@ExtendWith + SpringExtension in Spring 5 Test
January 11, 2019
SpringExtension
introduced in Spring 5, is used to integrate Spring TestContext with JUnit 5 Jupiter Test. SpringExtension
is used with JUnit 5 Jupiter @ExtendWith
annotation as following.
1. With JavaConfig.
@ExtendWith(SpringExtension.class) @ContextConfiguration(classes = AppConfig.class) public class MyAppTest { ------ }
@ExtendWith(SpringExtension.class) @ContextConfiguration("/spring-config.xml") public class MyAppTest { ------ }
SpringExtension
class with JUnit @ExtendWith
annotation.
Contents
Technologies Used
Find the technologies being used in our example.1. Java 11
2. Spring 5.1.3.RELEASE
3. Spring Boot 2.1.1.RELEASE
4. JUnit 5
5. Maven 3.5.2
6. Eclipse 2018-09
@ExtendWith(SpringExtension.class) Example
MyAppTest.javapackage com.concretepage; import static org.junit.Assert.assertTrue; import static org.junit.jupiter.api.Assertions.assertEquals; import org.junit.jupiter.api.AfterAll; import org.junit.jupiter.api.AfterEach; import org.junit.jupiter.api.BeforeAll; import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test; import org.junit.jupiter.api.TestInfo; import org.junit.jupiter.api.extension.ExtendWith; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.test.context.ContextConfiguration; import org.springframework.test.context.junit.jupiter.SpringExtension; import com.concretepage.config.AppConfig; import com.concretepage.service.MyService; @ExtendWith(SpringExtension.class) @ContextConfiguration(classes = AppConfig.class) public class MyAppTest { @Autowired private MyService myService; @BeforeAll static void initAll() { System.out.println("---Inside initAll---"); } @BeforeEach void init(TestInfo testInfo) { System.out.println("Start..." + testInfo.getDisplayName()); } @Test public void messageTest() { String msg = myService.getMessage(); assertEquals("Hello World!", msg); } @Test public void multiplyNumTest() { int val = myService.multiplyNum(5, 10); assertEquals(50, val); } @Test public void idAvailabilityTest() { boolean val = myService.isIdAvailable(100); assertTrue(val); } @AfterEach void tearDown(TestInfo testInfo) { System.out.println("Finished..." + testInfo.getDisplayName()); } @AfterAll static void tearDownAll() { System.out.println("---Inside tearDownAll---"); } }
@ContextConfiguration
loads an ApplicationContext
for Spring integration test. @ContextConfiguration
can load ApplicationContext
using XML resource or the JavaConfig annotated with @Configuration
.
Now find main Java files.
AppConfig.java
package com.concretepage.config; import org.springframework.context.annotation.ComponentScan; import org.springframework.context.annotation.Configuration; @Configuration @ComponentScan("com.concretepage") public class AppConfig { }
package com.concretepage.service; import org.springframework.stereotype.Service; @Service public class MyService { public String getMessage() { return "Hello World!"; } public int multiplyNum(int num1, int num2) { return num1 * num2; } public boolean isIdAvailable(long id) { if (id == 100) { return true; } return false; } }
package com.concretepage; import org.springframework.context.annotation.AnnotationConfigApplicationContext; import com.concretepage.service.MyService; public class SpringProfileDemo { public static void main(String[] args) { AnnotationConfigApplicationContext ctx = new AnnotationConfigApplicationContext(); ctx.scan("com.concretepage"); ctx.refresh(); MyService myService = ctx.getBean(MyService.class); System.out.println(myService.getMessage()); } }
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.concretepage</groupId> <artifactId>spring-boot-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>spring-demo</name> <description>Spring Demo Project</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>2.1.1.RELEASE</version> </parent> <properties> <context.path>spring-app</context.path> <java.version>11</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-api</artifactId> <version>5.3.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-engine</artifactId> <version>5.3.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter-params</artifactId> <version>5.3.2</version> <scope>test</scope> </dependency> <dependency> <groupId>org.junit.platform</groupId> <artifactId>junit-platform-launcher</artifactId> <version>1.3.2</version> <scope>test</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> <finalName>${context.path}</finalName> </build> </project>
MyAppTest
class in Eclipse and we will get following output.
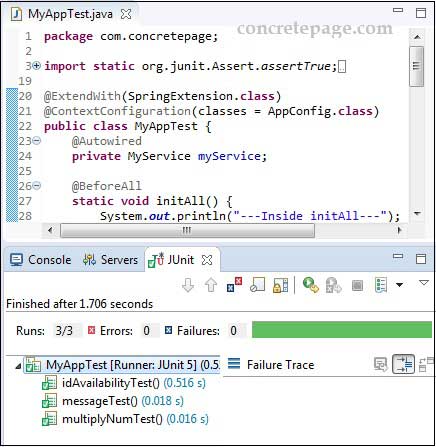
References
Spring Doc: SpringExtensionJUnit 5 @BeforeAll and @AfterAll Example