PrimeFaces 5 DataScroller Example
January 30, 2015
In this page we will learn PrimeFaces 5 DataScroller. DataScroller has been introduced in PrimeFaces 5. Using DataScroller, we can display data more while scrolling bar.
To use dataScroller tag we need to import below URL of PrimeFaces.
xmlns:p=http://primefaces.org/ui
Here p will be used to refer the dataScroller tag in UI as below
<p:dataScroller value="#{dataScrollerBean.students}" var="student" chunkSize="10">
value: The property of managed bean is referred.
var: While iterating each object is assigned to this variable.
chunkSize : The iteration is done chunk wise. On scrolling next iteration is done for the chunk size.
Software Required to Run Example
Find the software to run demo project for primeFaces 5 dataScroller Example.1. Java 6
2. Tomcat 7
3. Eclipse
4. Gradle
Project Structure in Eclipse
Find the project structure in eclipse.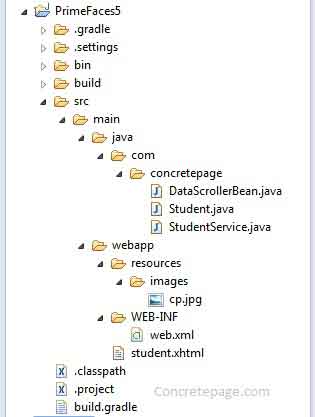
Gradle File to Resolve JAR of JSF 2 and PrimeFaces 5
Find the gradle file to resolve JSF 2 and PrimeFaces 5 jar.build.gradle
apply plugin: 'java' apply plugin: 'eclipse' apply plugin: 'war' archivesBaseName = 'primefaces5' version = '1' repositories { mavenCentral() } dependencies { compile 'com.sun.faces:jsf-api:2.2.9' compile 'com.sun.faces:jsf-impl:2.2.9' compile 'org.primefaces:primefaces:5.1' compile 'javax.enterprise:cdi-api:1.2' }
Create UI using PrimeFaces dataScroller, graphicImage and outputPanel
dataScroller is a tag introduced in PrimeFaces 5 which displays data chunk wise on scrolling bar. In the example we have 100 students and chunk size is 10. A loop of chunk size runs and loads data more to display the records. The object of each record is assigned to var attribute of dataScroller tag.contentflow.xhtml
<html lang="en" xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core" xmlns:p="http://primefaces.org/ui"> <h:head> <title>PrimeFaces 5 DataScroller Example</title> </h:head> <h:body> <h3>PrimeFaces 5 DataScroller Example</h3> <h:form> <p:dataScroller value="#{dataScrollerBean.students}" var="student" chunkSize="10"> <f:facet name="header"> Scroll Down to Load More Students </f:facet> <h:panelGrid columns="2" style="width:100%"> <p:graphicImage name="images/cp.jpg" /> <p:outputPanel> <h:panelGrid columns="2" cellpadding="5"> <h:outputText value="Id:" /> <h:outputText value="#{student.id}" /> <h:outputText value="Name:" /> <h:outputText value="#{student.name}"/> <h:outputText value="Location:" /> <h:outputText value="#{student.location}"/> </h:panelGrid> </p:outputPanel> </h:panelGrid> </p:dataScroller> </h:form> </h:body> </html>
Create JSF Managed Bean
Find the managed bean to be used for UI.DataScrollerBean.java
package com.concretepage; import java.io.Serializable; import java.util.List; import javax.annotation.PostConstruct; import javax.faces.bean.ManagedBean; import javax.faces.bean.ManagedProperty; import javax.faces.bean.ViewScoped; @ManagedBean(name = "dataScrollerBean") @ViewScoped public class DataScrollerBean implements Serializable { private static final long serialVersionUID = 1L; private List<Student> students; @ManagedProperty("#{studentService}") private StudentService studentService; @PostConstruct public void init() { students = studentService.getStudents(); } public List<Student> getStudents() { return students; } public void setStudentService(StudentService studentService) { this.studentService = studentService; } }
StudentService.java
package com.concretepage; import java.io.Serializable; import java.util.ArrayList; import java.util.List; import javax.faces.bean.ApplicationScoped; import javax.faces.bean.ManagedBean; @ManagedBean(name = "studentService") @ApplicationScoped public class StudentService implements Serializable { private static final long serialVersionUID = 1L; static List<Student> list = new ArrayList<Student>(); static{ for (int i= 1; i<=100; i++){ list.add(new Student(i, "Student-"+i, "Location-"+i)); } } public List<Student> getStudents(){ return list; } }
Student.java
package com.concretepage; public class Student { private Integer id; private String name; private String location; public Student(Integer id, String name, String location){ this.id = id; this.name = name; this.location = location; } public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getLocation() { return location; } public void setLocation(String location) { this.location = location; } }
web.xml
Find the web.xml being used in the example.web.xml
<?xml version="1.0" encoding="ISO-8859-1" ?> <web-app xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd" version="3.0"> <display-name>PrimeFaces 5 DataScroller Example </display-name> <servlet> <servlet-name>FacesServlet</servlet-name> <servlet-class>javax.faces.webapp.FacesServlet</servlet-class> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>FacesServlet</servlet-name> <url-pattern>/faces/*</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>FacesServlet</servlet-name> <url-pattern>*.xhtml</url-pattern> </servlet-mapping> <servlet-mapping> <servlet-name>FacesServlet</servlet-name> <url-pattern>*.jpg</url-pattern> </servlet-mapping> </web-app>
Output
Deploy the WAR file in Tomcat and access the URL as http://localhost:8080/primefaces5-1/student.xhtmlWe will see the UI as given below.
