuration, we need to configure following property in
application.properties
with INFO
level.
logging.level.org.springframework.boot.autoconfigure.security= INFO
/css/**
, /js/**
, /images/**
and **/favicon.ico
.
5. The features such as HSTS, XSS, CSRF, caching are provided by default in Spring Security.
29. How to configure Jersey in Spring Boot application?
Ans: To configure Jersey in Spring Boot application, usespring-boot-starter-jersey
starter.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-jersey</artifactId> </dependency>
We can configure following Jersey properties in
application.properties
to change default configurations.
spring.jersey.application-path: Application path that acts as base URI.
spring.jersey.type: The value can be servlet or filter. Default value is servlet.
spring.jersey.filter.order: Defines the Jersey filter chain order. Default value is 0.
spring.jersey.init.*: Init parameters that will be passed to Jersey servlet or filter.
spring.jersey.servlet.load-on-startup: Load on startup priority for Jersey servlet. Default is -1.
30. How many ways can we run Spring Boot application?
Ans: We can run our Spring Boot application in following ways.1. Using Maven Command: Go to the root folder of the project using command prompt and run the command.
mvn spring-boot:run
2. Using Eclipse: Using command prompt, go to the root folder of the project and run.
mvn clean eclipse:eclipse
3. Using Executable JAR: Using command prompt, go to the root folder of the project and run the command.
mvn clean package
java -jar target/myapp.jar
31. How to work with Spring Boot REST application?
Ans: To work with Spring Boot RESTful web service, we need to providespring-boot-starter-web
Maven dependency as following.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency>
jackson-databind
by default. Spring Boot REST gives JSON response by default because it detects jackson-databind
in its classpath.
To support XML response in Spring Boot REST, we need to provide
jackson-dataformat-xml
library with spring-boot-starter-web
. Find the Maven dependency.
<dependency> <groupId>com.fasterxml.jackson.dataformat</groupId> <artifactId>jackson-dataformat-xml</artifactId> </dependency>
@RestController
in controller classes.
32. What is Spring Boot Starter for SOAP web service?
Ans: To create SOAP web service, we needspring-boot-starter-web-services
as following.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web-services</artifactId> </dependency> <dependency> <groupId>wsdl4j</groupId> <artifactId>wsdl4j</artifactId> </dependency>
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter</artifactId> </dependency> <dependency> <groupId>org.springframework.ws</groupId> <artifactId>spring-ws-core</artifactId> </dependency>
33. How to get JdbcTemplate in Spring Boot application?
Ans: Find the steps to getJdbcTemplate
in Spring Boot application.
1. Resolve
spring-boot-starter-jdbc
dependency. This will auto-configure JdbcTemplate
.
2. Configure datasource in application.properties
.
spring.datasource.driver-class-name=com.mysql.jdbc.Driver spring.datasource.url=jdbc:mysql://localhost:3306/cpdb spring.datasource.username=root spring.datasource.password=cp
JdbcTemplate
.
@Repository public class CustomerDAO { @Autowired private JdbcTemplate jdbcTemplate; ----------------- }
34. How to use @EnableJpaRepositories in Spring Boot application?
Ans: Use@EnableJpaRepositories
annotation with @SpringBootApplication
. Using @EnableJpaRepositories
we will configure package name in which our repository classes reside. Suppose the package of our repository classes is com.cp.repository
, we will use @EnableJpaRepositories
as following.
@SpringBootApplication @EnableJpaRepositories("com.cp.repository") public class ApplicationMain { ------ }
basePackageClasses
attribute of the @EnableJpaRepositories
annotation.
35. How to get EntityManager in Spring Boot application?
Ans: Find the steps to getEntityManager
in Spring Boot application.
1. Resolve
spring-boot-starter-data-jpa
dependency.
2. Configure datasource in
application.properties
.
3. Use
@PersistenceContext
to get EntityManager
in DAO.
@Repository public class ArticleDAO { @PersistenceContext private EntityManager entityManager; ------ }
36. How to create Spring Boot MVC application?
Ans: Resolvespring-boot-starter-web
dependency.
When Spring Boot scans Spring Web in classpath, it atomically configures Spring Web MVC. To change any configuration, Spring Boot provides properties to be configured in
application.properties
. Find some properties.
spring.mvc.async.request-timeout: Timeout in milliseconds for asynchronous request.
spring.mvc.date-format: Date format to use.
spring.mvc.favicon.enabled: Enables and disables favicon. Default is true.
spring.mvc.locale: Define Locale.
spring.mvc.media-types.*: Maps file extensions to media type for content negotiation.
spring.mvc.servlet.load-on-startup: Configures startup priority for Spring Web Services Servlet. Default value is -1.
spring.mvc.static-path-pattern: Configures path pattern for static resources.
spring.mvc.view.prefix: Configures prefix for Spring view such as JSP.
spring.mvc.view.suffix: Configures view suffix.
To take a complete control on Spring MVC configuration, we can create a configuration class annotated with
@Configuration
and @EnableWebMvc
. To override any settings we need to extend WebMvcConfigurerAdapter
class. To create a view in Spring Boot MVC, we should prefer template engine and not JSP because for the JSP there are known limitations with embedded servlet container.
