Getting Started with Thymeleaf
April 13, 2023
On this page, we will learn creating Java Thymeleaf application.
1. Thymeleaf is a template engine that can process XML, XHTML, HTML5 files. Thymeleaf processes templates fast with least amount of IO operations.
2. Thymeleaf gives speed to project development in such a way that front end and backend team can work together on UI pages.
3. Thymeleaf provides internalization. The basic API provided by Thymeleaf are
ServletContextTemplateResolver
and TemplateEngine
. The ServletContextTemplateResolver
resolves templates from location and TemplateEngine
processes the templates using TemplateEngine.process()
method.
4. Thymeleaf expression can get values from property file and
WebContext
. We must care that property file and template must be of same name and located in same directory.
Technologies Used
1. Java 82. Thymeleaf 3.0.0.ALPHA03
3. Servlet 3.1
4. Tomcat 8
5. Gradle
6. Eclipse
Project Structure in Eclipse
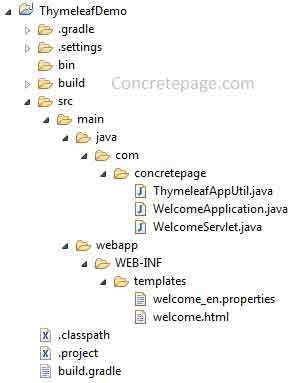
Gradle File
build.gradledependencies { compile 'javax.servlet:javax.servlet-api:3.1.0' compile 'org.thymeleaf:thymeleaf:3.0.0.ALPHA03' }
Using th:text and externalizing text
Find the HTML page used in our demo. If we directly access this HTML page, we will see offline values and when we run via server, we get values of tag body processed by Thymeleaf.WEB-INF\templates\welcome.html
<!DOCTYPE html SYSTEM "http://www.thymeleaf.org/dtd/xhtml1-strict-thymeleaf-4.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:th="http://www.thymeleaf.org"> <head> <title>Thymeleaf Demo</title> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> </head> <body> <p th:text="#{welcome.msg}">Welcome Offline</p> <p>Date : <span th:text="${currentDate}">Sat Oct 24 2015</span></p> </body> </html>
#{...}: Fetches the value from property file. The expression #{welcome.msg} gets the value of key welcome.msg from property file.
${...}: This is OGNL expression and fetches the value set from
WebContext
. The expression ${currentDate} gets the value for the attribute currentDate set in WebContext
.
Creating TemplateEngine
ThymeleafAppUtil.javapackage com.concretepage; import org.thymeleaf.TemplateEngine; import org.thymeleaf.templateresolver.ServletContextTemplateResolver; public class ThymeleafAppUtil { private static TemplateEngine templateEngine; static { ServletContextTemplateResolver templateResolver = new ServletContextTemplateResolver(); templateResolver.setTemplateMode("XHTML"); templateResolver.setPrefix("/WEB-INF/templates/"); templateResolver.setSuffix(".html"); templateResolver.setCacheTTLMs(3600000L); templateEngine = new TemplateEngine(); templateEngine.setTemplateResolver(templateResolver); } public static TemplateEngine getTemplateEngine() { return templateEngine; } }
TemplateEngine: It executes Thymeleaf templates.
Property file used in Demo
Property file and template must have same name and should be located in same directory.WEB-INF\templates\welcome_en.properties
welcome.msg= Welcome to Thymeleaf World!
Run Demo
WelcomeServlet.javapackage com.concretepage; import java.io.IOException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet(urlPatterns = "/welcome", loadOnStartup = 1) public class WelcomeServlet extends HttpServlet { private static final long serialVersionUID = 1L; public void doPost(HttpServletRequest request, HttpServletResponse response) throws IOException{ doGet(request,response); } public void doGet(HttpServletRequest request, HttpServletResponse response) throws IOException{ response.setContentType("text/html;charset=UTF-8"); response.setHeader("Pragma", "no-cache"); response.setHeader("Cache-Control", "no-cache"); response.setDateHeader("Expires", 0); WelcomeApplication application = new WelcomeApplication(); application.process(request, response); } }
Output
Find the URL to run the demo.http://localhost:8080/cp/welcome
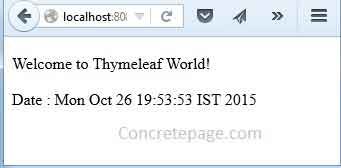