37. How to register Servlet in Spring Boot application?
Ans: We can register Servlet in many ways. Suppose we haveHelloServlet
.
1. Using
ServletRegistrationBean
: Create a ServletRegistrationBean
bean in JavaConfig and register Servlet. It works since Servlet 3.0.
WebConfig.java
@Configuration public class WebConfig { @Bean public ServletRegistrationBean<HttpServlet> countryServlet() { ServletRegistrationBean<HttpServlet> registration = new ServletRegistrationBean<>(); registration.setServlet(new HelloServlet()); registration.addUrlMappings("/country/*"); registration.setLoadOnStartup(1); return registration; } }
@ServletComponentScan
: It will scan Servlets annotated with @WebServlet
annotation. It is used with @Configuration
or @SpringBootApplication
annotations.
SpringBootAppStarter.java
@ServletComponentScan @SpringBootApplication public class ApplicationMain { public static void main(String[] args) { SpringApplication.run(ApplicationMain.class, args); } }
38. How to register Filter in Spring Boot application?
Ans: A Filter can be registered in Spring Boot in many ways. Suppose we have aHelloFilter
.
1. Using
FilterRegistrationBean
: Create a FilterRegistrationBean
bean in JavaConfig and register our Filter. It works since Servlet 3.0.
@Bean public FilterRegistrationBean<HelloFilter> abcFilter() { FilterRegistrationBean<HelloFilter> registration = new FilterRegistrationBean<>(); registration.setFilter(new HelloFilter()); registration.addUrlPatterns("/app/*"); registration.setOrder(Ordered.LOWEST_PRECEDENCE -1); return registration; }
@Component
and @Order
: We can register a filter using @Component
and set order using @Order
.
HelloFilter.java
@Order(Ordered.LOWEST_PRECEDENCE -1) @Component public class HelloFilter implements Filter { ------ }
@ServletComponentScan
: To register a filter in Spring Boot, we can use @ServletComponentScan
and the filter should be annotated with @WebFilter
annotation. We need to use @ServletComponentScan
with @Configuration
or @SpringBootApplication
annotations. @ServletComponentScan
in Spring Boot will scan servlets annotated with @WebServlet
, filters annotated with @WebFilter
and listeners annotated with @WebListener
only when using an embedded web server.
39. How to register Listener in Spring Boot application?
Ans: A Listener in Spring Boot application can be registered in many ways. Suppose we have aSessionCountListener
.
1. Using
ServletListenerRegistrationBean
: Create a ServletListenerRegistrationBean
bean in JavaConfig and register Listener.
@Bean public ServletListenerRegistrationBean<SessionCountListener> sessionCountListener() { ServletListenerRegistrationBean<SessionCountListener> registration = new ServletListenerRegistrationBean<>(); registration.setListener(new SessionCountListener()); return registration; }
@Component
.
@Component public class SessionCountListener implements HttpSessionListener { ------ }
@ServletComponentScan
with @Configuration
or @SpringBootApplication
annotations. The servlet listeners annotated with @WebListener
will be scanned by @ServletComponentScan
.
40. How to use Redis Cache in Spring Boot application?
Ans: Find the steps to use Redis cache in Spring Boot application.1. To get Redis connections, we use Lettuce or Jedis client libraries. Spring Boot starter
spring-boot-starter-data-redis
resolves Lettuce by default. To get pooled connection factory, we need to provide commons-pool2
on the classpath.
2. Redis properties are configured using
spring.redis.*
prefix in application.properties
file.
spring.redis.host=localhost spring.redis.port=6379 spring.redis.password= spring.redis.lettuce.pool.max-active=8 spring.redis.lettuce.pool.max-idle=6 spring.redis.lettuce.pool.min-idle=3 spring.redis.lettuce.pool.max-wait=-1ms spring.redis.lettuce.shutdown-timeout=200ms spring.cache.redis.cache-null-values=false spring.cache.redis.time-to-live=700000 spring.cache.redis.use-key-prefix=true
@EnableCaching
annotation with @Configuration
or @SpringBootApplication
annotations.
@SpringBootApplication @EnableCaching public class ApplicationMain { public static void main(String[] args) { SpringApplication.run(ApplicationMain.class, args); } }
41. How to use Jedis client with Spring Redis?
Ans: By default Spring Boot 2.0 starterspring-boot-starter-data-redis
uses Lettuce. To use Jedis we need to exclude Lettuce dependency and include Jedis. Find the Maven dependencies to use Jedis.
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> <exclusions> <exclusion> <groupId>io.lettuce</groupId> <artifactId>lettuce-core</artifactId> </exclusion> </exclusions> </dependency> <dependency> <groupId>redis.clients</groupId> <artifactId>jedis</artifactId> </dependency>
jedis
dependency will automatically resolve commons-pool2
on the classpath.
To configure Jedis pool we need to use
spring.redis.*
prefix with Jedis pool connection properties. Find the Jedis pool sample configurations.
application.properties
spring.redis.jedis.pool.max-active=7 spring.redis.jedis.pool.max-idle=7 spring.redis.jedis.pool.min-idle=2 spring.redis.jedis.pool.max-wait=-1ms